Edmonds-Karp Algorithm: Mastering Maximum Flow in Network Graphs
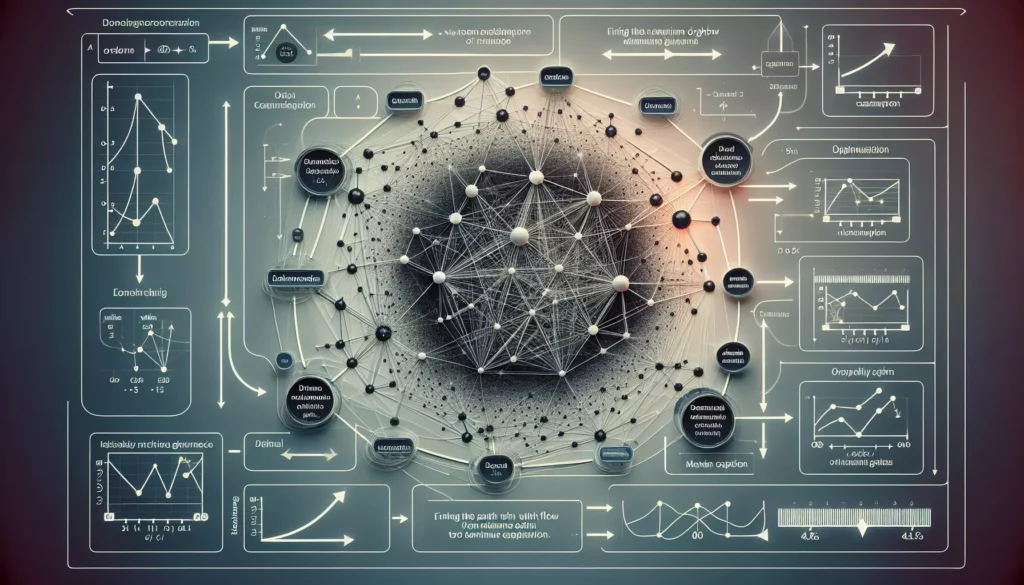
Welcome to another in-depth AlgoCademy tutorial! Today, we’re diving into one of the most fascinating algorithms in graph theory and network flow: the Edmonds-Karp algorithm. Whether you’re preparing for technical interviews at top tech companies or simply looking to expand your algorithmic toolkit, understanding this algorithm is crucial for tackling complex network flow problems.
What is the Edmonds-Karp Algorithm?
The Edmonds-Karp algorithm is an implementation of the Ford-Fulkerson method for computing the maximum flow in a flow network. Developed by Jack Edmonds and Richard Karp in 1972, this algorithm provides an efficient solution to the max-flow problem, which has applications in various fields such as transportation networks, telecommunications, and even bipartite matching.
Key Features of Edmonds-Karp:
- It uses Breadth-First Search (BFS) to find augmenting paths
- Time complexity: O(VE²), where V is the number of vertices and E is the number of edges
- Guaranteed to terminate for networks with integer capacities
Understanding Network Flow
Before we dive into the algorithm itself, let’s review some fundamental concepts of network flow:
Flow Network
A flow network is a directed graph where each edge has a capacity and a flow. The flow represents the amount of “stuff” (data, water, traffic, etc.) moving through the edge, while the capacity is the maximum amount that can flow through that edge.
Source and Sink
In a flow network, we have two special nodes:
- Source (s): The node where flow originates
- Sink (t): The node where flow terminates
Maximum Flow Problem
The goal is to find the maximum amount of flow that can be pushed from the source to the sink while respecting the capacity constraints of each edge.
How Edmonds-Karp Works
The Edmonds-Karp algorithm follows these main steps:
- Initialize the flow in all edges to 0
- While there exists an augmenting path from source to sink:
- Find an augmenting path using BFS
- Compute the bottleneck capacity (minimum residual capacity along the path)
- Update the flow along the path
- Return the total flow
Augmenting Path
An augmenting path is a path from source to sink in the residual graph where each edge has positive residual capacity. The residual capacity of an edge is the difference between its capacity and current flow.
Breadth-First Search (BFS)
Edmonds-Karp uses BFS to find augmenting paths. This choice guarantees that the algorithm always finds the shortest augmenting path, which leads to its polynomial time complexity.
Implementing Edmonds-Karp in Python
Let’s implement the Edmonds-Karp algorithm in Python. We’ll use an adjacency matrix to represent our graph for simplicity, but in practice, an adjacency list might be more efficient for sparse graphs.
from collections import deque
def edmonds_karp(graph, source, sink):
n = len(graph) # Number of nodes
flow = 0
while True:
# Find an augmenting path using BFS
parent = [-1] * n
parent[source] = -2
queue = deque([source])
while queue:
u = queue.popleft()
for v in range(n):
if parent[v] == -1 and graph[u][v] > 0:
parent[v] = u
queue.append(v)
if v == sink:
break
# If no augmenting path is found, we're done
if parent[sink] == -1:
break
# Compute the bottleneck capacity
path_flow = float('inf')
v = sink
while v != source:
u = parent[v]
path_flow = min(path_flow, graph[u][v])
v = u
# Update the flow
v = sink
while v != source:
u = parent[v]
graph[u][v] -= path_flow
graph[v][u] += path_flow
v = u
flow += path_flow
return flow
# Example usage
graph = [
[0, 16, 13, 0, 0, 0],
[0, 0, 10, 12, 0, 0],
[0, 4, 0, 0, 14, 0],
[0, 0, 9, 0, 0, 20],
[0, 0, 0, 7, 0, 4],
[0, 0, 0, 0, 0, 0]
]
source = 0
sink = 5
max_flow = edmonds_karp(graph, source, sink)
print(f"The maximum flow is: {max_flow}")
This implementation follows the steps we outlined earlier. Let’s break it down:
- We use a while loop to keep finding augmenting paths until no more exist.
- BFS is implemented using a queue (deque for efficiency) to find the shortest augmenting path.
- We keep track of the parent of each node to reconstruct the path.
- Once we find a path to the sink, we compute the bottleneck capacity.
- We then update the flow along this path, both forward and backward (for residual edges).
- The process repeats until no more augmenting paths are found.
Time Complexity Analysis
The time complexity of Edmonds-Karp is O(VE²), where V is the number of vertices and E is the number of edges in the graph. Here’s why:
- Each BFS takes O(E) time.
- There can be at most O(VE) augmenting paths because:
- Each augmenting path increases the flow by at least 1.
- The maximum flow is at most O(E * C), where C is the maximum capacity.
- The length of each augmenting path is at least one more than the previous one (due to BFS always finding the shortest path).
- Therefore, the total number of BFS operations is O(VE).
- Thus, the overall time complexity is O(E) * O(VE) = O(VE²).
Space Complexity
The space complexity of the Edmonds-Karp algorithm is O(V + E), which comes from:
- O(V²) for the adjacency matrix representation of the graph (can be O(E) with an adjacency list)
- O(V) for the queue used in BFS
- O(V) for the parent array
Practical Applications
The Edmonds-Karp algorithm and its underlying max-flow concept have numerous real-world applications:
1. Network Routing
In computer networks, max-flow algorithms can help optimize data routing, ensuring maximum throughput between different nodes.
2. Bipartite Matching
The algorithm can be used to solve assignment problems, such as matching job applicants to positions or students to classes.
3. Image Segmentation
In computer vision, max-flow algorithms are used for separating foreground from background in images.
4. Transportation Systems
Optimizing the flow of vehicles in road networks or goods in supply chains.
5. Project Scheduling
Determining the maximum number of tasks that can be completed given resource constraints.
Comparison with Other Algorithms
While Edmonds-Karp is a powerful algorithm, it’s worth comparing it with other approaches to the max-flow problem:
Ford-Fulkerson Method
Edmonds-Karp is actually an implementation of the Ford-Fulkerson method. The key difference is that Ford-Fulkerson doesn’t specify how to find augmenting paths, while Edmonds-Karp uses BFS.
Dinic’s Algorithm
Dinic’s algorithm is an improvement over Edmonds-Karp, with a time complexity of O(V²E). It uses the concept of blocking flows and level graphs to find augmenting paths more efficiently.
Push-Relabel Algorithm
This algorithm takes a different approach, working with “preflows” instead of augmenting paths. It has a better time complexity of O(V³) or O(V²√E) depending on the implementation.
Common Pitfalls and Tips
When implementing or using the Edmonds-Karp algorithm, keep these points in mind:
- Graph Representation: While we used an adjacency matrix in our example, an adjacency list can be more efficient for sparse graphs.
- Integer Overflow: Be cautious with large capacities that might cause integer overflow. Consider using long integers or implementing arbitrary precision arithmetic if needed.
- Floating-Point Precision: If dealing with floating-point capacities, be aware of potential precision issues.
- Negative Capacities: The algorithm assumes non-negative capacities. Ensure your input graph meets this requirement.
- Multiple Sources/Sinks: If your problem has multiple sources or sinks, you can add a super-source or super-sink to transform it into a standard max-flow problem.
Practice Problems
To solidify your understanding of the Edmonds-Karp algorithm and max-flow problems, try these practice problems:
- Basic Implementation: Implement the Edmonds-Karp algorithm from scratch and test it on various small graphs.
- Maximum Bipartite Matching: Use Edmonds-Karp to solve a bipartite matching problem, such as assigning tasks to workers.
- Image Segmentation: Implement a simple image segmentation algorithm using max-flow concepts.
- Network Bottleneck: Given a network with capacities, find the bottleneck that limits the maximum flow.
- Multi-source, Multi-sink: Modify the algorithm to handle multiple sources and sinks in a network.
Conclusion
The Edmonds-Karp algorithm is a powerful tool in the world of network flow problems. Its guaranteed polynomial-time complexity and relatively simple implementation make it a popular choice for solving max-flow problems in various domains.
As you continue your journey in algorithmic problem-solving, remember that understanding algorithms like Edmonds-Karp not only prepares you for technical interviews but also equips you with valuable problem-solving skills applicable to real-world scenarios.
Keep practicing, and don’t hesitate to explore more advanced flow algorithms like Dinic’s or Push-Relabel. Happy coding, and may your flows always be maximum!