LinkedIn Technical Interview Prep: A Comprehensive Guide
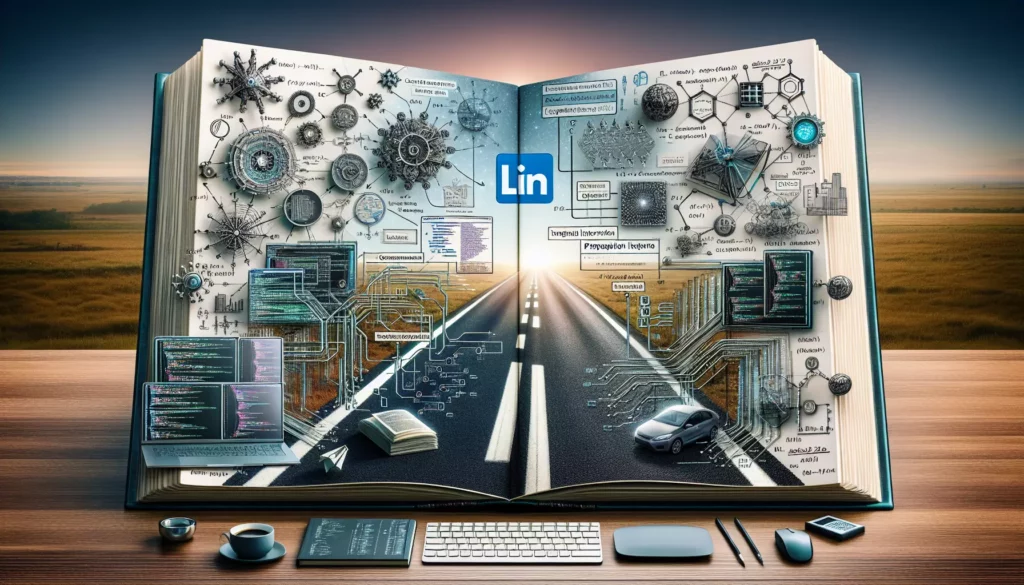
Preparing for a technical interview at LinkedIn can be a daunting task, but with the right approach and resources, you can significantly increase your chances of success. This comprehensive guide will walk you through the essential steps and strategies to ace your LinkedIn technical interview, covering everything from understanding the interview process to mastering key coding concepts and problem-solving techniques.
Table of Contents
- Understanding LinkedIn Technical Interviews
- Key Topics to Focus On
- Coding Practice and Resources
- Problem-Solving Strategies
- System Design and Architecture
- Behavioral Questions and Soft Skills
- Mock Interviews and Peer Practice
- Interview Day Tips
- Post-Interview Follow-up
- Conclusion
1. Understanding LinkedIn Technical Interviews
LinkedIn’s technical interview process typically consists of several rounds:
- Phone Screen: An initial conversation with a recruiter to discuss your background and the role.
- Technical Phone Interview: A coding interview conducted over the phone or video call, usually focusing on data structures and algorithms.
- On-site Interviews: Multiple rounds of face-to-face (or virtual) interviews, including:
- Coding interviews
- System design discussions
- Behavioral questions
The key to success is thorough preparation in all these areas. Let’s dive into each aspect in detail.
2. Key Topics to Focus On
To excel in LinkedIn’s technical interviews, you should have a strong grasp of the following topics:
Data Structures
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
Algorithms
- Sorting and Searching
- Recursion and Dynamic Programming
- Breadth-First Search (BFS) and Depth-First Search (DFS)
- Graph Algorithms
- Greedy Algorithms
Core Computer Science Concepts
- Object-Oriented Programming (OOP)
- Time and Space Complexity Analysis
- Operating Systems Basics
- Database Fundamentals
- Networking Principles
It’s crucial to not just memorize these concepts but to understand them deeply and be able to apply them to solve problems.
3. Coding Practice and Resources
Consistent practice is key to improving your coding skills and problem-solving abilities. Here are some resources and strategies to help you prepare:
Online Coding Platforms
- LeetCode: Offers a wide range of coding problems, including a section specifically for LinkedIn interview questions.
- HackerRank: Provides coding challenges and competitions to sharpen your skills.
- CodeSignal: Features a variety of coding tasks and assessments used by many tech companies.
Books
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Elements of Programming Interviews” by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash
- “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
Coding Practice Strategy
- Start with easy problems and gradually increase difficulty.
- Time yourself to simulate interview conditions.
- Practice explaining your thought process out loud.
- Review and optimize your solutions after solving problems.
- Learn multiple approaches to solve the same problem.
Remember, the goal is not just to solve problems but to understand the underlying patterns and techniques that can be applied to a wide range of scenarios.
4. Problem-Solving Strategies
Developing a systematic approach to problem-solving is crucial for technical interviews. Here’s a step-by-step strategy you can follow:
- Understand the Problem: Carefully read the question and ask clarifying questions if needed.
- Identify the Inputs and Outputs: Clearly define what you’re given and what you need to produce.
- Consider Edge Cases: Think about potential edge cases or special scenarios.
- Brainstorm Approaches: Consider multiple ways to solve the problem, starting with a brute-force approach.
- Choose an Approach: Select the most efficient solution that you can implement confidently.
- Plan Your Solution: Outline your approach before coding, possibly using pseudocode.
- Implement the Solution: Write clean, readable code.
- Test and Debug: Run through test cases and fix any issues.
- Optimize: If time allows, consider ways to improve your solution’s efficiency.
Let’s look at an example of how to apply this strategy to a common coding problem:
Example Problem: Two Sum
Given an array of integers nums and an integer target, return indices of the two numbers such that they add up to target. You may assume that each input would have exactly one solution, and you may not use the same element twice.
Here’s how we can approach this problem:
- Understand the Problem: We need to find two numbers in an array that sum up to a target value.
- Inputs and Outputs:
- Input: An array of integers (nums) and a target integer
- Output: Two indices of the numbers that add up to the target
- Edge Cases: Consider empty array, array with only one element, or no valid solution.
- Brainstorm Approaches:
- Brute Force: Check every pair of numbers (O(n^2) time complexity)
- Hash Table: Use a hash table to store complements (O(n) time complexity)
- Choose an Approach: Let’s go with the Hash Table approach for better efficiency.
- Plan the Solution: Use a hash map to store each number and its index. For each number, check if its complement exists in the hash map.
- Implement the Solution:
def twoSum(nums, target):
num_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
return [] # No solution found
- Test and Debug: Run through test cases like [2, 7, 11, 15] with target 9, and edge cases.
- Optimize: This solution is already optimized with O(n) time complexity and O(n) space complexity.
By following this structured approach, you demonstrate not only your coding skills but also your problem-solving abilities and thought process, which are highly valued in technical interviews.
5. System Design and Architecture
System design questions are a crucial part of LinkedIn’s technical interviews, especially for more senior positions. These questions assess your ability to design scalable, reliable, and efficient systems. Here are some key areas to focus on:
Core Concepts
- Scalability and Load Balancing
- Database Sharding and Partitioning
- Caching Strategies
- Microservices Architecture
- API Design
- Data Storage Solutions (SQL vs. NoSQL)
- Consistency and Availability Trade-offs
Preparation Tips
- Study existing large-scale systems and their architectures.
- Practice drawing system diagrams and explaining them clearly.
- Understand the trade-offs between different design choices.
- Be familiar with common design patterns and their use cases.
- Learn about LinkedIn’s technology stack and architecture.
System Design Interview Approach
- Clarify Requirements: Ask questions to understand the scope and constraints of the system.
- Define Core Features: Identify the most important functionalities to focus on.
- Estimate Scale: Consider the number of users, data volume, and traffic expectations.
- Design High-Level Architecture: Start with a basic design and iterate.
- Deep Dive into Components: Discuss specific components in detail as needed.
- Identify Bottlenecks: Consider potential issues and how to address them.
- Discuss Trade-offs: Explain the pros and cons of your design choices.
Example: Designing a URL Shortener
Let’s walk through a basic system design for a URL shortener service:
- Requirements:
- Shorten long URLs to short, unique aliases
- Redirect users from short URLs to original long URLs
- High availability and low latency
- Analytics for URL usage
- Core Features:
- URL shortening
- URL redirection
- Basic analytics
- Scale Estimation:
- Assume 100 million new URLs per month
- 1 billion redirects per month
- 5 years of data retention
- High-Level Design:
[Client] <--> [Load Balancer] <--> [Web Servers] <--> [Application Servers] ^ | [Database Cluster] ^ | [Cache Layer]
- Component Details:
- URL Shortening Algorithm: Use base62 encoding of an auto-incrementing ID
- Database: Use a NoSQL database like Cassandra for scalability
- Caching: Implement Redis for frequently accessed URLs
- Analytics: Use a separate data warehouse for analytics processing
- Bottlenecks and Solutions:
- High read traffic: Implement aggressive caching
- Database writes: Use write-behind caching and asynchronous updates for analytics
- Trade-offs:
- NoSQL vs SQL: Chose NoSQL for scalability at the cost of some consistency
- Caching: Improved performance but increased system complexity
This example demonstrates a structured approach to system design questions. Practice designing various systems to improve your skills in this area.
6. Behavioral Questions and Soft Skills
While technical skills are crucial, LinkedIn also places a high value on soft skills and cultural fit. Behavioral questions are designed to assess your past experiences, problem-solving abilities, teamwork, and alignment with LinkedIn’s culture and values.
Common Behavioral Question Themes
- Teamwork and collaboration
- Conflict resolution
- Leadership and initiative
- Handling failure or setbacks
- Adaptability and learning
- Project management and prioritization
Preparation Strategies
- Research LinkedIn’s Culture and Values: Familiarize yourself with LinkedIn’s mission, vision, and core values.
- Use the STAR Method: Structure your answers using the Situation, Task, Action, Result format.
- Prepare Specific Examples: Have concrete stories ready that demonstrate your skills and experiences.
- Practice Active Listening: Pay attention to the details of the question and address all parts in your response.
- Be Concise and Relevant: Keep your answers focused and relate them back to the role you’re applying for.
Example Behavioral Question and Response
Question: “Tell me about a time when you had to work with a difficult team member. How did you handle the situation?”
Answer using the STAR method:
- Situation: “In my previous role as a software engineer, I was part of a team working on a critical project to redesign our company’s main product interface.”
- Task: “One team member consistently missed deadlines and was often unresponsive to communications, which was putting our project timeline at risk.”
- Action: “I decided to approach the situation proactively:
- I scheduled a one-on-one meeting with the team member to understand if there were any underlying issues or challenges they were facing.
- During our discussion, I learned that they were overwhelmed with the technical aspects of their tasks.
- I offered to pair program with them on challenging parts and set up more frequent check-ins to provide support.
- I also worked with our project manager to adjust some task allocations to better match team members’ strengths.”
- Result: “As a result of these actions:
- The team member’s productivity improved significantly, and they started meeting deadlines consistently.
- Our team’s overall communication and collaboration improved.
- We completed the project on time and received positive feedback from stakeholders.
- This experience reinforced the importance of open communication and adaptability in team dynamics.”
This response demonstrates problem-solving skills, empathy, leadership initiative, and a commitment to team success – all valuable traits for a LinkedIn employee.
7. Mock Interviews and Peer Practice
One of the most effective ways to prepare for LinkedIn technical interviews is through mock interviews and peer practice. These simulations help you get comfortable with the interview format, improve your communication skills, and identify areas for improvement.
Benefits of Mock Interviews
- Reduce interview anxiety by familiarizing yourself with the process
- Practice articulating your thoughts clearly under pressure
- Receive feedback on your technical skills and communication style
- Identify and work on your weaknesses before the actual interview
How to Conduct Effective Mock Interviews
- Find a Practice Partner: This could be a colleague, friend in the industry, or a professional interview coach.
- Set the Stage: Recreate the interview environment as closely as possible, including using video calls for remote interviews.
- Use Realistic Questions: Base your practice on actual LinkedIn interview questions or similar technical problems.
- Time Your Sessions: Stick to realistic time constraints for each question or section.
- Provide and Receive Feedback: After the mock interview, discuss areas of strength and opportunities for improvement.
Online Resources for Mock Interviews
- Pramp: Offers free peer-to-peer mock interviews with other job seekers.
- InterviewBit: Provides a platform for mock interviews and coding practice.
- Interviewing.io: Offers anonymous technical interviews with engineers from top companies.
Peer Practice Strategies
- Form a Study Group: Connect with other candidates preparing for technical interviews.
- Rotate Roles: Take turns being the interviewer and the interviewee.
- Focus on Different Areas: Practice various aspects like coding, system design, and behavioral questions.
- Discuss Solutions: After each practice session, discuss different approaches to problems.
Example Mock Interview Scenario
Here’s a sample structure for a mock technical interview:
- Introduction (5 minutes): Brief overview of the candidate’s background and the role they’re interviewing for.
- Coding Problem (30 minutes): Present a coding challenge similar to those used in LinkedIn interviews. For example:
"Design a data structure that supports the following operations in O(1) time: - insert(x): Inserts an element x to the collection. - remove(x): Removes an element x from the collection if present. - getRandom(): Returns a random element from the current collection of elements."
Observe how the candidate approaches the problem, writes code, and explains their thought process.
- System Design Question (20 minutes): Ask a high-level system design question, such as:
"Design a simplified version of LinkedIn's news feed system."
Evaluate the candidate’s ability to break down the problem, consider scalability issues, and make design trade-offs.
- Behavioral Question (10 minutes): Ask a behavioral question like:
"Describe a situation where you had to make a difficult technical decision. How did you approach it, and what was the outcome?"
Assess the candidate’s communication skills, decision-making process, and ability to reflect on past experiences.
- Q&A (5 minutes): Allow time for the candidate to ask questions about the role or company.
- Feedback Session (10 minutes): Provide constructive feedback on technical skills, problem-solving approach, communication, and areas for improvement.
Regular practice with mock interviews will significantly improve your performance and confidence for the actual LinkedIn interview.
8. Interview Day Tips
When the big day arrives, being well-prepared and maintaining the right mindset can make a significant difference in your performance. Here are some essential tips to help you succeed on your LinkedIn interview day:
Before the Interview
- Get a Good Night’s Sleep: Ensure you’re well-rested and alert for the interview.
- Review Key Concepts: Do a quick review of important algorithms, data structures, and system design principles.
- Prepare Your Space: If it’s a virtual interview, set up a quiet, well-lit area with a stable internet connection.
- Test Your Tech: For virtual interviews, test your camera, microphone, and required software beforehand.
- Dress Appropriately: Wear professional attire, even for virtual interviews.
During the Interview
- Start with a Positive Attitude: Greet your interviewer warmly and show enthusiasm.
- Listen Carefully: Pay close attention to the questions and ask for clarification if needed.
- Think Aloud: Verbalize your thought process as you work through problems.
- Manage Your Time: Keep an eye on the clock and pace yourself appropriately.
- Stay Calm Under Pressure: If you get stuck, take a deep breath and approach the problem step by step.
- Be Open to Hints: If the interviewer offers a suggestion, consider it carefully.
- Ask Thoughtful Questions: Prepare insightful questions about the role, team, or company.
Technical Interview Best Practices
- Clarify the Problem: Ensure you fully understand the requirements before starting to code.
- Discuss Your Approach: Before diving into coding, explain your planned solution to the interviewer.
- Write Clean, Readable Code: Use proper indentation, meaningful variable names, and comments where necessary.
- Test Your Code: After implementing a solution, walk through some test cases to verify correctness.
- Optimize if Possible: If time allows, discuss potential optimizations or alternative approaches.
Handling Difficult Situations
- If You Don’t Know Something: Be honest about it, but explain how you would go about finding the answer.
- If You Make a Mistake: Stay calm, acknowledge the error, and work on correcting it.
- If You Get Stuck: Take a step back, break down the problem, and consider simpler cases or alternative approaches.
Virtual Interview Specific Tips
- Maintain Eye Contact: Look into the camera to create a sense of eye contact with the interviewer.
- Minimize Distractions: Turn off notifications on your device and ensure a quiet environment.
- Have a Backup Plan: Be prepared with a phone number to call in case of technical difficulties.
Remember, the interviewers at LinkedIn are not just evaluating your technical skills but also assessing how you approach problems, communicate your thoughts, and handle challenges. Stay confident, be yourself, and showcase your passion for technology and problem-solving.
9. Post-Interview Follow-up
The interview process doesn’t end when you walk out of the room or close the video call. Proper follow-up can leave a lasting positive impression and potentially influence the hiring decision. Here’s how to handle the post-interview phase effectively:
Immediate Actions
- Send a Thank-You Email: Within 24 hours of your interview, send a personalized thank-you email to your interviewer(s) or the recruiter.
- Reflect on the Interview: Take notes on the questions asked, your responses, and any areas where you feel you could improve.
- Address Any Missed Points: If you forgot to mention something important, you can briefly include it in your thank-you email.
Writing an Effective Thank-You Email
- Express gratitude for the interviewer’s time and the opportunity to learn about the role.
- Reiterate your interest in the position and how your skills align with it.
- Reference specific points from the interview to personalize the message.
- Keep it concise, professional, and error-free.
Example Thank-You Email
Subject: Thank You for the Software Engineer Interview
Dear [Interviewer's Name],
Thank you for taking the time to meet with me yesterday regarding the Software Engineer position at LinkedIn. I enjoyed our conversation about [specific topic discussed] and was excited to learn more about the challenges your team is tackling with [mentioned project or technology].
Our discussion reinforced my enthusiasm for the role and my confidence that my skills in [relevant skill], along with my experience in [relevant experience], would allow me to contribute effectively to your team's goals.
I was particularly intrigued by [something specific about LinkedIn or the role that was discussed]. It aligns well with my career aspirations and my passion for [relevant area of interest].
Thank you again for your time and consideration. I look forward to hearing about the next steps in the process. Please don't hesitate to contact me if you need any additional information.
Best regards,
[Your Name]
Following Up After the Thank-You Email
- Be Patient: Hiring processes can take time, especially at large companies like LinkedIn.
- Follow the Timeline: If the interviewer or recruiter provided a timeline for next steps, wait until after that date to follow up.
- Polite Persistence: If you haven’t heard back after the indicated timeline, it’s appropriate to send a polite follow-up email inquiring about the status of your application.
Example Follow-Up Email
Subject: Following Up on Software Engineer Application
Dear [Recruiter's Name],
I hope this email finds you well. I wanted to follow up on my application for the Software Engineer position at LinkedIn. I had the pleasure of interviewing with [Interviewer's Name] on [Date], and was told I might hear back about next steps by [Expected Date].
I remain very excited about the opportunity to join the LinkedIn team and contribute to [specific project or goal mentioned during the interview]. I'm writing to inquire if there are any updates on the status of my application or if you need any additional information from me.
Thank you for your time and consideration. I look forward to hearing from you.
Best regards,
[Your Name]
Continuing Your Preparation
While waiting for a response:
- Continue practicing coding and system design problems.
- Stay updated on LinkedIn’s latest products and tech news.
- Reflect on your interview performance and work on areas for improvement.
- Keep your options open and continue your job search process with other companies.
Remember, regardless of the outcome, each interview is a valuable learning experience. Use the insights gained to refine your skills and approach for future opportunities.
10. Conclusion
Preparing for a LinkedIn technical interview is a comprehensive process that requires dedication, practice, and a strategic approach. By focusing on key areas such as data structures, algorithms, system design, and behavioral skills, you can significantly increase your chances of success.
Remember these key takeaways:
- Consistent Practice: Regular coding practice on platforms like LeetCode and HackerRank is crucial.
- Holistic Preparation: Balance your focus between technical skills, problem-solving abilities, and soft skills.
- Understanding LinkedIn: Familiarize yourself with LinkedIn’s products, culture, and technical challenges.
- Communication Skills: Practice articulating your thoughts clearly, both for technical explanations and behavioral questions.
- Mock Interviews: Utilize mock interviews to simulate the actual interview experience and gain valuable feedback.
- Positive Attitude: Approach the interview with confidence, enthusiasm, and a growth mindset.
As you prepare, keep in mind that the goal of the interview process is not just to test your knowledge, but to assess your problem-solving approach, your ability to learn and adapt, and your potential to contribute to LinkedIn’s mission of connecting the world’s professionals.
While the interview process can be challenging, it’s also an opportunity to showcase your skills, learn about exciting projects at LinkedIn, and potentially join a team that’s making a significant impact in the tech industry.
Remember, every interview, regardless of the outcome, is a chance to grow and improve. Stay persistent, keep learning, and approach each opportunity with enthusiasm. With thorough preparation and the right mindset, you’ll be well-equipped to tackle your LinkedIn technical interview and take the next step in your career journey.
Best of luck with your preparation and your interview!