Critical Connections in a Network: Understanding Bridges in Graph Theory
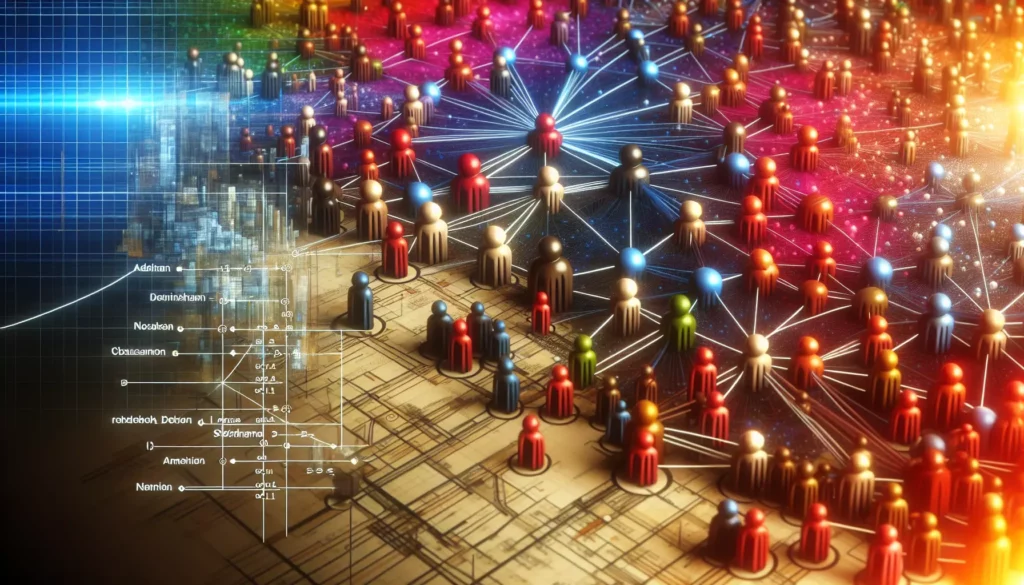
In the vast landscape of computer science and network analysis, understanding the concept of critical connections, also known as bridges in graph theory, is crucial. This concept plays a significant role in various applications, from network infrastructure to social network analysis. In this comprehensive guide, we’ll dive deep into the world of critical connections, exploring their importance, algorithms to detect them, and real-world applications.
What are Critical Connections?
Critical connections, or bridges, are edges in a graph whose removal would disconnect the graph into two or more components. In other words, these are the links that, if broken, would disrupt the flow of information or connectivity within a network.
To better understand this concept, let’s visualize a simple graph:
A --- B --- C
| |
D --- E
In this graph, the edge B-C is a critical connection. If we remove it, the graph splits into two separate components: {A, B, D, E} and {C}. No other edge in this graph has this property, making B-C the only bridge in this example.
Why are Critical Connections Important?
Understanding critical connections is vital for several reasons:
- Network Reliability: In computer networks, identifying bridges helps in assessing the network’s vulnerability. If a critical connection fails, it can lead to network partitioning.
- Infrastructure Planning: In urban planning and transportation, knowing the critical roads or bridges helps in disaster preparedness and infrastructure improvement.
- Social Network Analysis: In social networks, bridges can represent key connections between different communities or groups.
- Biological Networks: In protein interaction networks, critical connections can represent crucial interactions that, if disrupted, could significantly affect biological processes.
Algorithms to Find Critical Connections
Several algorithms can be used to identify critical connections in a graph. Let’s explore two popular approaches:
1. Tarjan’s Algorithm
Tarjan’s algorithm is an efficient method to find bridges in a graph. It uses depth-first search (DFS) and works in O(V+E) time complexity, where V is the number of vertices and E is the number of edges.
Here’s a high-level overview of how Tarjan’s algorithm works:
- Perform a DFS traversal of the graph.
- For each vertex, keep track of:
- Discovery time: When the vertex was first visited
- Low-link value: The lowest discovery time reachable from the vertex
- An edge (u, v) is a bridge if the low-link value of v is greater than the discovery time of u.
Let’s implement Tarjan’s algorithm in Python:
from collections import defaultdict
class Graph:
def __init__(self, vertices):
self.V = vertices
self.graph = defaultdict(list)
self.time = 0
def add_edge(self, u, v):
self.graph[u].append(v)
self.graph[v].append(u)
def bridge_util(self, u, visited, parent, low, disc, bridges):
visited[u] = True
disc[u] = self.time
low[u] = self.time
self.time += 1
for v in self.graph[u]:
if not visited[v]:
parent[v] = u
self.bridge_util(v, visited, parent, low, disc, bridges)
low[u] = min(low[u], low[v])
if low[v] > disc[u]:
bridges.append((u, v))
elif v != parent[u]:
low[u] = min(low[u], disc[v])
def find_bridges(self):
visited = [False] * self.V
disc = [float("Inf")] * self.V
low = [float("Inf")] * self.V
parent = [-1] * self.V
bridges = []
for i in range(self.V):
if not visited[i]:
self.bridge_util(i, visited, parent, low, disc, bridges)
return bridges
# Example usage
g = Graph(5)
g.add_edge(1, 0)
g.add_edge(0, 2)
g.add_edge(2, 1)
g.add_edge(0, 3)
g.add_edge(3, 4)
print("Bridges in the graph:")
print(g.find_bridges())
This implementation creates a Graph class with methods to add edges and find bridges. The find_bridges()
method initializes the necessary data structures and calls bridge_util()
for each unvisited vertex. The bridge_util()
method performs the DFS traversal and identifies the bridges.
2. Union-Find Algorithm
Another approach to finding critical connections is using the Union-Find data structure. This method involves removing each edge from the graph and checking if the graph remains connected.
Here’s a Python implementation using the Union-Find algorithm:
class UnionFind:
def __init__(self, n):
self.parent = list(range(n))
self.rank = [0] * n
def find(self, x):
if self.parent[x] != x:
self.parent[x] = self.find(self.parent[x])
return self.parent[x]
def union(self, x, y):
px, py = self.find(x), self.find(y)
if px == py:
return False
if self.rank[px] < self.rank[py]:
self.parent[px] = py
elif self.rank[px] > self.rank[py]:
self.parent[py] = px
else:
self.parent[py] = px
self.rank[px] += 1
return True
def critical_connections(n, connections):
uf = UnionFind(n)
edge_set = set(map(tuple, (map(sorted, connections))))
result = []
for u, v in edge_set:
uf_copy = UnionFind(n)
for x, y in edge_set:
if (x, y) != (u, v):
uf_copy.union(x, y)
if uf_copy.find(u) != uf_copy.find(v):
result.append([u, v])
return result
# Example usage
n = 4
connections = [[0,1],[1,2],[2,0],[1,3]]
print(critical_connections(n, connections))
This implementation uses a Union-Find data structure to efficiently check the connectivity of the graph. For each edge, we create a copy of the graph without that edge and check if it remains connected. If not, the edge is a critical connection.
Time Complexity Analysis
Let’s compare the time complexities of the two algorithms we’ve discussed:
- Tarjan’s Algorithm: O(V + E), where V is the number of vertices and E is the number of edges. This algorithm is very efficient as it only requires a single pass through the graph.
- Union-Find Algorithm: O(E * (V + E)), where E is the number of edges and V is the number of vertices. For each edge, we potentially iterate through all other edges, making this approach less efficient for large graphs.
For most practical purposes, Tarjan’s algorithm is preferred due to its linear time complexity.
Real-World Applications
Understanding critical connections has numerous practical applications across various fields:
1. Network Infrastructure
In computer networks, identifying critical connections helps in:
- Designing robust network topologies
- Planning redundancy to prevent single points of failure
- Prioritizing maintenance and upgrades for crucial links
2. Social Network Analysis
In social networks, bridges can represent:
- Key individuals connecting different communities
- Weak ties that are crucial for information flow between groups
- Potential points of intervention for information dissemination or containment
3. Transportation Systems
In transportation networks, critical connections help in:
- Identifying crucial roads or bridges for emergency planning
- Optimizing traffic flow and reducing congestion
- Prioritizing infrastructure investments
4. Biological Networks
In biological systems, understanding critical connections aids in:
- Identifying key protein interactions in cellular networks
- Understanding the spread of diseases in populations
- Analyzing ecological networks and food webs
Challenges and Considerations
While the concept of critical connections is powerful, there are some challenges and considerations to keep in mind:
1. Dynamic Networks
Many real-world networks are dynamic, with connections forming and breaking over time. Algorithms for finding critical connections in dynamic graphs are an active area of research.
2. Weighted Graphs
In some scenarios, edges may have different weights or importance. Adapting bridge-finding algorithms to consider edge weights can provide more nuanced insights.
3. Scalability
As networks grow larger, the computational cost of finding critical connections increases. Developing efficient algorithms for massive graphs remains a challenge.
4. Approximation Algorithms
For very large graphs, approximation algorithms that identify most, but not all, critical connections might be more practical than exact algorithms.
Future Directions
The study of critical connections continues to evolve. Some exciting areas for future research include:
- Quantum Algorithms: Exploring how quantum computing might accelerate the discovery of critical connections in large graphs.
- Machine Learning Approaches: Developing ML models to predict critical connections based on graph structure and dynamics.
- Temporal Graphs: Extending the concept of critical connections to graphs that change over time.
- Hypergraphs: Investigating critical connections in hypergraphs, where edges can connect more than two vertices.
Conclusion
Critical connections, or bridges, are fundamental concepts in graph theory with wide-ranging applications. From ensuring the robustness of computer networks to understanding social dynamics, the ability to identify these crucial links provides valuable insights across various domains.
As we’ve explored in this article, there are efficient algorithms like Tarjan’s method to find these connections, each with its own strengths and use cases. Understanding these algorithms and their implementations is crucial for anyone working with network analysis, whether in computer science, social sciences, or biological systems.
As networks continue to grow in size and complexity, the importance of identifying and analyzing critical connections will only increase. Whether you’re a software engineer designing resilient systems, a data scientist analyzing social networks, or a researcher studying complex biological interactions, the concept of critical connections provides a powerful tool for understanding and optimizing interconnected systems.
By mastering these concepts and algorithms, you’ll be well-equipped to tackle a wide range of problems in network analysis and graph theory. As you continue your journey in coding education and programming skills development, remember that understanding these fundamental algorithms is key to solving complex real-world problems and excelling in technical interviews at top tech companies.