Fast and Slow Pointers: A Powerful Technique for Solving Linked List Problems
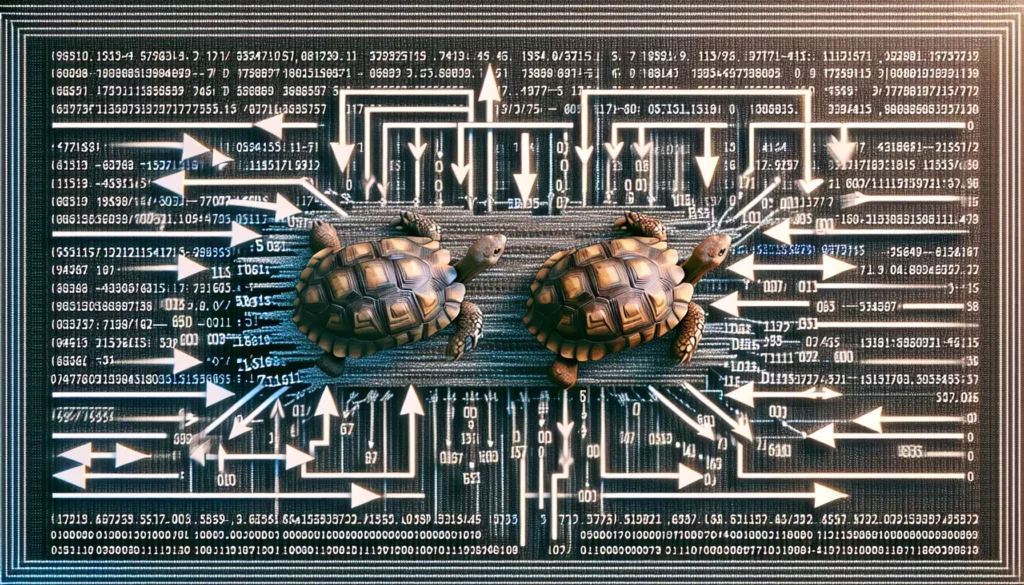
In the world of coding interviews and algorithmic problem-solving, linked lists are a common data structure that often appear in various challenges. One particularly useful technique for tackling linked list problems is the “Fast and Slow Pointers” approach, also known as the “Tortoise and Hare” algorithm. This method can be incredibly effective for detecting cycles, finding middle elements, and solving other linked list-related problems efficiently.
In this comprehensive guide, we’ll dive deep into the fast and slow pointers technique, exploring its applications, implementation, and how it can give you an edge in coding interviews. Whether you’re preparing for technical interviews at top tech companies or simply looking to improve your algorithmic skills, understanding this concept is crucial for any aspiring software engineer.
Table of Contents
- Understanding Fast and Slow Pointers
- How Fast and Slow Pointers Work
- Common Applications of Fast and Slow Pointers
- Detecting Cycles in a Linked List
- Finding the Middle of a Linked List
- Checking if a Linked List is a Palindrome
- Finding the Nth Node from the End
- Time and Space Complexity Analysis
- Tips for Using Fast and Slow Pointers in Interviews
- Practice Problems and Solutions
- Conclusion
1. Understanding Fast and Slow Pointers
The fast and slow pointers technique involves using two pointers that traverse a linked list at different speeds. As the name suggests, one pointer (the “fast” pointer) moves faster than the other (the “slow” pointer). This difference in speed creates a gap between the two pointers, which can be leveraged to solve various problems efficiently.
The core idea behind this technique is that by the time the fast pointer reaches the end of the list (or detects a cycle), the slow pointer will be at a strategically important position, such as the middle of the list or at the start of a cycle.
2. How Fast and Slow Pointers Work
The basic implementation of fast and slow pointers follows these steps:
- Initialize two pointers,
slow
andfast
, to the head of the linked list. - Move the
slow
pointer one step at a time (slow = slow.next). - Move the
fast
pointer two steps at a time (fast = fast.next.next). - Continue this process until the fast pointer reaches the end of the list or until a specific condition is met (e.g., detecting a cycle).
Here’s a simple implementation in Python:
def fast_slow_pointers(head):
slow = head
fast = head
while fast and fast.next:
slow = slow.next
fast = fast.next.next
# Additional logic based on the specific problem
# For example, detecting a cycle:
if slow == fast:
return True # Cycle detected
return False # No cycle found or end of list reached
This basic structure can be adapted to solve various linked list problems, as we’ll see in the following sections.
3. Common Applications of Fast and Slow Pointers
The fast and slow pointers technique has several common applications in linked list problems. Some of the most frequently encountered uses include:
- Detecting cycles in a linked list
- Finding the middle node of a linked list
- Determining if a linked list is a palindrome
- Finding the nth node from the end of a linked list
- Detecting the start of a cycle in a linked list
Let’s explore each of these applications in detail.
4. Detecting Cycles in a Linked List
One of the most common applications of the fast and slow pointers technique is detecting cycles in a linked list. This problem is often referred to as the “Floyd’s Cycle-Finding Algorithm” or the “Tortoise and Hare Algorithm”.
The idea is simple: if there’s a cycle in the linked list, the fast pointer will eventually catch up to the slow pointer, and they’ll meet at some node within the cycle.
Here’s an implementation of cycle detection using fast and slow pointers:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def has_cycle(head):
if not head or not head.next:
return False
slow = head
fast = head.next
while slow != fast:
if not fast or not fast.next:
return False
slow = slow.next
fast = fast.next.next
return True
In this implementation, we start the fast pointer one step ahead of the slow pointer. If there’s no cycle, the fast pointer will reach the end of the list. If there is a cycle, the pointers will meet, indicating the presence of a cycle.
5. Finding the Middle of a Linked List
Another useful application of fast and slow pointers is finding the middle node of a linked list. This technique is particularly efficient because it allows us to find the middle in a single pass through the list.
The idea is to move the fast pointer twice as fast as the slow pointer. When the fast pointer reaches the end of the list, the slow pointer will be at the middle.
Here’s an implementation to find the middle node:
def find_middle(head):
if not head or not head.next:
return head
slow = head
fast = head
while fast.next and fast.next.next:
slow = slow.next
fast = fast.next.next
return slow
This function returns the middle node of the linked list. For lists with an even number of nodes, it returns the first of the two middle nodes.
6. Checking if a Linked List is a Palindrome
The fast and slow pointers technique can also be used to determine if a linked list is a palindrome. This problem combines finding the middle of the list with reversing the second half and comparing it to the first half.
Here’s an implementation to check if a linked list is a palindrome:
def is_palindrome(head):
if not head or not head.next:
return True
# Find the middle node
slow = fast = head
while fast.next and fast.next.next:
slow = slow.next
fast = fast.next.next
# Reverse the second half of the list
second_half = reverse_list(slow.next)
first_half = head
# Compare the two halves
while second_half:
if first_half.val != second_half.val:
return False
first_half = first_half.next
second_half = second_half.next
return True
def reverse_list(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
This solution first finds the middle of the list using the fast and slow pointers. Then it reverses the second half of the list and compares it with the first half to determine if the list is a palindrome.
7. Finding the Nth Node from the End
The fast and slow pointers technique can be adapted to find the nth node from the end of a linked list in a single pass. The idea is to maintain a gap of n nodes between the fast and slow pointers.
Here’s an implementation to find the nth node from the end:
def find_nth_from_end(head, n):
if not head or n < 1:
return None
fast = slow = head
# Move fast pointer n steps ahead
for _ in range(n):
if not fast:
return None # n is greater than the length of the list
fast = fast.next
# Move both pointers until fast reaches the end
while fast:
slow = slow.next
fast = fast.next
return slow
This function first moves the fast pointer n steps ahead. Then it moves both pointers until the fast pointer reaches the end. At this point, the slow pointer will be at the nth node from the end.
8. Time and Space Complexity Analysis
One of the key advantages of the fast and slow pointers technique is its efficiency in terms of both time and space complexity.
Time Complexity: In all the applications we’ve discussed, the time complexity is O(n), where n is the number of nodes in the linked list. This is because we traverse the list only once, even though we’re using two pointers.
Space Complexity: The space complexity for these algorithms is O(1), which is constant space. We only use a fixed amount of extra space (for the two pointers) regardless of the size of the input list.
This combination of linear time complexity and constant space complexity makes the fast and slow pointers technique highly efficient and desirable for solving linked list problems, especially in scenarios where memory usage is a concern.
9. Tips for Using Fast and Slow Pointers in Interviews
When using the fast and slow pointers technique in coding interviews, keep these tips in mind:
- Recognize the pattern: Look for problems involving linked lists where you need to find a specific position (like the middle) or detect a cycle.
- Handle edge cases: Always consider edge cases such as empty lists or lists with only one or two nodes.
- Explain your approach: Clearly explain the intuition behind using two pointers and how they help solve the problem efficiently.
- Be careful with pointer movements: Ensure you’re moving the pointers correctly and check for null pointers to avoid runtime errors.
- Consider the return value: Depending on the problem, you might need to return a boolean, a node, or modify the list in place.
- Practice variations: Familiarize yourself with different variations of the technique, as interviewers might ask you to modify the basic approach slightly.
10. Practice Problems and Solutions
To solidify your understanding of the fast and slow pointers technique, here are some practice problems along with their solutions:
Problem 1: Detect the start of a cycle in a linked list
def detect_cycle_start(head):
if not head or not head.next:
return None
# Detect cycle
slow = fast = head
while fast and fast.next:
slow = slow.next
fast = fast.next.next
if slow == fast:
break
else:
return None # No cycle
# Find cycle start
slow = head
while slow != fast:
slow = slow.next
fast = fast.next
return slow # Cycle start node
Problem 2: Remove the Nth node from the end of the list
def remove_nth_from_end(head, n):
dummy = ListNode(0)
dummy.next = head
fast = slow = dummy
# Move fast pointer n steps ahead
for _ in range(n):
fast = fast.next
# Move both pointers until fast reaches the end
while fast.next:
slow = slow.next
fast = fast.next
# Remove the nth node
slow.next = slow.next.next
return dummy.next
Problem 3: Determine if a linked list has an even or odd number of nodes
def is_even_length(head):
if not head:
return True
fast = head
while fast and fast.next:
fast = fast.next.next
return fast is None
These practice problems demonstrate different applications and variations of the fast and slow pointers technique. By solving these and similar problems, you’ll gain confidence in applying this powerful method to a wide range of linked list challenges.
11. Conclusion
The fast and slow pointers technique is a powerful tool in any programmer’s arsenal, especially when dealing with linked list problems. Its ability to solve complex problems with linear time complexity and constant space complexity makes it an invaluable approach in coding interviews and real-world programming scenarios.
By mastering this technique, you’ll be well-equipped to tackle a variety of linked list problems efficiently. Remember to practice regularly and explore different variations of the problems to strengthen your understanding and problem-solving skills.
As you continue your journey in algorithmic problem-solving, consider how the principles behind fast and slow pointers might apply to other data structures or problem domains. The ability to think creatively about pointer manipulation and traversal can open up new approaches to solving complex computational problems.
Keep practicing, stay curious, and happy coding!