Rod Cutting Problem: A Comprehensive Guide to Dynamic Programming
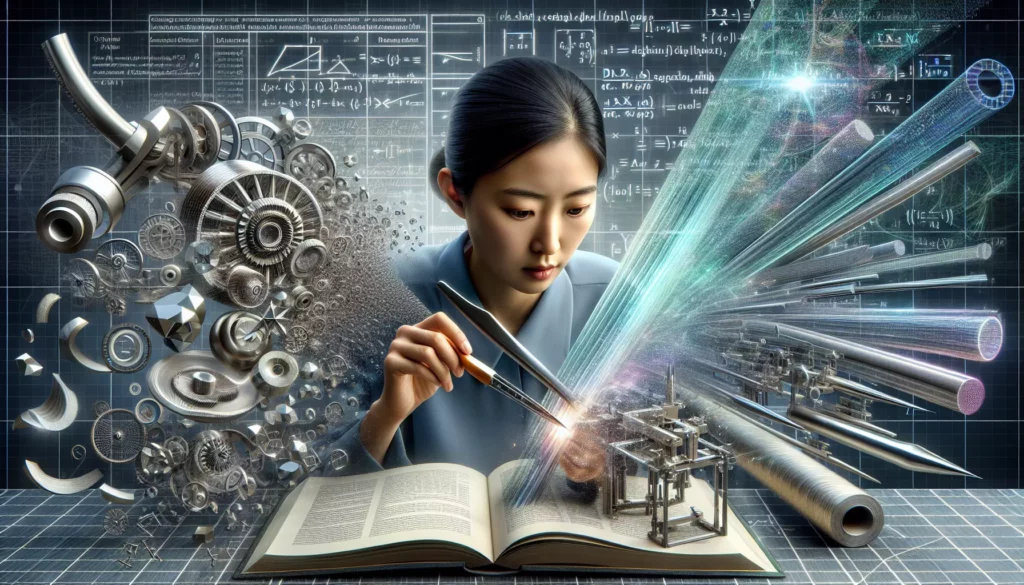
Welcome to AlgoCademy’s in-depth exploration of the Rod Cutting Problem, a classic example of dynamic programming in computer science. This problem is not only a staple in coding interviews but also a fundamental concept that sharpens your algorithmic thinking and problem-solving skills. Whether you’re a beginner looking to understand dynamic programming or an experienced coder preparing for technical interviews at major tech companies, this guide will provide you with the knowledge and practical skills to tackle the Rod Cutting Problem effectively.
Table of Contents
- Introduction to the Rod Cutting Problem
- Problem Statement and Formal Definition
- Naive Recursive Approach
- Dynamic Programming Solution
- Time and Space Complexity Analysis
- Implementation in Various Programming Languages
- Variations and Related Problems
- Interview Tips and Common Pitfalls
- Conclusion and Further Resources
1. Introduction to the Rod Cutting Problem
The Rod Cutting Problem is a classic optimization problem in computer science and operations research. It serves as an excellent introduction to dynamic programming concepts and techniques. The problem’s simplicity in statement, coupled with its depth in solution, makes it a favorite among educators and interviewers alike.
At its core, the Rod Cutting Problem asks: Given a rod of length n and a price list for rod pieces of different lengths, how should we cut the rod to maximize the total revenue? This seemingly straightforward question opens up a world of algorithmic possibilities and teaches us valuable lessons about efficient problem-solving and optimization.
2. Problem Statement and Formal Definition
Let’s formally define the Rod Cutting Problem:
- You are given a rod of length n inches.
- You are also given a price list that contains prices of all pieces of size smaller than n.
- Determine the maximum value obtainable by cutting up the rod and selling the pieces.
For example, suppose the given rod is of length 8 and the price list is as follows:
length | 1 2 3 4 5 6 7 8
price | 1 5 8 9 10 17 17 20
The maximum obtainable value is 22 (by cutting in two pieces of lengths 2 and 6)
Mathematically, we can express this problem as:
maximize Σ(i=1 to n) (p[i] * x[i])
subject to Σ(i=1 to n) (i * x[i]) <= n
and x[i] >= 0 for all i
Where:
- n is the length of the rod
- p[i] is the price of a rod of length i
- x[i] is the number of pieces of length i to cut
3. Naive Recursive Approach
Before diving into the dynamic programming solution, let’s consider a naive recursive approach to solve this problem. This approach will help us understand the inherent complexity and the need for a more efficient solution.
The basic idea is to consider all possible ways to cut the rod and choose the one that gives maximum revenue. We can express this recursively as follows:
cutRod(price, n) = max(price[i] + cutRod(price, n-i-1)) for all i in {0, 1 .. n-1}
Here’s a simple implementation in Python:
def cut_rod_naive(price, n):
if n <= 0:
return 0
max_val = float('-inf')
for i in range(n):
max_val = max(max_val, price[i] + cut_rod_naive(price, n-i-1))
return max_val
# Example usage
price = [1, 5, 8, 9, 10, 17, 17, 20]
n = len(price)
print(f"Maximum obtainable value is {cut_rod_naive(price, n)}")
While this approach correctly solves the problem, it has a time complexity of O(2^n), making it impractical for larger inputs. This is where dynamic programming comes to our rescue.
4. Dynamic Programming Solution
Dynamic programming is an algorithmic paradigm that solves a given complex problem by breaking it into subproblems and stores the results of subproblems to avoid computing the same results again. It is mainly an optimization over plain recursion.
For the Rod Cutting Problem, we can apply dynamic programming in two ways: Top-Down (Memoization) and Bottom-Up (Tabulation).
Top-Down Approach (Memoization)
In the top-down approach, we start from the main problem and break it down into subproblems. We use a cache (usually an array) to store the results of subproblems. This approach is also known as memoization.
Here’s the Python implementation of the top-down approach:
def cut_rod_top_down(price, n):
memo = [-1] * (n + 1)
def cut_rod_recursive(price, n):
if n <= 0:
return 0
if memo[n] != -1:
return memo[n]
max_val = float('-inf')
for i in range(n):
max_val = max(max_val, price[i] + cut_rod_recursive(price, n-i-1))
memo[n] = max_val
return max_val
return cut_rod_recursive(price, n)
# Example usage
price = [1, 5, 8, 9, 10, 17, 17, 20]
n = len(price)
print(f"Maximum obtainable value is {cut_rod_top_down(price, n)}")
Bottom-Up Approach (Tabulation)
The bottom-up approach starts with the smallest subproblems and works its way up to the main problem. This approach is typically implemented using iteration rather than recursion.
Here’s the Python implementation of the bottom-up approach:
def cut_rod_bottom_up(price, n):
val = [0 for x in range(n+1)]
val[0] = 0
for i in range(1, n+1):
max_val = float('-inf')
for j in range(i):
max_val = max(max_val, price[j] + val[i-j-1])
val[i] = max_val
return val[n]
# Example usage
price = [1, 5, 8, 9, 10, 17, 17, 20]
n = len(price)
print(f"Maximum obtainable value is {cut_rod_bottom_up(price, n)}")
5. Time and Space Complexity Analysis
Let’s analyze the time and space complexity of our dynamic programming solutions:
Time Complexity
- Naive Recursive Approach: O(2^n)
- Top-Down (Memoization): O(n^2)
- Bottom-Up (Tabulation): O(n^2)
Both dynamic programming approaches significantly improve upon the naive recursive solution, reducing the time complexity from exponential to polynomial.
Space Complexity
- Naive Recursive Approach: O(n) (due to the recursion stack)
- Top-Down (Memoization): O(n)
- Bottom-Up (Tabulation): O(n)
The space complexity remains linear for all approaches, but the dynamic programming solutions use this space more efficiently by storing and reusing computed results.
6. Implementation in Various Programming Languages
To help you prepare for coding interviews across different platforms, let’s implement the Rod Cutting Problem solution in a few popular programming languages:
Java Implementation
public class RodCutting {
public static int cutRod(int price[], int n) {
int val[] = new int[n+1];
val[0] = 0;
for (int i = 1; i <= n; i++) {
int max_val = Integer.MIN_VALUE;
for (int j = 0; j < i; j++)
max_val = Math.max(max_val, price[j] + val[i-j-1]);
val[i] = max_val;
}
return val[n];
}
public static void main(String args[]) {
int arr[] = new int[] {1, 5, 8, 9, 10, 17, 17, 20};
int size = arr.length;
System.out.println("Maximum Obtainable Value is " + cutRod(arr, size));
}
}
C++ Implementation
#include <iostream>
#include <climits>
using namespace std;
int cutRod(int price[], int n) {
int val[n+1];
val[0] = 0;
for (int i = 1; i <= n; i++) {
int max_val = INT_MIN;
for (int j = 0; j < i; j++)
max_val = max(max_val, price[j] + val[i-j-1]);
val[i] = max_val;
}
return val[n];
}
int main() {
int arr[] = {1, 5, 8, 9, 10, 17, 17, 20};
int size = sizeof(arr)/sizeof(arr[0]);
cout << "Maximum Obtainable Value is " << cutRod(arr, size) << endl;
return 0;
}
7. Variations and Related Problems
The Rod Cutting Problem is part of a larger family of dynamic programming problems. Understanding its solution can help you tackle similar problems. Here are some variations and related problems:
- Coin Change Problem: Given a set of coin denominations and a target amount, find the minimum number of coins needed to make up that amount.
- Knapsack Problem: Given a set of items, each with a weight and a value, determine the number of each item to include in a collection so that the total weight is less than or equal to a given limit and the total value is as large as possible.
- Longest Common Subsequence: Given two sequences, find the length of longest subsequence present in both of them.
- Matrix Chain Multiplication: Given a sequence of matrices, find the most efficient way to multiply these matrices together.
Each of these problems can be solved using similar dynamic programming techniques, and mastering the Rod Cutting Problem will give you a solid foundation for tackling these and many other optimization problems.
8. Interview Tips and Common Pitfalls
When facing the Rod Cutting Problem or similar dynamic programming questions in an interview, keep these tips in mind:
- Understand the problem thoroughly: Make sure you grasp all aspects of the problem before diving into the solution. Ask clarifying questions if needed.
- Start with a simple approach: Begin with the naive recursive solution to demonstrate your problem-solving process.
- Identify overlapping subproblems: This is key to recognizing that dynamic programming can be applied.
- Choose the right DP approach: Decide whether top-down or bottom-up is more suitable for the given problem and explain your reasoning.
- Optimize space if possible: After implementing a working solution, consider if you can optimize the space complexity.
- Analyze time and space complexity: Be prepared to discuss the complexity of your solution in detail.
- Consider edge cases: Don’t forget to handle edge cases like an empty input or negative values.
Common pitfalls to avoid:
- Jumping to the DP solution without explaining your thought process
- Neglecting to handle base cases in recursive solutions
- Confusing top-down and bottom-up approaches
- Forgetting to initialize your DP table or memoization array
- Not considering the space complexity of your solution
9. Conclusion and Further Resources
The Rod Cutting Problem is a fantastic introduction to dynamic programming and serves as a stepping stone to more complex optimization problems. By mastering this problem, you’ve gained valuable insights into problem decomposition, subproblem identification, and efficient algorithm design.
To further enhance your skills in dynamic programming and prepare for coding interviews, consider exploring these resources:
- AlgoCademy’s interactive coding tutorials on dynamic programming
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein (CLRS) – Chapter on Dynamic Programming
- LeetCode’s Dynamic Programming problem set
- GeeksforGeeks’ Dynamic Programming articles and problems
Remember, the key to mastering dynamic programming is practice. Try to solve various problems, implement solutions in different programming languages, and always strive to optimize your code. With consistent effort and the right resources, you’ll be well-prepared to tackle even the most challenging dynamic programming problems in your coding interviews.
Happy coding, and may your algorithms always find the optimal solution!