Regular Expression Matching: Mastering Dynamic Programming
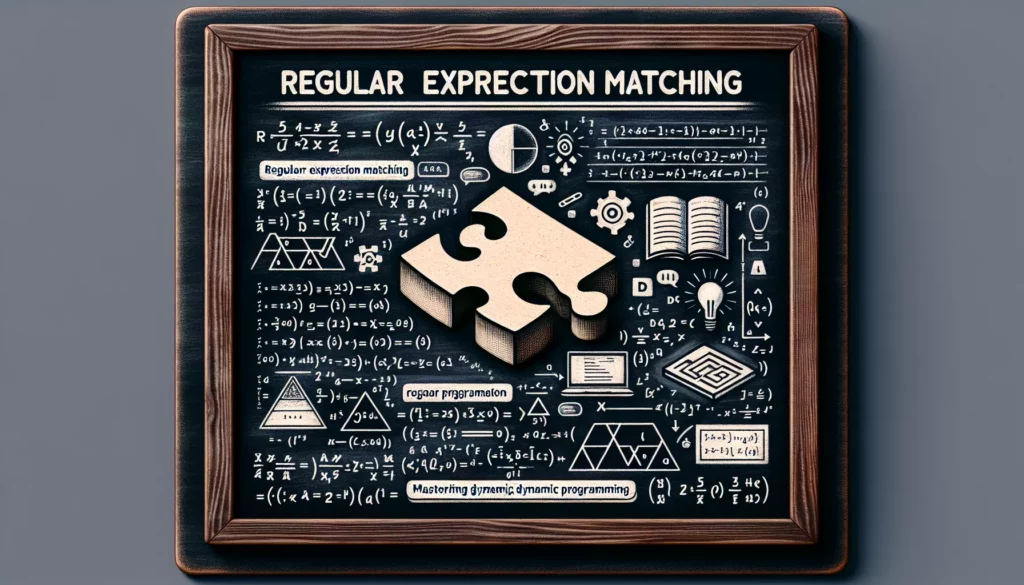
Welcome to AlgoCademy’s comprehensive guide on Regular Expression Matching using Dynamic Programming. This advanced topic is crucial for coding interviews, especially when targeting top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). In this tutorial, we’ll dive deep into the problem, explore its intricacies, and develop a robust solution using dynamic programming techniques.
Understanding the Problem
Before we delve into the solution, let’s clearly define the problem of Regular Expression Matching:
Given an input string
s
and a patternp
, implement regular expression matching with support for'.'
and'*'
where:
'.'
Matches any single character.'*'
Matches zero or more of the preceding element.The matching should cover the entire input string (not partial).
This problem is a classic example of where dynamic programming shines. It’s a favorite among interviewers because it tests a candidate’s ability to break down a complex problem, recognize patterns, and implement an efficient solution.
Why is this Problem Important?
Regular Expression Matching is not just an academic exercise; it has real-world applications in various domains:
- Text Processing: Used in search and replace operations in text editors.
- Data Validation: Verifying if input data matches a specific pattern (e.g., email addresses, phone numbers).
- Parsing: Extracting information from structured text data.
- Compiler Design: Used in lexical analysis phase of compilers.
Moreover, this problem tests several key programming skills:
- String manipulation
- Recursive thinking
- Dynamic programming
- Time and space complexity analysis
Approaching the Problem
Let’s break down our approach into steps:
- Understand the problem constraints
- Develop a recursive solution
- Identify overlapping subproblems
- Implement a dynamic programming solution
- Optimize for time and space complexity
1. Understanding the Problem Constraints
Before diving into the solution, it’s crucial to clarify the constraints and edge cases:
- The input string
s
contains only lowercase English letters. - The pattern
p
contains only lowercase English letters,'.'
, and'*'
. - It is guaranteed for each appearance of the character
'*'
, there will be a previous valid character to match. - The matching should cover the entire input string (not partial).
2. Developing a Recursive Solution
Let’s start by thinking about this problem recursively. We can compare characters from both strings one by one:
- If the current characters match or the pattern has a
'.'
, we move to the next characters in both strings. - If we encounter a
'*'
in the pattern, we have two choices:- Ignore the
'*'
and its preceding character (zero occurrence) - Use the
'*'
to match the current character in s (one or more occurrences)
- Ignore the
Here’s a basic recursive implementation:
class Solution:
def isMatch(self, s: str, p: str) -> bool:
if not p:
return not s
first_match = bool(s) and p[0] in {s[0], '.'}
if len(p) >= 2 and p[1] == '*':
return (self.isMatch(s, p[2:]) or
first_match and self.isMatch(s[1:], p))
else:
return first_match and self.isMatch(s[1:], p[1:])
This recursive solution works, but it’s inefficient for large inputs due to redundant computations.
3. Identifying Overlapping Subproblems
The key to applying dynamic programming is recognizing overlapping subproblems. In this case, we often end up solving the same subproblems multiple times. For example, when matching "aaaab"
against "a*b"
, we repeatedly check if "aaab"
, "aab"
, "ab"
match "a*b"
.
4. Implementing a Dynamic Programming Solution
To optimize our solution, we can use a 2D DP table where dp[i][j]
represents whether the first i
characters of s
match the first j
characters of p
.
Here’s the dynamic programming implementation:
class Solution:
def isMatch(self, s: str, p: str) -> bool:
m, n = len(s), len(p)
dp = [[False] * (n + 1) for _ in range(m + 1)]
# Empty pattern matches empty string
dp[0][0] = True
# Handle patterns like a*, a*b*, etc.
for j in range(1, n + 1):
if p[j-1] == '*':
dp[0][j] = dp[0][j-2]
for i in range(1, m + 1):
for j in range(1, n + 1):
if p[j-1] == '*':
dp[i][j] = dp[i][j-2]
if p[j-2] == '.' or p[j-2] == s[i-1]:
dp[i][j] |= dp[i-1][j]
elif p[j-1] == '.' or p[j-1] == s[i-1]:
dp[i][j] = dp[i-1][j-1]
return dp[m][n]
5. Optimizing for Time and Space Complexity
Let’s analyze the time and space complexity of our dynamic programming solution:
- Time Complexity: O(mn), where m is the length of string s and n is the length of pattern p. We fill a 2D table of size (m+1) x (n+1).
- Space Complexity: O(mn) to store the DP table.
While this is a significant improvement over the recursive solution, we can optimize the space complexity further. Notice that each cell in our DP table only depends on the current row and the previous row. We can reduce our space complexity to O(n) by using only two rows:
class Solution:
def isMatch(self, s: str, p: str) -> bool:
m, n = len(s), len(p)
dp = [False] * (n + 1)
dp[0] = True
for j in range(1, n + 1):
if p[j-1] == '*':
dp[j] = dp[j-2]
for i in range(1, m + 1):
prev = dp[0]
dp[0] = False
for j in range(1, n + 1):
temp = dp[j]
if p[j-1] == '*':
dp[j] = dp[j-2]
if p[j-2] == '.' or p[j-2] == s[i-1]:
dp[j] |= prev
elif p[j-1] == '.' or p[j-1] == s[i-1]:
dp[j] = prev
else:
dp[j] = False
prev = temp
return dp[n]
This optimized version maintains the O(mn) time complexity while reducing the space complexity to O(n).
Common Pitfalls and Edge Cases
When implementing this solution, be aware of these common pitfalls and edge cases:
- Empty Strings: Ensure your solution handles empty input strings and patterns correctly.
- Consecutive Stars: Patterns like
"a**"
are valid and should be handled correctly. - Dot and Star Combination: The pattern
".*"
is particularly tricky as it can match any sequence of characters. - Greedy vs Non-Greedy Matching: This implementation uses greedy matching. Be prepared to discuss the differences if asked.
Testing Your Solution
To ensure your implementation is correct, test it with various input combinations. Here are some test cases to get you started:
def test_regex_matching():
solution = Solution()
assert solution.isMatch("aa", "a") == False
assert solution.isMatch("aa", "a*") == True
assert solution.isMatch("ab", ".*") == True
assert solution.isMatch("aab", "c*a*b") == True
assert solution.isMatch("mississippi", "mis*is*p*.") == False
assert solution.isMatch("", "") == True
assert solution.isMatch("a", "") == False
assert solution.isMatch("", "a*") == True
print("All test cases passed!")
Performance Analysis
Let’s compare the performance of our recursive and dynamic programming solutions:
Approach | Time Complexity | Space Complexity |
---|---|---|
Recursive | O(2^(m+n)) | O(m+n) |
DP (2D array) | O(mn) | O(mn) |
DP (1D array) | O(mn) | O(n) |
The dynamic programming solution offers a significant improvement in time complexity, making it suitable for larger inputs. The space-optimized version further reduces memory usage, which can be crucial in memory-constrained environments.
Real-World Applications
Understanding and implementing regular expression matching is valuable beyond coding interviews. Here are some real-world applications:
- Data Validation: Verifying user inputs in web forms (e.g., email addresses, phone numbers).
- Log Analysis: Parsing and filtering log files to extract relevant information.
- Search Engines: Implementing advanced search functionalities with wildcard matching.
- Compiler Design: Lexical analysis phase of compilers often uses regex for tokenization.
- Data Scraping: Extracting specific patterns of data from web pages or documents.
Advanced Topics and Variations
Once you’ve mastered the basic regular expression matching problem, consider exploring these advanced topics and variations:
- Non-Greedy Matching: Implement a version that uses non-greedy (lazy) matching instead of greedy matching.
- Extended Regex Features: Add support for more regex features like character classes
[a-z]
, alternation|
, or capturing groups()
. - Finite Automata: Implement regex matching using finite automata theory.
- Regex Engine Optimization: Explore techniques to optimize regex engines for faster matching, such as using Thompson’s construction algorithm.
- Backtracking and Its Pitfalls: Study the backtracking behavior in regex engines and how to avoid catastrophic backtracking.
Conclusion
Congratulations! You’ve now mastered the Regular Expression Matching problem using dynamic programming. This problem showcases the power of DP in solving complex pattern matching tasks efficiently. As you’ve seen, the journey from a recursive solution to an optimized DP approach involves careful analysis and step-by-step optimization.
Remember, the key takeaways from this problem are:
- Recognizing overlapping subproblems in recursive solutions
- Constructing a DP table to store intermediate results
- Optimizing space complexity by reducing dimensions in the DP table
- Handling edge cases and special characters in pattern matching
As you continue your journey with AlgoCademy, apply these principles to other problems. Regular expression matching is just one example of how dynamic programming can transform exponential-time algorithms into efficient polynomial-time solutions.
Keep practicing, and don’t hesitate to revisit this problem as you grow in your understanding of algorithms and data structures. The skills you’ve developed here will serve you well in coding interviews and real-world programming challenges.
Happy coding, and may your algorithms always be optimal!