Meet in the Middle: A Powerful Algorithmic Technique for Efficient Problem Solving
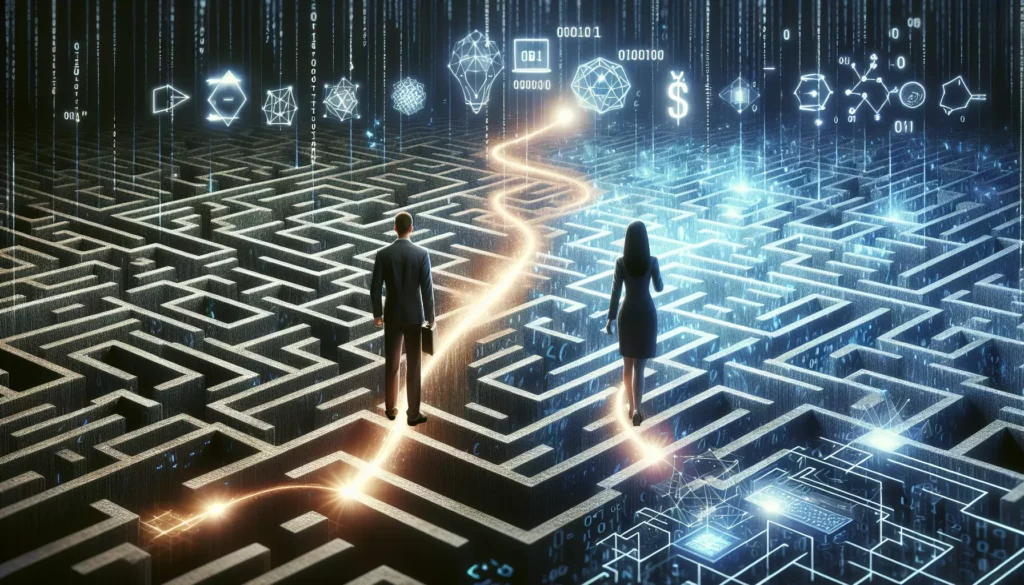
In the world of algorithmic problem-solving, efficiency is key. As programmers, we’re constantly seeking ways to optimize our code and reduce time complexity. One powerful technique that often comes to the rescue is the “Meet in the Middle” algorithm. This approach can significantly improve the performance of certain types of problems, especially those involving large search spaces or combinatorial challenges.
In this comprehensive guide, we’ll dive deep into the Meet in the Middle algorithm, exploring its concept, implementation, and applications. Whether you’re preparing for technical interviews at top tech companies or simply looking to enhance your problem-solving skills, understanding this technique can give you a significant edge.
Table of Contents
- What is Meet in the Middle?
- How Does Meet in the Middle Work?
- When to Use Meet in the Middle
- Implementing Meet in the Middle
- Example Problems and Solutions
- Optimization Tips and Tricks
- Comparison with Other Techniques
- Real-world Applications
- Interview Preparation Strategies
- Conclusion
1. What is Meet in the Middle?
Meet in the Middle is an algorithmic technique that splits a problem into two smaller subproblems, solves them independently, and then combines the results to find the overall solution. This approach is particularly useful when dealing with problems that have an exponential time complexity when solved directly.
The key idea behind Meet in the Middle is to reduce the search space by dividing it into two halves and exploring each half separately. By doing so, we can often achieve a significant reduction in time complexity, turning an otherwise intractable problem into a manageable one.
2. How Does Meet in the Middle Work?
The Meet in the Middle algorithm typically follows these steps:
- Divide the input data into two roughly equal parts.
- Generate all possible combinations or results for each half.
- Store the results from one half in a data structure (usually a hash table or a sorted array).
- Iterate through the results of the other half and check for complementary results in the stored data.
- Combine the complementary results to form the final solution.
By splitting the problem in half, we reduce the time complexity from O(2^n) to O(2^(n/2)), which can make a substantial difference for large inputs.
3. When to Use Meet in the Middle
Meet in the Middle is particularly effective in scenarios where:
- The problem has a large search space that grows exponentially.
- The input can be naturally divided into two parts.
- There’s a clear way to combine partial solutions from each half.
- The problem involves finding a specific sum, subset, or configuration that satisfies certain conditions.
Common problem types where Meet in the Middle shines include:
- Subset sum problems
- Knapsack problems
- Optimization problems with constraints
- Certain types of dynamic programming problems
4. Implementing Meet in the Middle
Let’s look at a basic implementation of the Meet in the Middle algorithm in Python. We’ll use a simple subset sum problem as an example: given a set of numbers, find if there exists a subset with a sum equal to a target value.
def meet_in_the_middle(arr, target):
n = len(arr)
left_half = arr[:n//2]
right_half = arr[n//2:]
def generate_subsets(arr):
n = len(arr)
subsets = []
for i in range(1 << n):
subset_sum = 0
for j in range(n):
if i & (1 << j):
subset_sum += arr[j]
subsets.append(subset_sum)
return subsets
left_sums = generate_subsets(left_half)
right_sums = generate_subsets(right_half)
left_sums.sort()
right_sums.sort()
for left_sum in left_sums:
complement = target - left_sum
if binary_search(right_sums, complement):
return True
return False
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return True
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return False
# Example usage
arr = [3, 34, 4, 12, 5, 2]
target = 9
result = meet_in_the_middle(arr, target)
print(f"Subset with sum {target} exists: {result}")
This implementation demonstrates the core concepts of Meet in the Middle:
- We split the input array into two halves.
- We generate all possible subset sums for each half.
- We sort the subset sums for efficient searching.
- We iterate through one half and use binary search to find complementary sums in the other half.
5. Example Problems and Solutions
Let’s explore a few more problems where Meet in the Middle can be applied effectively:
5.1 The Knapsack Problem
Problem: Given a set of items with weights and values, determine the most valuable combination of items that can be carried in a knapsack with a weight limit.
def knapsack_meet_in_middle(weights, values, capacity):
n = len(weights)
left_half = list(zip(weights[:n//2], values[:n//2]))
right_half = list(zip(weights[n//2:], values[n//2:]))
def generate_combinations(items):
combinations = []
for i in range(1 << len(items)):
total_weight = 0
total_value = 0
for j in range(len(items)):
if i & (1 << j):
weight, value = items[j]
total_weight += weight
total_value += value
combinations.append((total_weight, total_value))
return combinations
left_combinations = generate_combinations(left_half)
right_combinations = generate_combinations(right_half)
right_combinations.sort()
max_value = 0
for left_weight, left_value in left_combinations:
if left_weight > capacity:
continue
remaining_capacity = capacity - left_weight
right_index = binary_search_upper_bound(right_combinations, remaining_capacity)
if right_index > 0:
max_value = max(max_value, left_value + right_combinations[right_index-1][1])
return max_value
def binary_search_upper_bound(arr, target):
left, right = 0, len(arr)
while left < right:
mid = (left + right) // 2
if arr[mid][0] <= target:
left = mid + 1
else:
right = mid
return left
# Example usage
weights = [10, 20, 30, 40, 50]
values = [60, 100, 120, 160, 200]
capacity = 50
result = knapsack_meet_in_middle(weights, values, capacity)
print(f"Maximum value: {result}")
5.2 Find Four Elements That Sum to a Given Value
Problem: Given an array of integers, find four elements that sum to a given target value.
def find_four_sum(arr, target):
n = len(arr)
pair_sums = {}
# Generate all possible pair sums
for i in range(n):
for j in range(i+1, n):
curr_sum = arr[i] + arr[j]
if curr_sum in pair_sums:
pair_sums[curr_sum].append((i, j))
else:
pair_sums[curr_sum] = [(i, j)]
# Check for complementary pairs
for i in range(n):
for j in range(i+1, n):
complement = target - (arr[i] + arr[j])
if complement in pair_sums:
for k, l in pair_sums[complement]:
if i != k and i != l and j != k and j != l:
return [arr[i], arr[j], arr[k], arr[l]]
return None
# Example usage
arr = [10, 2, 3, 4, 5, 9, 7, 8]
target = 23
result = find_four_sum(arr, target)
print(f"Four elements that sum to {target}: {result}")
6. Optimization Tips and Tricks
While Meet in the Middle is already an optimization technique, there are ways to further improve its efficiency:
- Use appropriate data structures: Choose the right data structure for storing and searching intermediate results. Hash tables can provide O(1) average-case lookup times.
- Prune unnecessary computations: If possible, avoid generating combinations that you know won’t contribute to the solution.
- Utilize bit manipulation: For subset generation, bit manipulation can be faster than recursive approaches.
- Balance the division: Try to divide the problem as evenly as possible to maximize the benefit of the technique.
- Consider memory-time tradeoffs: Sometimes, using more memory can significantly reduce computation time.
7. Comparison with Other Techniques
Meet in the Middle often serves as an alternative to other problem-solving techniques. Let’s compare it with some common approaches:
7.1 Meet in the Middle vs. Brute Force
Brute force explores all possible combinations, leading to an exponential time complexity (e.g., O(2^n) for subset problems). Meet in the Middle reduces this to O(2^(n/2)), which is a significant improvement for large n.
7.2 Meet in the Middle vs. Dynamic Programming
Dynamic Programming (DP) can solve many problems that Meet in the Middle addresses, often with better time complexity. However, Meet in the Middle can be simpler to implement and may use less memory. It’s particularly useful when the DP solution would require an impractical amount of memory.
7.3 Meet in the Middle vs. Divide and Conquer
While both techniques involve dividing the problem, Meet in the Middle specifically focuses on reducing the search space by combining results from two halves. Divide and Conquer typically solves subproblems recursively and may not always yield the same complexity improvements.
8. Real-world Applications
Meet in the Middle finds applications in various real-world scenarios:
- Cryptography: In cryptanalysis, Meet in the Middle attacks are used against certain types of encryption schemes.
- Optimization problems: In logistics and resource allocation, where finding optimal combinations is crucial.
- Bioinformatics: For analyzing genetic sequences and finding patterns in large datasets.
- Financial modeling: In portfolio optimization and risk assessment calculations.
9. Interview Preparation Strategies
If you’re preparing for technical interviews, especially for top tech companies, here are some strategies to master Meet in the Middle:
- Practice problem recognition: Learn to identify problems where Meet in the Middle could be applicable.
- Implement from scratch: Practice implementing the algorithm without relying on library functions.
- Analyze time and space complexity: Be prepared to discuss the efficiency gains and memory trade-offs.
- Combine with other techniques: Explore how Meet in the Middle can be used alongside other algorithms and data structures.
- Solve variations: Practice with different problem types to understand the versatility of the technique.
10. Conclusion
Meet in the Middle is a powerful algorithmic technique that can transform seemingly intractable problems into manageable ones. By dividing the search space and cleverly combining partial solutions, it offers a way to optimize certain types of algorithms significantly.
As you continue your journey in algorithmic problem-solving, keep Meet in the Middle in your toolkit. It may not be applicable to every problem, but when it fits, it can provide elegant and efficient solutions. Remember that mastering this technique, along with others, is key to becoming a well-rounded programmer and acing those technical interviews.
Practice implementing Meet in the Middle for various problems, analyze its performance, and understand its limitations. With time and experience, you’ll develop an intuition for when and how to apply this powerful technique effectively.
Happy coding, and may you always find the optimal solution right in the middle!