Avoid These Coding Interview Pitfalls to Improve Faster
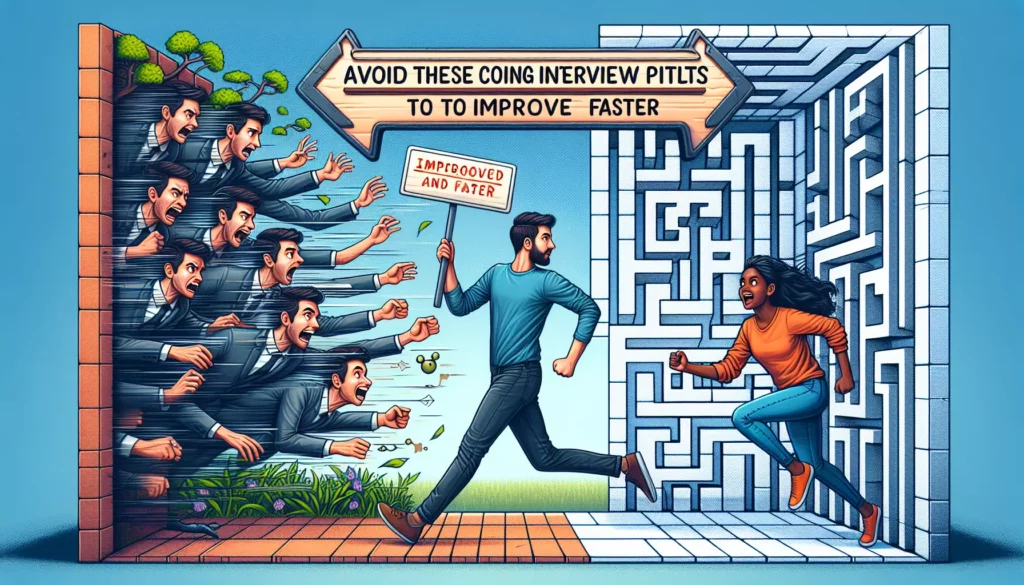
Coding interviews can be daunting, especially when you’re aiming for positions at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). As you prepare for these challenging interviews, it’s crucial to not only focus on what you should do but also be aware of common pitfalls that can hinder your progress. In this comprehensive guide, we’ll explore the most frequent mistakes candidates make during coding interviews and provide actionable strategies to avoid them, helping you improve faster and increase your chances of success.
1. Rushing to Code Without a Plan
One of the biggest mistakes candidates make is jumping straight into coding without taking the time to understand the problem and devise a strategy. This approach often leads to inefficient solutions or, worse, completely missing the point of the question.
Why it’s a problem:
- Leads to suboptimal solutions
- Wastes valuable interview time
- Shows poor problem-solving skills
How to avoid it:
- Take a few minutes to analyze the problem thoroughly
- Ask clarifying questions to ensure you understand the requirements
- Discuss your approach with the interviewer before coding
- Outline your solution on paper or a whiteboard
Remember, interviewers are more interested in your thought process than seeing you type code immediately. By taking the time to plan, you demonstrate critical thinking and methodical problem-solving skills.
2. Neglecting Time and Space Complexity
Many candidates focus solely on getting a working solution without considering the efficiency of their code. In coding interviews, especially for FAANG companies, the time and space complexity of your solution is crucial.
Why it’s a problem:
- Inefficient solutions may not scale for large inputs
- Shows lack of understanding of algorithmic efficiency
- Misses opportunities to demonstrate advanced problem-solving skills
How to avoid it:
- Always analyze the time and space complexity of your solution
- Discuss trade-offs between different approaches
- Practice optimizing your solutions for better complexity
- Learn common time complexity classes (O(1), O(log n), O(n), O(n log n), O(n^2), etc.)
Here’s a simple example to illustrate the importance of considering time complexity:
// Inefficient solution: O(n^2)
def find_sum_pair(arr, target):
for i in range(len(arr)):
for j in range(i+1, len(arr)):
if arr[i] + arr[j] == target:
return [i, j]
return []
// Efficient solution: O(n)
def find_sum_pair_optimized(arr, target):
seen = {}
for i, num in enumerate(arr):
complement = target - num
if complement in seen:
return [seen[complement], i]
seen[num] = i
return []
The optimized solution uses a hash map to achieve linear time complexity, demonstrating a better understanding of data structures and algorithms.
3. Poor Communication During the Interview
Coding interviews are not just about writing code; they’re also about communicating your thoughts and ideas effectively. Many candidates make the mistake of staying silent while coding or failing to explain their reasoning.
Why it’s a problem:
- Interviewers can’t follow your thought process
- Missed opportunities to showcase problem-solving skills
- Difficulty in receiving hints or guidance from the interviewer
How to avoid it:
- Practice thinking out loud while solving problems
- Explain your approach and reasoning as you code
- Ask for feedback or clarification when needed
- Use clear and concise language to describe your solution
Remember, the interviewer is your collaborator, not your adversary. Effective communication can turn the interview into a productive problem-solving session, showcasing your ability to work in a team.
4. Ignoring Edge Cases and Input Validation
A common pitfall in coding interviews is focusing only on the happy path and neglecting edge cases or input validation. This oversight can lead to bugs and shows a lack of attention to detail.
Why it’s a problem:
- Demonstrates incomplete problem-solving
- Can lead to runtime errors or incorrect results
- Misses opportunities to show thoroughness and foresight
How to avoid it:
- Always consider edge cases (empty input, null values, extreme values)
- Implement input validation where appropriate
- Discuss potential edge cases with the interviewer
- Write test cases that cover various scenarios
Here’s an example of how to handle edge cases and input validation:
def find_maximum(arr):
if not arr:
raise ValueError("Input array cannot be empty")
max_value = float('-inf')
for num in arr:
if not isinstance(num, (int, float)):
raise TypeError(f"Invalid input type: {type(num)}")
max_value = max(max_value, num)
return max_value
# Test cases
print(find_maximum([1, 5, 3, 9, 2])) # Output: 9
print(find_maximum([-1, -5, -3])) # Output: -1
print(find_maximum([0])) # Output: 0
try:
print(find_maximum([]))
except ValueError as e:
print(f"Error: {e}")
try:
print(find_maximum([1, 2, "3"]))
except TypeError as e:
print(f"Error: {e}")
This example demonstrates proper handling of empty input and type checking, showing attention to detail and robustness in coding.
5. Neglecting Code Quality and Readability
In the pressure of an interview, it’s easy to focus solely on getting a working solution at the expense of code quality and readability. However, writing clean, well-structured code is a crucial skill that interviewers look for, especially at top tech companies.
Why it’s a problem:
- Difficult for interviewers to understand and evaluate your code
- Suggests poor coding practices and habits
- Makes it harder to debug and modify your solution
How to avoid it:
- Use meaningful variable and function names
- Write modular code with clear separation of concerns
- Add comments to explain complex logic or algorithms
- Follow consistent indentation and formatting
- Refactor your code if time allows
Here’s an example of how to improve code readability:
// Poor readability
def f(a,b):
c=[]
for i in range(len(a)):
if a[i]%2==0:c.append(a[i])
for i in range(len(b)):
if b[i]%2!=0:c.append(b[i])
return c
// Improved readability
def merge_even_odd(list1, list2):
"""
Merges even numbers from list1 and odd numbers from list2.
Args:
list1 (list): First input list
list2 (list): Second input list
Returns:
list: Merged list containing even numbers from list1 and odd numbers from list2
"""
result = []
for num in list1:
if num % 2 == 0:
result.append(num)
for num in list2:
if num % 2 != 0:
result.append(num)
return result
# Example usage
print(merge_even_odd([1, 2, 3, 4], [5, 6, 7, 8])) # Output: [2, 4, 5, 7]
The improved version is much easier to read, understand, and maintain, showcasing better coding practices.
6. Failing to Test Your Code
A critical mistake many candidates make is not testing their code thoroughly before declaring it complete. This can lead to overlooking bugs or edge cases that the interviewer may catch, potentially costing you the job opportunity.
Why it’s a problem:
- Leaves room for undetected errors in your solution
- Shows lack of attention to detail and quality assurance
- Misses an opportunity to demonstrate debugging skills
How to avoid it:
- Always test your code with multiple inputs, including edge cases
- Use print statements or debuggers to verify intermediate results
- Walk through your code step-by-step to ensure correctness
- If time allows, write unit tests for your functions
Here’s an example of how to incorporate testing into your coding interview process:
def is_palindrome(s):
"""
Checks if a given string is a palindrome.
Args:
s (str): Input string
Returns:
bool: True if the string is a palindrome, False otherwise
"""
# Remove non-alphanumeric characters and convert to lowercase
cleaned = ''.join(c.lower() for c in s if c.isalnum())
return cleaned == cleaned[::-1]
# Test cases
test_cases = [
("A man, a plan, a canal: Panama", True),
("race a car", False),
("", True),
("Madam, I'm Adam", True),
("12321", True),
("OpenAI", False)
]
for input_string, expected_output in test_cases:
result = is_palindrome(input_string)
print(f"Input: '{input_string}'")
print(f"Expected: {expected_output}, Got: {result}")
print(f"Test {'passed' if result == expected_output else 'failed'}")
print("---")
By including comprehensive test cases, you demonstrate thoroughness and confidence in your solution.
7. Not Asking for Help or Clarification
Many candidates hesitate to ask for help or clarification during coding interviews, fearing it might make them appear less competent. However, asking thoughtful questions can actually demonstrate your problem-solving approach and communication skills.
Why it’s a problem:
- Can lead to misunderstanding the problem or requirements
- Misses opportunities for valuable hints or guidance
- Shows poor communication skills in a team setting
How to avoid it:
- Don’t hesitate to ask for clarification on problem statements or constraints
- If you’re stuck, explain your thought process and ask for hints
- Engage the interviewer in discussion about trade-offs or alternative approaches
- Be open about any assumptions you’re making
Remember, the interview is a collaborative process. Asking questions shows that you’re engaged and thinking critically about the problem.
8. Giving Up Too Easily
Coding interviews often involve challenging problems that may not have an immediate solution. Some candidates give up too quickly when faced with difficulty, missing the opportunity to showcase their problem-solving skills and perseverance.
Why it’s a problem:
- Demonstrates lack of resilience and problem-solving skills
- Misses opportunities to learn and improve during the interview
- Fails to show your full potential to the interviewer
How to avoid it:
- Stay calm and maintain a positive attitude when faced with challenges
- Break down complex problems into smaller, manageable steps
- Start with a brute force solution and then work on optimizing it
- Think out loud and discuss your approach, even if you’re not sure it’s correct
- Use examples or diagrams to visualize the problem and potential solutions
Remember, interviewers are often more interested in your problem-solving process than in seeing a perfect solution immediately.
9. Neglecting to Review and Optimize Your Solution
After implementing a working solution, many candidates consider the task complete without taking the time to review and optimize their code. This misses a crucial opportunity to demonstrate your ability to critically analyze and improve your work.
Why it’s a problem:
- Overlooks potential optimizations or improvements
- Misses chances to showcase advanced problem-solving skills
- Fails to demonstrate attention to detail and code quality
How to avoid it:
- Always review your code after implementing a solution
- Look for opportunities to optimize time or space complexity
- Consider alternative data structures or algorithms that might be more efficient
- Refactor your code to improve readability and maintainability
- Discuss potential improvements with the interviewer, even if you don’t have time to implement them
Here’s an example of how you might optimize a solution:
// Initial solution: O(n^2) time complexity
def find_duplicate(arr):
for i in range(len(arr)):
for j in range(i+1, len(arr)):
if arr[i] == arr[j]:
return arr[i]
return None
// Optimized solution: O(n) time complexity, O(n) space complexity
def find_duplicate_optimized(arr):
seen = set()
for num in arr:
if num in seen:
return num
seen.add(num)
return None
// Further optimized solution: O(n) time complexity, O(1) space complexity
def find_duplicate_cycle_detection(arr):
slow = fast = arr[0]
while True:
slow = arr[slow]
fast = arr[arr[fast]]
if slow == fast:
break
slow = arr[0]
while slow != fast:
slow = arr[slow]
fast = arr[fast]
return slow
# Test the solutions
test_arr = [3, 1, 3, 4, 2]
print(find_duplicate(test_arr)) # Output: 3
print(find_duplicate_optimized(test_arr)) # Output: 3
print(find_duplicate_cycle_detection(test_arr)) # Output: 3
By presenting multiple solutions with increasing levels of optimization, you demonstrate your ability to think critically about efficiency and showcase your knowledge of advanced algorithms.
10. Forgetting to Ask Questions at the End
Many candidates focus solely on solving the coding problem and forget that the interview is also an opportunity to learn about the company and the role. Not asking questions at the end of the interview can make you appear disinterested or unprepared.
Why it’s a problem:
- Misses an opportunity to show genuine interest in the company and role
- Fails to gather important information for your own decision-making
- Can leave a less engaging final impression on the interviewer
How to avoid it:
- Prepare a list of thoughtful questions about the company, team, and role
- Ask about the interviewer’s experience and what they enjoy about working there
- Inquire about the company’s engineering culture and development practices
- Ask about potential challenges or exciting projects in the role
- Use this time to clarify any doubts about the interview process or next steps
Some example questions you might ask:
- “What does a typical day look like for a software engineer in your team?”
- “How does the company support continuous learning and professional development?”
- “Can you tell me about a recent project that you found particularly challenging or exciting?”
- “How does the team balance between shipping features quickly and maintaining code quality?”
- “What opportunities are there for mentorship or collaboration with senior engineers?”
Remember, asking insightful questions not only helps you gather valuable information but also demonstrates your enthusiasm and thoughtfulness to the interviewer.
Conclusion
Avoiding these common pitfalls can significantly improve your performance in coding interviews, especially when aiming for positions at top tech companies. By focusing on thorough problem analysis, efficient coding practices, effective communication, and continuous improvement, you’ll be better prepared to showcase your skills and land your dream job.
Remember that practice is key to mastering these skills. Platforms like AlgoCademy offer interactive coding tutorials and resources specifically designed to help you prepare for technical interviews at major tech companies. By leveraging such tools and consistently working on your problem-solving skills, you’ll be well-equipped to tackle even the most challenging coding interviews with confidence.
As you continue your journey in software development and interview preparation, keep these tips in mind and always strive for improvement. With dedication and the right approach, you’ll be well on your way to succeeding in your coding interviews and advancing your career in the tech industry.