Kruskal’s Algorithm: Mastering the Minimum Spanning Tree
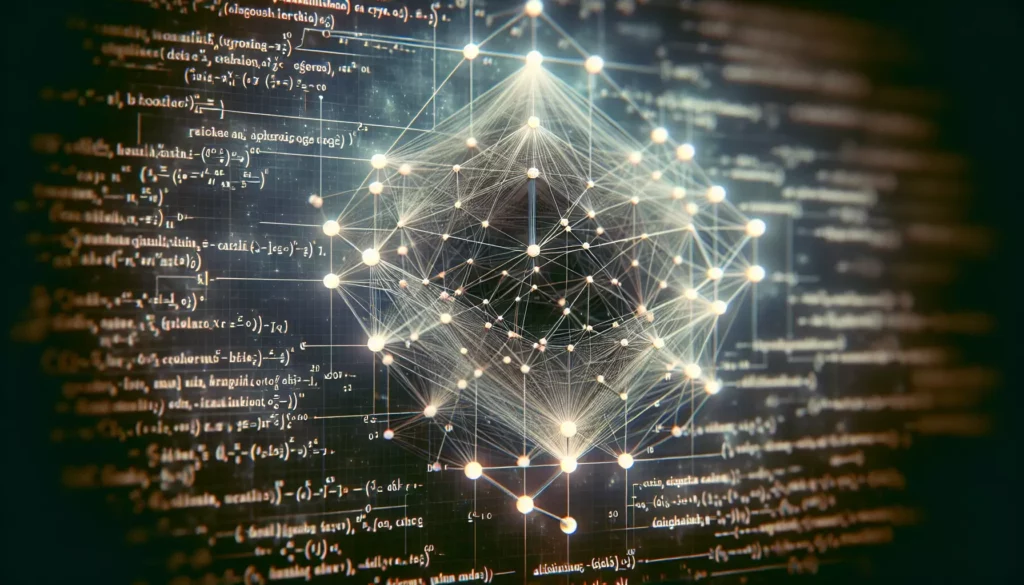
In the world of computer science and graph theory, Kruskal’s algorithm stands as a fundamental technique for finding the minimum spanning tree (MST) of a weighted, undirected graph. This algorithm, developed by Joseph Kruskal in 1956, has become an essential tool for solving various optimization problems in network design, clustering, and even in the realm of computer graphics. In this comprehensive guide, we’ll dive deep into Kruskal’s algorithm, exploring its inner workings, implementation, and real-world applications.
Understanding the Basics
What is a Minimum Spanning Tree?
Before we delve into Kruskal’s algorithm, it’s crucial to understand what a minimum spanning tree is. A spanning tree of a graph is a subgraph that includes all the vertices of the original graph and is a tree (i.e., it has no cycles). A minimum spanning tree is a spanning tree with the minimum total edge weight among all possible spanning trees of the graph.
For example, consider a graph representing cities connected by roads, where the edge weights represent distances. The minimum spanning tree would give us the shortest possible route that connects all cities without any loops.
The Concept Behind Kruskal’s Algorithm
Kruskal’s algorithm follows a greedy approach to construct the minimum spanning tree. The core idea is simple yet powerful:
- Sort all the edges of the graph in non-decreasing order of their weights.
- Pick the smallest edge and add it to the growing spanning tree if it doesn’t create a cycle.
- Repeat step 2 until the spanning tree includes all the vertices of the original graph.
This approach ensures that we always choose the most economical edge at each step, ultimately leading to the minimum spanning tree.
Implementing Kruskal’s Algorithm
Now that we understand the concept, let’s dive into the implementation of Kruskal’s algorithm. We’ll use Python for our example, but the principles can be applied to any programming language.
Data Structures Needed
To implement Kruskal’s algorithm efficiently, we need two main data structures:
- Disjoint Set (Union-Find): This data structure helps us quickly determine if adding an edge will create a cycle in the growing spanning tree.
- Priority Queue or Sorted List: This is used to store the edges sorted by their weights.
The Algorithm in Python
Let’s start with the implementation of the Disjoint Set data structure:
class DisjointSet:
def __init__(self, vertices):
self.parent = {v: v for v in vertices}
self.rank = {v: 0 for v in vertices}
def find(self, item):
if self.parent[item] != item:
self.parent[item] = self.find(self.parent[item])
return self.parent[item]
def union(self, x, y):
xroot = self.find(x)
yroot = self.find(y)
if self.rank[xroot] < self.rank[yroot]:
self.parent[xroot] = yroot
elif self.rank[xroot] > self.rank[yroot]:
self.parent[yroot] = xroot
else:
self.parent[yroot] = xroot
self.rank[xroot] += 1
Now, let’s implement Kruskal’s algorithm:
def kruskal_mst(graph):
edges = [(weight, u, v) for u in graph for v, weight in graph[u].items()]
edges.sort()
vertices = list(graph.keys())
disjoint_set = DisjointSet(vertices)
minimum_spanning_tree = []
for weight, u, v in edges:
if disjoint_set.find(u) != disjoint_set.find(v):
disjoint_set.union(u, v)
minimum_spanning_tree.append((u, v, weight))
return minimum_spanning_tree
This implementation takes a graph represented as a dictionary of dictionaries, where each key is a vertex, and its value is another dictionary representing its neighbors and the corresponding edge weights.
Time Complexity Analysis
The time complexity of Kruskal’s algorithm depends on the data structures used and the number of edges and vertices in the graph. Let’s break it down:
- Sorting the edges: O(E log E), where E is the number of edges
- Iterating through the sorted edges: O(E)
- Union-Find operations: O(α(V)) per operation, where α is the inverse Ackermann function (practically constant)
Therefore, the overall time complexity is O(E log E) or O(E log V), as E is at most V^2 for a dense graph. This makes Kruskal’s algorithm particularly efficient for sparse graphs.
Optimizations and Variations
While the basic implementation of Kruskal’s algorithm is quite efficient, there are several optimizations and variations that can be applied to improve its performance or adapt it to specific scenarios:
1. Path Compression in Union-Find
We’ve already implemented path compression in our find
method. This optimization flattens the tree structure of the disjoint set, reducing the time complexity of subsequent find operations.
2. Randomized Kruskal’s Algorithm
Instead of sorting all edges, we can randomly select edges and add them to the MST if they don’t create a cycle. This approach can be faster for dense graphs:
import random
def randomized_kruskal_mst(graph):
edges = [(weight, u, v) for u in graph for v, weight in graph[u].items()]
random.shuffle(edges)
vertices = list(graph.keys())
disjoint_set = DisjointSet(vertices)
minimum_spanning_tree = []
for weight, u, v in edges:
if disjoint_set.find(u) != disjoint_set.find(v):
disjoint_set.union(u, v)
minimum_spanning_tree.append((u, v, weight))
if len(minimum_spanning_tree) == len(vertices) - 1:
break
return minimum_spanning_tree
3. Borůvka’s Algorithm
Borůvka’s algorithm is another approach to finding the MST, which can be faster than Kruskal’s in certain scenarios. It works by repeatedly finding the minimum-weight edge for each component and merging components:
def boruvka_mst(graph):
vertices = list(graph.keys())
disjoint_set = DisjointSet(vertices)
minimum_spanning_tree = []
while len(set(disjoint_set.find(v) for v in vertices)) > 1:
min_edges = {}
for u in graph:
for v, weight in graph[u].items():
root_u, root_v = disjoint_set.find(u), disjoint_set.find(v)
if root_u != root_v:
if root_u not in min_edges or weight < min_edges[root_u][2]:
min_edges[root_u] = (u, v, weight)
for u, v, weight in min_edges.values():
if disjoint_set.find(u) != disjoint_set.find(v):
disjoint_set.union(u, v)
minimum_spanning_tree.append((u, v, weight))
return minimum_spanning_tree
Applications of Kruskal’s Algorithm
Kruskal’s algorithm and the concept of minimum spanning trees have a wide range of applications across various domains:
1. Network Design
One of the most common applications of Kruskal’s algorithm is in network design. For example:
- Telecommunications: Designing efficient cable or fiber optic networks to connect cities or buildings.
- Computer Networks: Optimizing the layout of local area networks (LANs) or wide area networks (WANs).
- Transportation: Planning road networks or public transit systems to minimize construction costs while ensuring connectivity.
2. Clustering and Data Analysis
Kruskal’s algorithm can be adapted for clustering in data analysis:
- Hierarchical Clustering: By running Kruskal’s algorithm and stopping at a certain threshold, we can create clusters of data points.
- Image Segmentation: In computer vision, MST-based methods can be used to segment images by treating pixels as vertices and color differences as edge weights.
3. Computer Graphics
In computer graphics and computational geometry, Kruskal’s algorithm finds applications in:
- Mesh Generation: Creating efficient triangulations for 3D modeling and rendering.
- Terrain Analysis: Analyzing digital elevation models to identify ridges and valleys.
4. Operations Research
Kruskal’s algorithm is useful in various optimization problems:
- Supply Chain Optimization: Minimizing transportation costs in logistics networks.
- Facility Location: Determining optimal locations for facilities to serve a set of customers or points.
Challenges and Limitations
While Kruskal’s algorithm is powerful and widely applicable, it’s important to be aware of its limitations and challenges:
1. Memory Usage
For very large graphs, storing all edges sorted by weight can consume significant memory. This can be mitigated by using external sorting algorithms for extremely large datasets.
2. Dynamic Graphs
Kruskal’s algorithm is designed for static graphs. If the graph structure or edge weights change frequently, recomputing the MST from scratch can be inefficient. In such cases, dynamic graph algorithms might be more suitable.
3. Parallel Computing
While the basic Kruskal’s algorithm is inherently sequential due to its greedy nature, there have been efforts to parallelize it for use in distributed systems or on multi-core processors. However, achieving significant speedup can be challenging.
4. Negative Weight Cycles
Kruskal’s algorithm assumes that the graph doesn’t contain negative weight cycles. If such cycles exist, the concept of a minimum spanning tree becomes ill-defined.
Advanced Topics and Research Directions
For those looking to dive deeper into the world of minimum spanning trees and graph algorithms, here are some advanced topics and current research directions:
1. Approximate Minimum Spanning Trees
In some applications, finding an exact MST might be too time-consuming. Researchers have developed algorithms to find approximate MSTs much faster, sacrificing a small amount of optimality for speed.
2. Metric MST Problem
When the graph’s edge weights satisfy the triangle inequality (as in Euclidean space), specialized algorithms can find the MST more efficiently than general methods.
3. Minimum Spanning Tree Verification
Given a spanning tree, how quickly can we verify if it’s the minimum spanning tree? This problem has connections to the theory of proof systems and verification algorithms.
4. Online and Streaming Algorithms
Researchers are developing algorithms to maintain an MST (or an approximation of it) as edges are added or removed from the graph in real-time, without recomputing from scratch.
5. Quantum Algorithms for MST
With the advent of quantum computing, there’s ongoing research into quantum algorithms for graph problems, including finding minimum spanning trees potentially faster than classical algorithms.
Conclusion
Kruskal’s algorithm is a cornerstone of graph theory and algorithm design, offering an elegant and efficient solution to the minimum spanning tree problem. Its simplicity, coupled with its wide range of applications, makes it an essential tool in the toolkit of any computer scientist or software engineer.
As we’ve explored in this article, understanding Kruskal’s algorithm opens doors to solving complex optimization problems across various domains. From network design to data analysis, the principles behind this algorithm continue to find new applications in our increasingly connected and data-driven world.
Whether you’re preparing for a technical interview, working on a network optimization project, or simply expanding your algorithmic knowledge, mastering Kruskal’s algorithm is a valuable investment. It not only solves a specific problem but also introduces you to fundamental concepts like greedy algorithms, disjoint sets, and graph theory that are applicable in many other areas of computer science.
As you continue your journey in algorithm design and problem-solving, remember that Kruskal’s algorithm is just the beginning. The field of graph algorithms is vast and ever-evolving, with new challenges and optimizations constantly emerging. Keep exploring, practicing, and applying these concepts to real-world problems, and you’ll find yourself well-equipped to tackle complex computational challenges in your career.
Happy coding, and may your spanning trees always be minimal!