Case Study: How Jane Got an Offer from Google
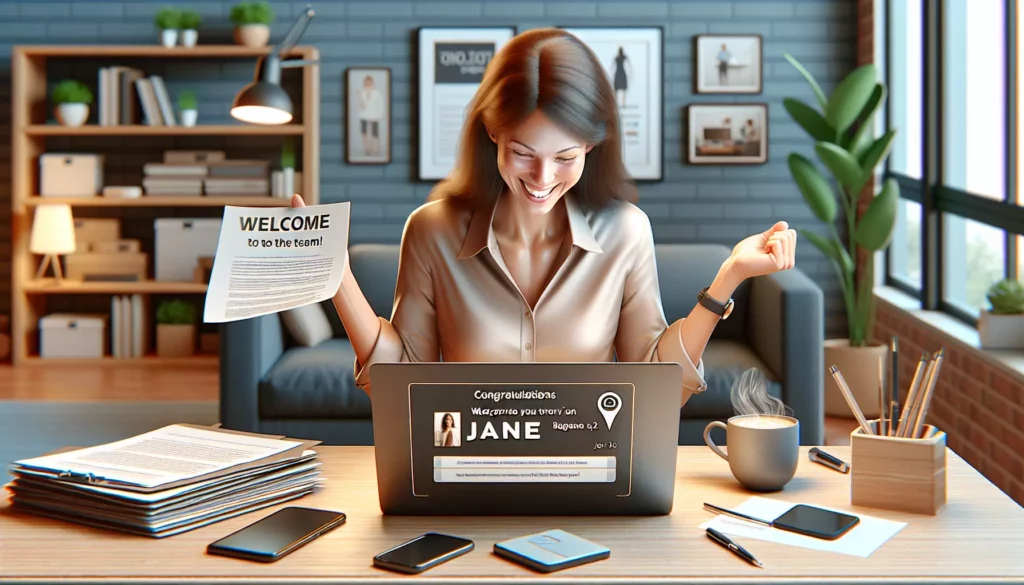
In the competitive world of tech, landing a job at a prestigious company like Google is often seen as the pinnacle of success for many aspiring software engineers. Today, we’re going to dive into the inspiring journey of Jane, a self-taught programmer who managed to secure a coveted position at Google. Her story is not just one of personal triumph, but also a testament to the power of dedication, strategic learning, and leveraging the right resources. Let’s explore how Jane transformed from a coding novice to a Google employee, and what lessons we can learn from her experience.
Jane’s Background: Starting from Scratch
Jane’s journey began much like many others in the tech world. She wasn’t a computer science graduate, nor did she have any formal training in programming. In fact, Jane had a background in marketing and had been working in that field for several years. However, she always had a fascination with technology and a curiosity about how things worked behind the scenes.
At the age of 28, Jane decided it was time for a career change. She had heard about the opportunities in the tech industry and was intrigued by the creative and problem-solving aspects of programming. With no prior experience, she embarked on her coding journey, determined to break into the field and eventually land a job at a top tech company.
The First Steps: Learning the Basics
Jane’s first challenge was to learn the fundamentals of programming. She started with free online resources, watching YouTube tutorials and reading coding blogs. However, she quickly realized that while these resources were helpful, she needed a more structured approach to truly progress.
This is when Jane discovered AlgoCademy. The platform’s interactive coding tutorials and step-by-step guidance provided her with the structure she needed. She started with the basics of Python, learning about variables, data types, control structures, and functions.
Here’s an example of one of the first Python programs Jane wrote:
def greet(name):
print(f"Hello, {name}! Welcome to the world of programming.")
greet("Jane")
This simple function taught Jane about defining functions, using parameters, and string formatting. It was a small step, but it marked the beginning of her programming journey.
Building a Strong Foundation
As Jane progressed through AlgoCademy’s curriculum, she realized the importance of building a strong foundation in computer science concepts. She dedicated time to understanding data structures and algorithms, recognizing their crucial role in technical interviews at companies like Google.
Jane spent several months working through AlgoCademy’s data structures course, learning about arrays, linked lists, stacks, queues, trees, and graphs. She found the platform’s visual explanations particularly helpful in grasping these abstract concepts.
For example, when learning about binary search trees, Jane implemented the following code:
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = Node(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = Node(value)
else:
self._insert_recursive(node.left, value)
else:
if node.right is None:
node.right = Node(value)
else:
self._insert_recursive(node.right, value)
# Usage
bst = BinarySearchTree()
bst.insert(5)
bst.insert(3)
bst.insert(7)
bst.insert(1)
bst.insert(9)
This implementation helped Jane understand the structure of a binary search tree and how elements are inserted to maintain the tree’s properties.
Mastering Algorithms
With a solid understanding of data structures, Jane moved on to algorithms. She recognized that algorithmic thinking was crucial not just for passing technical interviews, but for becoming a proficient problem solver in her future role.
Jane spent considerable time on AlgoCademy’s algorithm challenges, starting with basic sorting and searching algorithms and gradually moving to more complex topics like dynamic programming and graph algorithms.
One of the algorithms Jane found particularly challenging but rewarding was the implementation of Dijkstra’s algorithm for finding the shortest path in a graph:
import heapq
def dijkstra(graph, start):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return distances
# Example usage
graph = {
'A': {'B': 4, 'C': 2},
'B': {'D': 3, 'E': 1},
'C': {'B': 1, 'D': 5},
'D': {'E': 2},
'E': {}
}
print(dijkstra(graph, 'A'))
Implementing and understanding this algorithm not only improved Jane’s coding skills but also enhanced her problem-solving abilities, preparing her for the challenges she would face in technical interviews.
Practice Makes Perfect: Mock Interviews and Coding Challenges
As Jane’s skills improved, she realized that knowing the concepts wasn’t enough. She needed to practice applying her knowledge under pressure, simulating real interview conditions. This is where AlgoCademy’s mock interview feature proved invaluable.
Jane participated in numerous mock interviews, facing a variety of coding challenges similar to those asked in actual Google interviews. These sessions helped her in several ways:
- Improved her ability to communicate her thought process while coding
- Enhanced her speed and accuracy in solving problems
- Helped her manage time effectively during coding challenges
- Exposed her to a wide range of problem types, preparing her for any question that might come up
One of the mock interview questions that Jane found particularly challenging was implementing a Least Recently Used (LRU) cache. Here’s the solution she came up with after several attempts:
from collections import OrderedDict
class LRUCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = OrderedDict()
def get(self, key):
if key not in self.cache:
return -1
else:
self.cache.move_to_end(key)
return self.cache[key]
def put(self, key, value):
if key in self.cache:
self.cache.move_to_end(key)
self.cache[key] = value
if len(self.cache) > self.capacity:
self.cache.popitem(last=False)
# Usage
cache = LRUCache(2)
cache.put(1, 1)
cache.put(2, 2)
print(cache.get(1)) # returns 1
cache.put(3, 3) # evicts key 2
print(cache.get(2)) # returns -1 (not found)
cache.put(4, 4) # evicts key 1
print(cache.get(1)) # returns -1 (not found)
print(cache.get(3)) # returns 3
print(cache.get(4)) # returns 4
This problem tested Jane’s understanding of data structures, her ability to optimize for time complexity, and her skill in implementing a practical caching mechanism. It was challenges like these that prepared her for the rigorous technical interviews at Google.
Leveraging AI-Powered Assistance
One of the features that set AlgoCademy apart for Jane was its AI-powered assistance. When she got stuck on a problem or needed clarification on a concept, she could turn to the AI tutor for help. This feature provided Jane with:
- Personalized explanations tailored to her learning style
- Step-by-step guidance on complex problems
- Suggestions for optimizing her code
- Recommendations for related topics to explore
The AI assistant was particularly helpful when Jane was learning about system design, a crucial topic for Google interviews. When she struggled with designing a distributed cache, the AI provided her with a high-level overview and then guided her through the implementation details.
Beyond Coding: Soft Skills and Interview Preparation
While technical skills were crucial, Jane recognized that succeeding in a Google interview required more than just coding prowess. She used AlgoCademy’s resources to prepare for other aspects of the interview process:
- Behavioral Interviews: Jane practiced answering common behavioral questions, focusing on her problem-solving skills, teamwork experiences, and ability to handle challenges.
- System Design: She studied system design principles and practiced designing scalable systems, a key component of senior-level interviews at Google.
- Company Research: Jane thoroughly researched Google’s history, culture, and recent developments, preparing herself to answer and ask informed questions during the interviews.
- Communication Skills: Through mock interviews, Jane honed her ability to clearly explain her thought process and technical decisions.
The Application Process
After months of preparation, Jane felt ready to apply to Google. She took the following steps:
- Resume Preparation: Jane crafted a resume that highlighted her newly acquired skills, personal projects, and relevant experience. She used AlgoCademy’s resume review feature to ensure her resume was ATS-friendly and highlighted her strengths effectively.
- Online Application: She submitted her application through Google’s careers website, making sure to tailor her cover letter to the specific role she was applying for.
- Online Assessment: Jane was invited to complete an online coding assessment. Thanks to her extensive practice on AlgoCademy, she was able to solve the problems efficiently and accurately.
- Phone Screen: She passed the online assessment and was invited for a phone screen with a Google engineer. The interview involved coding on a shared document, where Jane had to solve a medium-difficulty algorithm problem.
- On-site Interviews: After succeeding in the phone screen, Jane was invited for a full day of on-site interviews at Google. This consisted of multiple rounds of technical interviews, a system design interview, and a behavioral interview.
The Google Interviews: Putting Skills to the Test
Jane’s on-site interview day at Google was intense but exciting. Here’s a breakdown of her experience:
1. Coding Interviews
Jane had three coding interviews, each lasting about 45 minutes. The questions ranged from medium to hard difficulty and covered various topics:
- A graph traversal problem that required implementing a modified version of breadth-first search
- A dynamic programming question involving optimizing a sequence of operations
- A string manipulation problem that tested her ability to optimize for both time and space complexity
In each interview, Jane made sure to:
- Clarify the problem requirements before starting to code
- Think out loud and explain her approach
- Write clean, well-commented code
- Discuss the time and space complexity of her solutions
- Propose and implement optimizations when possible
Here’s an example of how Jane approached one of the coding problems:
# Problem: Find the longest palindromic substring in a given string
class Solution:
def longestPalindrome(self, s: str) -> str:
if not s:
return ""
start = 0
max_length = 1
# Helper function to expand around center
def expand_around_center(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
for i in range(len(s)):
# Check for odd-length palindromes
length1 = expand_around_center(i, i)
# Check for even-length palindromes
length2 = expand_around_center(i, i + 1)
length = max(length1, length2)
if length > max_length:
start = i - (length - 1) // 2
max_length = length
return s[start:start + max_length]
# Test the solution
solution = Solution()
print(solution.longestPalindrome("babad")) # Output: "bab" or "aba"
print(solution.longestPalindrome("cbbd")) # Output: "bb"
Jane explained her approach, discussed the time complexity (O(n^2)), and even suggested potential optimizations, such as Manacher’s algorithm for O(n) time complexity.
2. System Design Interview
The system design interview was particularly challenging for Jane, given her non-traditional background. However, her preparation on AlgoCademy paid off. She was asked to design a distributed file storage system similar to Dropbox.
Jane’s approach included:
- Clarifying requirements and constraints
- Estimating the scale of the system
- Designing the high-level architecture
- Diving into specific components like the metadata database, file chunking, and synchronization mechanisms
- Discussing potential bottlenecks and how to address them
While she didn’t have all the answers, Jane’s structured approach and willingness to learn impressed the interviewer.
3. Behavioral Interview
In the behavioral interview, Jane was asked about her background, her transition into programming, and how she handles challenges. She used the STAR (Situation, Task, Action, Result) method to structure her responses, focusing on her problem-solving skills and her ability to learn quickly.
Jane also took the opportunity to ask thoughtful questions about Google’s culture, development opportunities, and the specific team she was interviewing for. This demonstrated her genuine interest in the company and the role.
The Offer and Reflection
A week after her on-site interviews, Jane received the call she had been hoping for – Google was extending her an offer! As she reflected on her journey, several key factors stood out as crucial to her success:
- Structured Learning: AlgoCademy’s curriculum provided Jane with a clear path from beginner to interview-ready, ensuring she didn’t miss any crucial concepts.
- Consistent Practice: Daily coding challenges and mock interviews on AlgoCademy helped Jane build confidence and improve her problem-solving skills.
- Comprehensive Preparation: Beyond just coding, Jane’s preparation included system design, behavioral interviews, and company research.
- Leveraging AI Assistance: The AI-powered tutor on AlgoCademy provided personalized help when Jane got stuck, accelerating her learning.
- Persistence and Dedication: Jane’s journey took over a year of consistent effort, showing that with dedication, even those without a traditional CS background can succeed.
Lessons for Aspiring Google Engineers
Jane’s success story offers valuable insights for others aspiring to join Google or other top tech companies:
- Start with a Strong Foundation: Ensure you have a solid understanding of computer science fundamentals, including data structures and algorithms.
- Practice, Practice, Practice: Consistent problem-solving is key. Aim to solve at least one coding challenge daily.
- Simulate Real Interview Conditions: Use platforms like AlgoCademy to participate in mock interviews and get comfortable with the pressure of coding while explaining your thought process.
- Don’t Neglect Soft Skills: Communication, teamwork, and problem-solving abilities are just as important as technical skills.
- Understand the Company: Research Google’s culture, products, and recent developments. This knowledge will help you during interviews and show your genuine interest.
- Leverage AI and Other Resources: Use AI-powered tools like those offered by AlgoCademy to get personalized assistance and accelerate your learning.
- Be Persistent: Remember that the journey to Google often involves setbacks. Learn from each experience and keep improving.
Conclusion
Jane’s journey from a marketing professional to a Google engineer is a testament to the power of dedication, strategic learning, and leveraging the right resources. Her story demonstrates that with the right approach and tools like AlgoCademy, even those without a traditional computer science background can achieve their dreams of working at top tech companies.
While every individual’s path will be unique, the core principles of Jane’s success – consistent practice, comprehensive preparation, and leveraging AI-assisted learning – can be applied by anyone aspiring to elevate their programming career.
Remember, the journey to becoming a Google engineer is as much about personal growth and problem-solving skills as it is about coding proficiency. By following in Jane’s footsteps and utilizing resources like AlgoCademy, you too can turn your dream of working at Google into a reality. The path may be challenging, but with persistence and the right approach, it’s certainly achievable. Best of luck on your coding journey!