Amazon Technical Interview Prep: A Comprehensive Guide
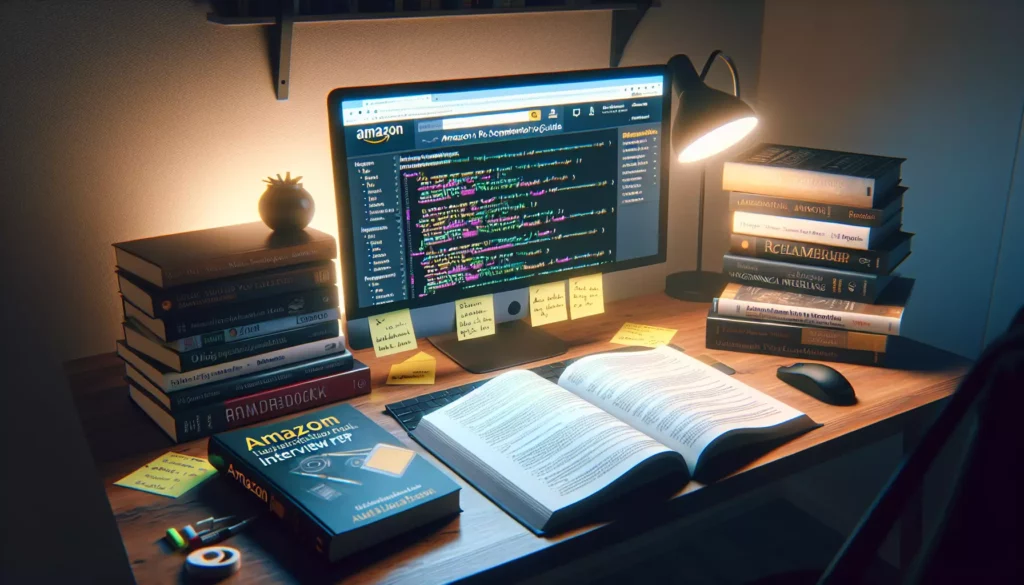
Preparing for a technical interview at Amazon can be a daunting task, but with the right approach and resources, you can significantly increase your chances of success. In this comprehensive guide, we’ll walk you through everything you need to know to ace your Amazon technical interview, from understanding the interview process to mastering key coding concepts and problem-solving strategies.
Table of Contents
- Understanding the Amazon Interview Process
- Key Topics to Master
- Coding Practice and Resources
- System Design and Scalability
- Behavioral Questions and Leadership Principles
- Mock Interviews and Peer Practice
- Interview Day Tips
- Post-Interview Follow-up
1. Understanding the Amazon Interview Process
Before diving into preparation, it’s crucial to understand what to expect during the Amazon technical interview process. Typically, it consists of several stages:
- Initial Phone Screen: A brief conversation with a recruiter to assess your background and interest.
- Online Assessment: A combination of coding challenges and work-style assessment.
- Technical Phone Interview: A remote coding interview focusing on problem-solving and algorithms.
- On-site Interviews: A series of 4-5 interviews, including coding, system design, and behavioral questions.
Each stage is designed to evaluate different aspects of your skills and potential fit within Amazon’s culture. Understanding this structure will help you tailor your preparation effectively.
2. Key Topics to Master
To excel in Amazon’s technical interviews, you should have a strong grasp of the following topics:
Data Structures
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
Algorithms
- Sorting and Searching
- Recursion and Dynamic Programming
- Breadth-First Search (BFS) and Depth-First Search (DFS)
- Binary Search
- Greedy Algorithms
Problem-Solving Techniques
- Time and Space Complexity Analysis
- Optimization Techniques
- Edge Case Handling
- Testing and Debugging
Let’s dive deeper into one of these topics with an example:
Example: Implementing a Binary Search Tree (BST)
A Binary Search Tree is a fundamental data structure that’s often featured in technical interviews. Here’s a basic implementation in Python:
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = Node(value)
return
current = self.root
while True:
if value < current.value:
if current.left is None:
current.left = Node(value)
break
current = current.left
else:
if current.right is None:
current.right = Node(value)
break
current = current.right
def search(self, value):
current = self.root
while current:
if value == current.value:
return True
elif value < current.value:
current = current.left
else:
current = current.right
return False
Understanding how to implement and manipulate data structures like BSTs is crucial for succeeding in Amazon’s technical interviews.
3. Coding Practice and Resources
Consistent practice is key to improving your coding skills and problem-solving abilities. Here are some recommended resources and strategies:
Online Coding Platforms
- LeetCode: Offers a wide range of coding problems, including a section specifically for Amazon interview questions.
- HackerRank: Provides coding challenges and competitions to sharpen your skills.
- AlgoExpert: Offers curated lists of coding problems with video explanations.
Books
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Elements of Programming Interviews” by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash
Practice Strategy
- Start with easy problems and gradually increase difficulty.
- Time yourself to simulate interview conditions.
- Focus on understanding the problem-solving approach rather than memorizing solutions.
- Review and learn from others’ solutions after solving a problem.
- Regularly revisit problems to reinforce your understanding.
Example: Two Sum Problem
Let’s look at a common coding problem you might encounter during an Amazon interview:
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
# Test the function
print(two_sum([2, 7, 11, 15], 9)) # Output: [0, 1]
This problem demonstrates the use of a hash table to optimize the solution, a technique frequently used in coding interviews.
4. System Design and Scalability
For more senior positions, Amazon places a strong emphasis on system design questions. These assess your ability to design large-scale distributed systems. Key areas to focus on include:
- Scalability and Performance
- Load Balancing
- Caching
- Database Sharding
- Microservices Architecture
- CAP Theorem
When approaching system design questions, follow this general framework:
- Clarify requirements and constraints
- Outline high-level design
- Deep dive into core components
- Identify and address bottlenecks
- Discuss trade-offs and potential improvements
Example: Designing a URL Shortener
Here’s a high-level overview of how you might approach designing a URL shortener system:
- Requirements:
- Shorten long URLs
- Redirect users to original URL when accessing shortened link
- High availability and low latency
- High-Level Design:
- API Gateway for handling requests
- Application servers for URL shortening and redirection logic
- Database to store URL mappings
- Caching layer for frequently accessed URLs
- Deep Dive:
- URL shortening algorithm (e.g., base62 encoding of incremental IDs)
- Database schema and indexing
- Caching strategy (e.g., LRU cache)
- Scalability Considerations:
- Database sharding for horizontal scaling
- Read replicas for handling high read traffic
- CDN integration for global low-latency access
Being able to articulate such designs clearly and discuss trade-offs will greatly impress your interviewers.
5. Behavioral Questions and Leadership Principles
Amazon places a strong emphasis on cultural fit and assesses candidates based on their Leadership Principles. Be prepared to answer behavioral questions that demonstrate your alignment with these principles:
- Customer Obsession
- Ownership
- Invent and Simplify
- Are Right, A Lot
- Learn and Be Curious
- Hire and Develop the Best
- Insist on the Highest Standards
- Think Big
- Bias for Action
- Frugality
- Earn Trust
- Dive Deep
- Have Backbone; Disagree and Commit
- Deliver Results
Use the STAR method (Situation, Task, Action, Result) to structure your responses to behavioral questions. Here’s an example:
Example: Demonstrating “Ownership”
Question: “Tell me about a time when you took on a task that was beyond your job responsibilities.”
Answer:
- Situation: In my previous role, our team was facing a critical deadline for a major product launch.
- Task: While my primary responsibility was frontend development, I noticed our backend team was overwhelmed and struggling to complete a crucial API on time.
- Action: I volunteered to assist with the backend development, spending extra hours learning the necessary skills and collaborating closely with the backend team.
- Result: We successfully completed the API on schedule, ensuring a smooth product launch. This experience not only helped the team but also expanded my skill set, making me a more versatile developer.
Preparing several such examples for each Leadership Principle will help you confidently address behavioral questions during your interview.
6. Mock Interviews and Peer Practice
One of the most effective ways to prepare for Amazon technical interviews is through mock interviews and peer practice. These simulate real interview conditions and help you:
- Improve your ability to think out loud and communicate your problem-solving process
- Get comfortable with the pressure of being observed while coding
- Receive feedback on your performance and identify areas for improvement
- Practice time management during problem-solving
Resources for Mock Interviews:
- Pramp: A free platform that pairs you with other candidates for mock interviews.
- interviewing.io: Offers anonymous mock interviews with experienced engineers.
- AlgoCademy: Provides AI-powered mock interviews and personalized feedback.
Tips for Effective Peer Practice:
- Find a study partner or group with similar goals.
- Alternate between interviewer and interviewee roles.
- Use a timer to simulate real interview conditions.
- Provide constructive feedback to each other after each session.
- Discuss different approaches to problems and learn from each other’s perspectives.
Example: Mock Interview Scenario
Here’s a sample dialogue demonstrating how you might approach a problem during a mock interview:
Interviewer: “Given a binary tree, write a function to check if it’s a valid binary search tree (BST).”
Candidate: “Thank you for the question. Before I start coding, let me make sure I understand the problem correctly. A valid BST is a binary tree where for each node, all values in its left subtree are less than the node’s value, and all values in its right subtree are greater than the node’s value. Is this correct?”
Interviewer: “That’s correct. Please proceed.”
Candidate: “Great. Let me think about the approach for a moment… We could use a recursive solution here. We’ll need to keep track of the valid range for each node as we traverse the tree. Let me write out the function signature and then explain my approach further.”
def is_valid_bst(root):
def validate(node, low=float('-inf'), high=float('inf')):
if not node:
return True
if node.val <= low or node.val >= high:
return False
return validate(node.left, low, node.val) and validate(node.right, node.val, high)
return validate(root)
Candidate: “Now, let me explain this solution. We’re using a helper function ‘validate’ that takes three parameters: the current node, the lower bound, and the upper bound for the valid range of values for this node. We start with negative infinity as the lower bound and positive infinity as the upper bound for the root node.
As we recursively traverse the tree, we update these bounds. For the left child, the upper bound becomes the parent’s value, and for the right child, the lower bound becomes the parent’s value. If at any point we find a node that’s outside its valid range, we return False. If we successfully traverse the entire tree without finding any invalid nodes, we return True.
The time complexity of this solution is O(n) where n is the number of nodes in the tree, as we visit each node once. The space complexity is O(h) where h is the height of the tree, due to the recursion stack.
Would you like me to walk through an example or discuss any part of the solution in more detail?”
This example demonstrates clear communication, problem-solving approach, and code implementation – all crucial elements in a successful interview.
7. Interview Day Tips
When the big day arrives, keep these tips in mind to perform at your best:
- Be well-rested: Get a good night’s sleep before the interview.
- Arrive early: For on-site interviews, arrive at least 15 minutes early. For virtual interviews, test your setup well in advance.
- Bring necessary items: For on-site interviews, bring a government-issued ID, copies of your resume, and a notebook.
- Dress appropriately: While Amazon has a casual dress code, it’s best to err on the side of business casual for interviews.
- Stay calm and focused: Take deep breaths and remember that it’s okay to ask for clarification or take a moment to think.
- Communicate clearly: Explain your thought process as you work through problems. This is often as important as the solution itself.
- Be yourself: Show your personality and enthusiasm for technology and problem-solving.
Virtual Interview Tips
If your interview is conducted remotely, additional considerations include:
- Ensure a stable internet connection
- Choose a quiet, well-lit location
- Test your camera and microphone beforehand
- Have a backup plan (e.g., phone number) in case of technical issues
- Keep a glass of water nearby
- Close unnecessary applications to avoid distractions
8. Post-Interview Follow-up
After your interview, take these steps to leave a positive final impression:
- Send a thank-you email: Within 24 hours, send a brief email to your interviewer(s) or recruiter, expressing gratitude for their time and reiterating your interest in the position.
- Reflect on your performance: Make notes about questions you found challenging or areas where you think you could improve. This will be valuable for future interviews, regardless of the outcome.
- Be patient: The hiring process at large companies like Amazon can take time. If you haven’t heard back within the timeframe provided, it’s appropriate to follow up with your recruiter for an update.
- Keep learning: Continue to practice and improve your skills while waiting for a response. This keeps you sharp for potential follow-up interviews or future opportunities.
Example Thank-You Email
Subject: Thank you for the interview - [Your Name]
Dear [Interviewer's Name],
I wanted to thank you for taking the time to meet with me yesterday regarding the Software Development Engineer position at Amazon. I enjoyed our conversation about [specific topic discussed] and was excited to learn more about the challenges your team is tackling.
Our discussion reinforced my enthusiasm for the role and my desire to contribute to Amazon's mission of [relevant company goal]. I'm particularly interested in the opportunity to [specific aspect of the job you discussed].
If you need any additional information from me, please don't hesitate to reach out. I look forward to hearing about the next steps in the process.
Thank you again for your time and consideration.
Best regards,
[Your Name]
Remember, this email should be concise, personalized, and error-free.
Conclusion
Preparing for an Amazon technical interview requires dedication, consistent practice, and a strategic approach. By focusing on core computer science concepts, honing your problem-solving skills, understanding Amazon’s culture and leadership principles, and practicing mock interviews, you’ll be well-equipped to showcase your abilities and land your dream job at Amazon.
Remember that the interview process is not just about demonstrating your technical skills, but also about showing your passion for technology, your ability to learn and adapt, and your potential to contribute to Amazon’s innovative culture. Stay confident, be yourself, and let your skills and enthusiasm shine through.
Good luck with your Amazon technical interview! With thorough preparation and the right mindset, you’re setting yourself up for success in this exciting opportunity.