Essential JavaScript Interview Questions You Must Know
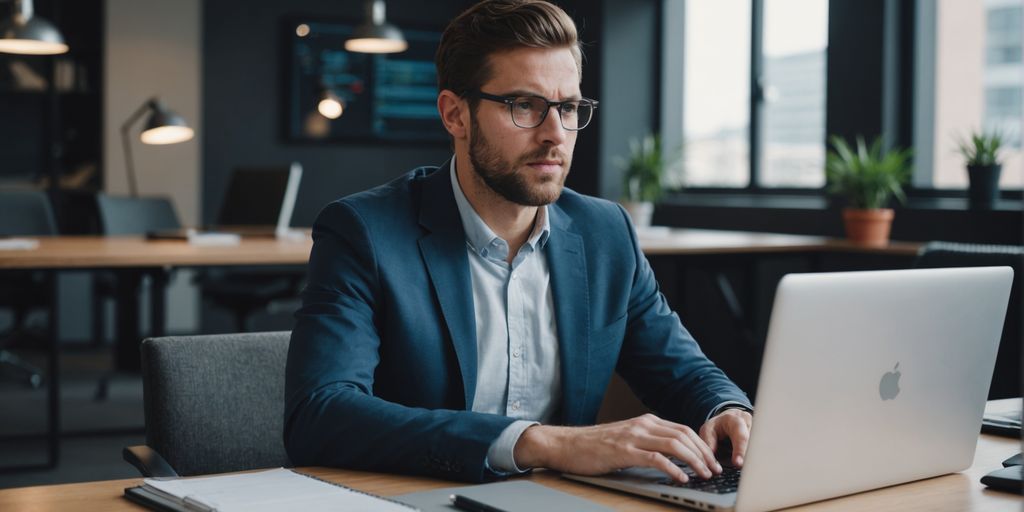
Preparing for a JavaScript interview can be challenging. You need to know the basics and understand more complex ideas. This guide will help you get ready by covering important topics and common questions.
Key Takeaways
- Understand JavaScript basics like data types, variables, and functions.
- Learn advanced topics such as closures, promises, and modules.
- Get familiar with common interview questions about ‘var’, ‘let’, ‘const’, and ‘this’.
- Practice debugging and error handling in JavaScript.
- Work on real-world projects to improve your skills.
Understanding JavaScript Fundamentals
Data Types and Variables
JavaScript is a high-level, interpreted programming language that allows you to create interactive web pages and applications. It has six primitive data types:
- Number
- String
- Boolean
- Null
- Undefined
- Symbol
Additionally, it has two compound data types:
- Object
- Array
Control Structures and Loops
Control structures in JavaScript help manage the flow of the program. Common control structures include:
- if-else statements
- switch statements
- try-catch blocks
Loops are used to execute a block of code multiple times. The main types of loops are:
- for loop
- while loop
- do-while loop
Functions and Scope
Functions are blocks of code designed to perform a particular task. They can be defined using the function keyword or as arrow functions. Scope determines the accessibility of variables. JavaScript has two types of scope:
- Global Scope
- Local Scope
Understanding these fundamentals is crucial for mastering JavaScript and building dynamic web applications.
Advanced JavaScript Concepts
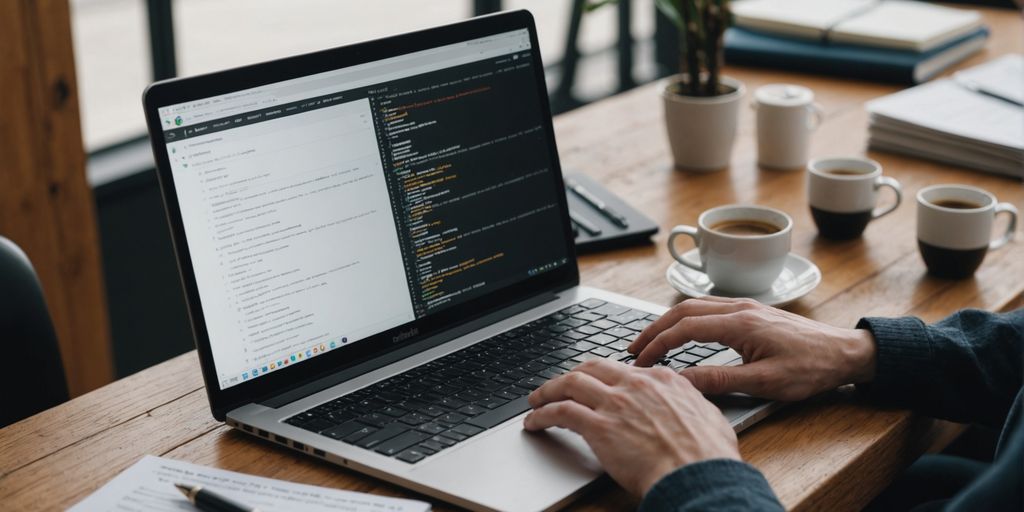
Closures and Lexical Environment
Closures are functions that remember the environment in which they were created. This means they have access to variables from their containing function, even after that function has finished executing. Closures are essential for creating private variables and functions.
Promises and Asynchronous Programming
Promises are used to handle asynchronous operations in JavaScript. They represent a value that may be available now, or in the future, or never. Promises have three states: pending, fulfilled, and rejected. Using promises, you can avoid callback hell and write cleaner, more readable code.
Modules and Imports
Modules in JavaScript allow you to break your code into separate files, making it easier to manage and maintain. You can export functions, objects, or primitives from one module and import them into another. This helps in organizing code and reusing components across different parts of your application.
Understanding these advanced concepts is crucial for any JavaScript developer looking to build robust and efficient applications.
Common JavaScript Interview Questions
Difference Between var, let, and const
Understanding the differences between var
, let
, and const
is crucial for any JavaScript developer. var
is function-scoped, while let
and const
are block-scoped. This means that variables declared with var
are accessible throughout the function, whereas those declared with let
and const
are only accessible within the block they are defined in. Additionally, const
is used for variables that should not be reassigned after their initial assignment.
Understanding Hoisting
Hoisting is a JavaScript mechanism where variables and function declarations are moved to the top of their containing scope during the compile phase. This means you can use functions and variables before they are declared in the code. However, only the declarations are hoisted, not the initializations. For example:
console.log(myVar); // undefined
var myVar = 5;
In this example, the declaration of myVar
is hoisted, but its assignment is not.
Explaining ‘this’ Keyword
The this
keyword in JavaScript refers to the object it belongs to. Its value depends on where it is used:
- In a method,
this
refers to the owner object. - Alone,
this
refers to the global object (in browsers, it’s the window object). - In a function,
this
refers to the global object. - In an event,
this
refers to the element that received the event.
Understanding how this
works is essential for mastering JavaScript, especially when dealing with object-oriented programming and event handling.
Mastering these common JavaScript interview questions can significantly boost your confidence and performance in technical interviews.
Debugging and Error Handling in JavaScript
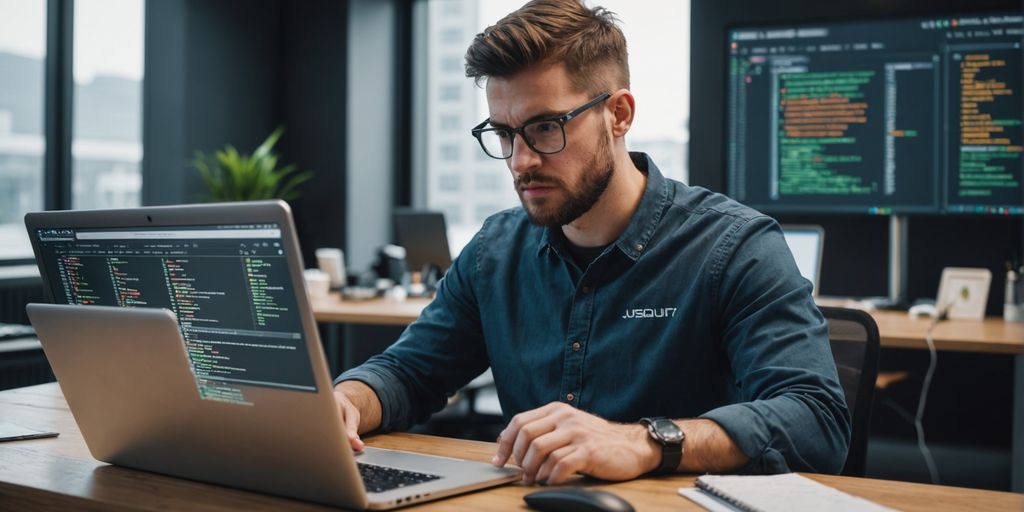
Common JavaScript Errors
JavaScript errors can be grouped into three main types:
- Syntax Errors: These happen when the code breaks the rules of the JavaScript language. For example, a missing semicolon or an unclosed string.
- Logical Errors: These occur when the code doesn’t do what you expect, but there is no error message. They are usually caused by a mistake in the code’s logic.
- Runtime Errors: These happen when the code is correct in terms of syntax and logic, but an error still occurs during execution. For example, accessing an undefined variable or calling a function that doesn’t exist.
Debugging Techniques
To debug a JavaScript program, follow these steps:
- Identify the Error: Use the JavaScript console in the browser’s developer tools. The console will show any errors that occur during script execution, such as syntax errors, type errors, and reference errors.
- Examine the Code: Look at the code line by line to find the source of the issue.
- Use Breakpoints: Set breakpoints in your code to pause execution and inspect variables and the call stack.
- Use console.error(): When logging errors to the console inside a catch block, using
console.error()
rather thanconsole.log()
is advised for debugging. It formats the error message and makes it easier to spot.
Using Browser Developer Tools
Browser developer tools are essential for debugging JavaScript. They offer features like:
- Console: Displays errors and allows you to run JavaScript code.
- Sources: Lets you view and edit your code, set breakpoints, and step through code.
- Network: Shows network requests and responses, useful for debugging AJAX calls.
- Elements: Allows you to inspect and modify the DOM and CSS.
Debugging is a crucial skill for any JavaScript developer. It helps you find and fix errors quickly, making your code more reliable and efficient.
JavaScript in Practice
Building Projects with JavaScript
Building projects is one of the best ways to master JavaScript effortlessly. Start with small projects like a to-do list or a simple calculator. As you get more comfortable, move on to more complex projects like a weather app or a chat application. This hands-on approach helps you understand how different parts of JavaScript work together.
JavaScript Frameworks and Libraries
JavaScript frameworks and libraries can make your coding life easier. Some popular ones include React, Angular, and Vue.js. These tools help you build complex applications more efficiently. They come with pre-built functions and components, so you don’t have to start from scratch.
Real-world Coding Challenges
To prepare for real-world coding challenges, practice with interactive JavaScript exercises. These exercises often come with practice quizzes and track your progress. They help you get ready for real-world coding challenges by simulating the types of problems you might face in a job.
Practicing coding challenges regularly can significantly improve your problem-solving skills and prepare you for job interviews.
JavaScript Performance Optimization
Memory Management
Efficient memory management is crucial for optimizing JavaScript performance. Minimizing memory leaks can significantly improve the speed and responsiveness of your applications. Here are some tips:
- Avoid global variables as they remain in memory throughout the application’s lifecycle.
- Use local variables and functions to limit the scope and lifespan of variables.
- Regularly nullify references to objects that are no longer needed.
Efficient DOM Manipulation
Manipulating the DOM is one of the most expensive operations in JavaScript. To enhance performance, follow these guidelines:
- Minimize the number of DOM accesses and updates. Batch DOM changes together to reduce reflows and repaints.
- Use document fragments to make multiple changes to the DOM at once.
- Cache DOM references to avoid repeated lookups.
Reducing DOM manipulation is essential for writing more efficient code.
Optimizing Loops and Functions
Loops and functions are fundamental in JavaScript, but they can also be performance bottlenecks if not optimized. Consider these practices:
- Use
for
loops instead offorEach
for better performance in large datasets. - Avoid using nested loops when possible; try to flatten the data structure instead.
- Optimize recursive functions by using memoization to store previously computed results.
By following these tips, you can ensure your JavaScript code runs faster and more efficiently.
Conclusion
Preparing for a JavaScript interview can be a challenging yet rewarding journey. By focusing on the essential questions and concepts, you can build a strong foundation and boost your confidence. Remember to practice coding regularly, understand the core principles, and stay updated with the latest advancements in JavaScript. With dedication and the right preparation, you’ll be well-equipped to impress your interviewers and land your dream job. Good luck!
Frequently Asked Questions
What is JavaScript and why is it important?
JavaScript is a programming language used to make web pages interactive. It’s essential because it runs on nearly every website and is crucial for web development.
How does ‘this’ keyword work in JavaScript?
‘This’ refers to the object it belongs to. In a method, it refers to the owner object. Alone, it refers to the global object. In a function, it refers to the global object, but in strict mode, it is undefined.
What are the differences between var, let, and const?
‘Var’ is function-scoped and can be redeclared. ‘Let’ is block-scoped and can’t be redeclared. ‘Const’ is also block-scoped but can’t be reassigned or redeclared.
Can you explain what a closure is in JavaScript?
A closure is a function that remembers its outer variables and can access them. It’s useful for creating private variables and functions.
What is the purpose of promises in JavaScript?
Promises handle asynchronous operations. They allow you to attach callbacks for success or failure, making code easier to manage and read.
How do you debug JavaScript code?
You can debug using browser developer tools. These tools let you set breakpoints, inspect variables, and step through code to find and fix issues.