Algorithm Animation: Revolutionizing Visual Learning Tools for Coding Education
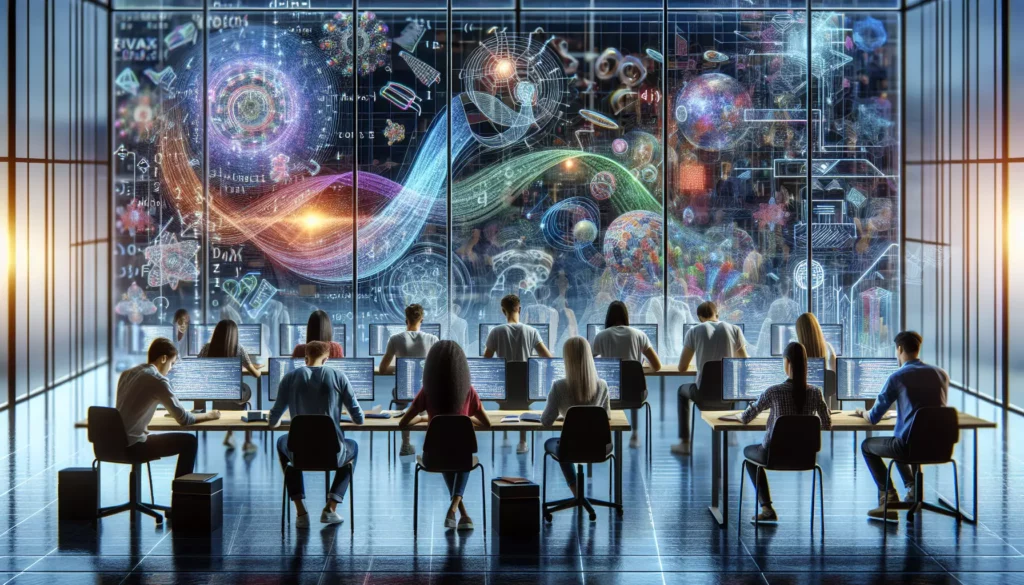
In the ever-evolving landscape of coding education, algorithm animation has emerged as a powerful visual learning tool that is transforming the way students and aspiring programmers grasp complex algorithmic concepts. As the demand for skilled programmers continues to grow, particularly in preparation for technical interviews at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), innovative platforms like AlgoCademy are leveraging algorithm animation to enhance the learning experience and accelerate skill development.
This comprehensive guide will explore the world of algorithm animation, its impact on coding education, and how it’s being utilized to create more effective and engaging learning experiences. We’ll delve into the benefits, implementation techniques, and real-world applications of this cutting-edge visual learning tool.
Understanding Algorithm Animation
Algorithm animation is a dynamic visualization technique that brings algorithms to life through animated graphics and interactive displays. It allows learners to observe the step-by-step execution of an algorithm, making abstract concepts more tangible and easier to comprehend. By presenting algorithms in a visual format, learners can better understand the flow of data, the decision-making process, and the overall logic behind various algorithmic solutions.
Key Components of Algorithm Animation
- Visual Representation: Algorithms are depicted using graphical elements such as shapes, colors, and movements.
- Step-by-Step Execution: The animation progresses through each stage of the algorithm, allowing learners to follow the process in detail.
- Interactive Controls: Users can often pause, rewind, or fast-forward the animation to focus on specific parts of the algorithm.
- Data Visualization: Input data and intermediate results are visually represented to show how information is processed throughout the algorithm.
The Impact of Algorithm Animation on Coding Education
The integration of algorithm animation into coding education has brought about significant improvements in learning outcomes and student engagement. Here are some of the key benefits:
1. Enhanced Understanding of Complex Concepts
Algorithm animation breaks down complex algorithms into digestible visual components, making it easier for learners to grasp intricate concepts. This visual approach is particularly beneficial for understanding:
- Sorting algorithms (e.g., bubble sort, quicksort, merge sort)
- Graph algorithms (e.g., breadth-first search, depth-first search)
- Tree traversal techniques
- Dynamic programming solutions
2. Improved Retention and Recall
Visual learning has been shown to improve information retention and recall. By associating algorithmic concepts with visual representations, learners are more likely to remember and apply these concepts in future problem-solving scenarios.
3. Increased Engagement and Motivation
Interactive algorithm animations make the learning process more engaging and enjoyable. This increased engagement often translates to higher motivation levels among students, encouraging them to explore more advanced topics and tackle challenging problems.
4. Faster Debugging and Problem-Solving
By visualizing the step-by-step execution of an algorithm, learners can more easily identify errors in their code and understand why certain approaches may be inefficient. This skill is crucial for debugging and optimizing code in real-world scenarios.
5. Bridging the Gap Between Theory and Practice
Algorithm animation helps bridge the gap between theoretical knowledge and practical application. By seeing algorithms in action, learners can better understand how to implement these concepts in their own code.
Implementing Algorithm Animation in Coding Education Platforms
To effectively implement algorithm animation in coding education platforms like AlgoCademy, several key considerations and techniques should be taken into account:
1. Choosing the Right Visualization Library
Selecting an appropriate visualization library is crucial for creating effective algorithm animations. Some popular options include:
- D3.js: A powerful JavaScript library for creating dynamic, interactive data visualizations in web browsers.
- p5.js: A JavaScript library that makes it easy to create graphics and interactive experiences, ideal for educational purposes.
- Three.js: A cross-browser JavaScript library used to create and display animated 3D computer graphics in a web browser.
2. Designing Clear and Intuitive Visualizations
When creating algorithm animations, it’s essential to design visualizations that are both clear and intuitive. Consider the following best practices:
- Use consistent color coding to represent different elements or states within the algorithm.
- Implement smooth transitions between steps to help learners follow the flow of the algorithm.
- Provide clear labels and annotations to explain each step of the process.
- Ensure that the visualization is scalable and responsive across different devices and screen sizes.
3. Incorporating Interactive Elements
To maximize the effectiveness of algorithm animations, incorporate interactive elements that allow learners to engage with the visualization actively. Some ideas include:
- Playback controls (play, pause, step forward, step backward)
- Speed adjustment options to slow down or speed up the animation
- Input fields for users to modify the initial data or parameters
- Clickable elements that provide additional information or explanations
4. Syncing Animation with Code Execution
To create a seamless learning experience, it’s important to synchronize the algorithm animation with the actual code execution. This can be achieved by:
- Highlighting the relevant code lines as the animation progresses
- Updating variable values in real-time alongside the visual representation
- Providing a split-screen view that shows both the code and the animation simultaneously
5. Optimizing Performance
Ensure that the algorithm animations run smoothly across different devices and browsers by optimizing performance:
- Use efficient rendering techniques to minimize CPU and GPU usage
- Implement lazy loading for complex animations to reduce initial load times
- Consider using WebGL for hardware-accelerated graphics rendering when appropriate
Real-World Applications of Algorithm Animation in Coding Education
Algorithm animation has found numerous applications in coding education, particularly in platforms like AlgoCademy that focus on preparing learners for technical interviews and advancing their programming skills. Here are some practical use cases:
1. Sorting Algorithm Visualizations
Sorting algorithms are a fundamental concept in computer science and are frequently tested in technical interviews. Algorithm animations can effectively illustrate the differences between various sorting techniques, such as:
- Bubble Sort
- Selection Sort
- Insertion Sort
- Merge Sort
- Quick Sort
- Heap Sort
For example, a bubble sort animation might look like this:
<!-- Bubble Sort Animation (Pseudocode) -->
function bubbleSortAnimation(array) {
for (i = 0; i < array.length - 1; i++) {
for (j = 0; j < array.length - i - 1; j++) {
if (array[j] > array[j + 1]) {
// Swap elements
swap(array[j], array[j + 1]);
// Animate the swap
animateSwap(j, j + 1);
}
}
}
}
2. Graph Algorithm Visualizations
Graph algorithms are crucial for solving complex problems and are often featured in technical interviews. Algorithm animations can help learners understand the traversal and search processes in graph structures. Some common graph algorithms that benefit from visualization include:
- Breadth-First Search (BFS)
- Depth-First Search (DFS)
- Dijkstra’s Shortest Path Algorithm
- Kruskal’s Minimum Spanning Tree Algorithm
- Topological Sorting
Here’s a simple example of how a Depth-First Search animation might be implemented:
<!-- DFS Animation (Pseudocode) -->
function dfsAnimation(graph, startNode) {
let visited = new Set();
function dfs(node) {
visited.add(node);
animateVisit(node);
for (let neighbor of graph[node]) {
if (!visited.has(neighbor)) {
animateEdge(node, neighbor);
dfs(neighbor);
}
}
}
dfs(startNode);
}
3. Dynamic Programming Visualizations
Dynamic programming is an advanced technique that often challenges learners. Algorithm animations can break down the process of building optimal solutions from subproblems, making it easier to understand concepts like:
- Fibonacci Sequence
- Longest Common Subsequence
- Knapsack Problem
- Matrix Chain Multiplication
An animation for the Fibonacci sequence calculation might look like this:
<!-- Fibonacci Animation (Pseudocode) -->
function fibonacciAnimation(n) {
let dp = new Array(n + 1);
dp[0] = 0;
dp[1] = 1;
for (let i = 2; i <= n; i++) {
dp[i] = dp[i-1] + dp[i-2];
animateCalculation(i, dp[i-1], dp[i-2], dp[i]);
}
return dp[n];
}
4. Tree Traversal Visualizations
Tree data structures and their traversal algorithms are fundamental in computer science. Algorithm animations can effectively demonstrate different traversal techniques, such as:
- In-order Traversal
- Pre-order Traversal
- Post-order Traversal
- Level-order Traversal
Here’s an example of how an in-order traversal animation might be implemented:
<!-- In-order Traversal Animation (Pseudocode) -->
function inOrderTraversalAnimation(root) {
if (root === null) return;
inOrderTraversalAnimation(root.left);
animateVisit(root);
inOrderTraversalAnimation(root.right);
}
Challenges and Considerations in Implementing Algorithm Animation
While algorithm animation offers numerous benefits for coding education, there are several challenges and considerations to keep in mind when implementing this visual learning tool:
1. Balancing Simplicity and Accuracy
Creating animations that are both simple enough for beginners to understand and accurate enough to represent the algorithm correctly can be challenging. It’s important to strike a balance between simplifying complex concepts and maintaining the integrity of the algorithm’s logic.
2. Scalability for Large Data Sets
Some algorithms may behave differently or become less efficient with large data sets. Designing animations that can effectively demonstrate algorithm performance across various input sizes while maintaining smooth performance can be technically challenging.
3. Accessibility Considerations
Ensuring that algorithm animations are accessible to all learners, including those with visual impairments or other disabilities, requires careful consideration. Providing alternative text descriptions, keyboard navigation options, and other accessibility features is crucial for inclusive education.
4. Cross-Platform Compatibility
Creating animations that work consistently across different devices, browsers, and operating systems can be challenging. It’s important to test and optimize animations for a wide range of platforms to ensure a smooth learning experience for all users.
5. Performance Optimization
Complex animations can be resource-intensive, potentially leading to slow performance or high battery consumption on mobile devices. Optimizing animations for performance while maintaining visual quality is an ongoing challenge.
Future Trends in Algorithm Animation for Coding Education
As technology continues to advance, we can expect to see several exciting developments in the field of algorithm animation for coding education:
1. Virtual and Augmented Reality Integration
The integration of VR and AR technologies could revolutionize algorithm animation by allowing learners to interact with three-dimensional representations of algorithms in immersive environments.
2. Machine Learning-Powered Adaptive Animations
AI and machine learning techniques could be used to create adaptive animations that adjust their complexity and pace based on the learner’s skill level and learning style.
3. Collaborative Learning Environments
Future platforms may incorporate real-time collaborative features, allowing multiple learners to interact with and manipulate algorithm animations together, fostering peer-to-peer learning and problem-solving.
4. Integration with Coding IDEs
Tighter integration between algorithm animations and popular integrated development environments (IDEs) could provide seamless visualization of code execution directly within the coding interface.
5. Natural Language Processing for Animation Generation
Advanced NLP techniques could enable the automatic generation of algorithm animations from written or spoken descriptions, making it easier for educators to create custom visualizations.
Conclusion
Algorithm animation has emerged as a powerful visual learning tool in coding education, offering numerous benefits for learners at all levels. From enhancing understanding of complex concepts to improving retention and engagement, this innovative approach is transforming the way programming skills are developed and refined.
Platforms like AlgoCademy are at the forefront of this revolution, leveraging algorithm animation to create more effective and engaging learning experiences. As the demand for skilled programmers continues to grow, particularly in preparation for technical interviews at major tech companies, the role of algorithm animation in coding education is likely to become increasingly significant.
By embracing this technology and continually refining its implementation, educators and learning platforms can provide aspiring programmers with the tools they need to visualize, understand, and master complex algorithmic concepts. As we look to the future, the continued evolution of algorithm animation promises to unlock new possibilities in coding education, preparing the next generation of developers to tackle the challenges of tomorrow’s technological landscape.