Mastering Bit Manipulation: Essential Skills for Coding Interviews
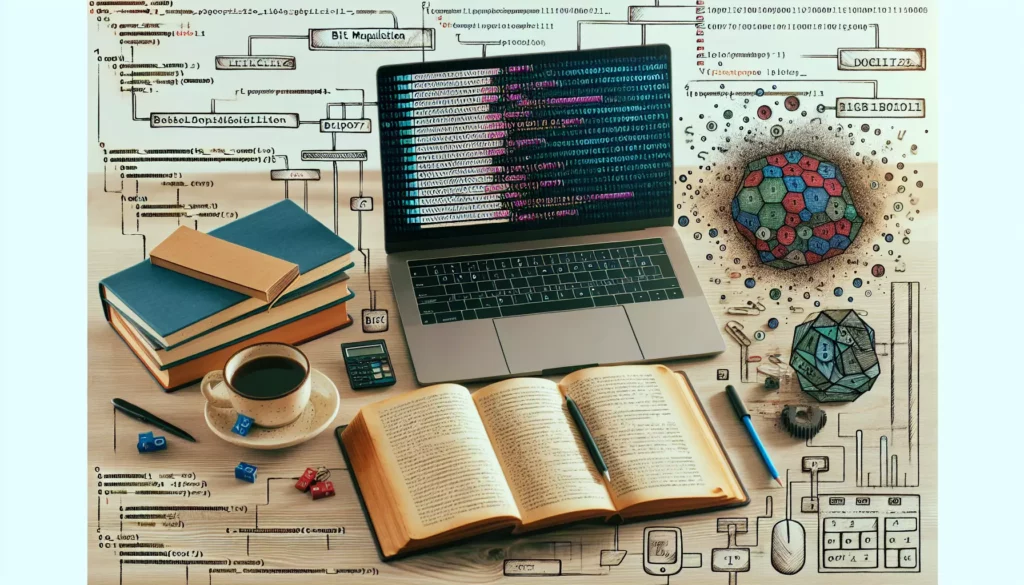
In the world of computer science and programming, bit manipulation is a fundamental skill that often separates good programmers from great ones. As you progress in your coding journey and prepare for technical interviews, especially with major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), understanding and mastering bit manipulation becomes crucial. In this comprehensive guide, we’ll dive deep into the world of bit manipulation, exploring its concepts, applications, and common techniques that you’re likely to encounter in coding interviews.
What is Bit Manipulation?
Bit manipulation refers to the process of applying logical operations on a sequence of bits to achieve a desired result. It involves working with individual bits of a data item through bitwise operations. These operations are performed at the binary level, making them extremely fast and efficient.
In most programming languages, integers are represented using binary numbers, which are sequences of 0s and 1s. Each digit in this sequence is called a bit. Bit manipulation allows us to perform operations directly on these bits, often resulting in more efficient algorithms for certain problems.
Why is Bit Manipulation Important?
Understanding bit manipulation is crucial for several reasons:
- Efficiency: Bit manipulation operations are typically much faster than arithmetic operations, as they are directly executed by the CPU.
- Space Optimization: Using bits to represent data can significantly reduce memory usage in certain scenarios.
- Low-Level Programming: It’s essential for system-level programming, embedded systems, and working with hardware.
- Algorithmic Techniques: Many advanced algorithms and data structures rely on bit manipulation for optimal performance.
- Interview Questions: Bit manipulation is a favorite topic in technical interviews, especially at top tech companies.
Basic Bitwise Operators
Before diving into complex bit manipulation techniques, it’s essential to understand the basic bitwise operators available in most programming languages:
- AND (&): Returns 1 if both bits are 1, otherwise 0.
- OR (|): Returns 1 if at least one of the bits is 1, otherwise 0.
- XOR (^): Returns 1 if the bits are different, otherwise 0.
- NOT (~): Inverts all the bits (0 becomes 1, and 1 becomes 0).
- Left Shift (<<): Shifts all bits to the left by a specified number of positions.
- Right Shift (>>): Shifts all bits to the right by a specified number of positions.
Let’s look at some examples of these operators in action:
// AND operation
5 & 3 = 101 & 011 = 001 = 1
// OR operation
5 | 3 = 101 | 011 = 111 = 7
// XOR operation
5 ^ 3 = 101 ^ 011 = 110 = 6
// NOT operation
~5 = ~(0000 0101) = 1111 1010 = -6 (in two's complement)
// Left Shift
5 << 1 = 0101 << 1 = 1010 = 10
// Right Shift
5 >> 1 = 0101 >> 1 = 0010 = 2
Common Bit Manipulation Techniques
Now that we’ve covered the basics, let’s explore some common bit manipulation techniques that are often used in coding interviews and real-world applications.
1. Checking if a number is even or odd
We can use the AND operator to check if a number is even or odd. The least significant bit of an even number is always 0, while for an odd number, it’s 1.
bool isEven(int n) {
return !(n & 1);
}
bool isOdd(int n) {
return n & 1;
}
2. Multiplying or dividing a number by 2
Left shifting a number by 1 is equivalent to multiplying it by 2, while right shifting by 1 is equivalent to dividing by 2 (integer division).
int multiplyBy2(int n) {
return n << 1;
}
int divideBy2(int n) {
return n >> 1;
}
3. Setting, clearing, and toggling a bit
These operations are fundamental in bit manipulation and are often used in more complex algorithms.
// Set the k-th bit
int setBit(int n, int k) {
return n | (1 << k);
}
// Clear the k-th bit
int clearBit(int n, int k) {
return n & ~(1 << k);
}
// Toggle the k-th bit
int toggleBit(int n, int k) {
return n ^ (1 << k);
}
4. Checking if a number is a power of 2
A number that is a power of 2 has only one bit set in its binary representation. We can use this property to check if a number is a power of 2.
bool isPowerOfTwo(int n) {
return n > 0 && !(n & (n - 1));
}
5. Counting set bits (popcount)
Counting the number of set bits (1s) in a binary number is a common operation. Here’s an efficient way to do it:
int countSetBits(int n) {
int count = 0;
while (n) {
count += n & 1;
n >>= 1;
}
return count;
}
6. Finding the rightmost set bit
This technique is useful in various algorithms, including those dealing with binary search trees.
int rightmostSetBit(int n) {
return n & -n;
}
Advanced Bit Manipulation Techniques
As you progress in your coding skills, you’ll encounter more advanced bit manipulation techniques. Let’s explore some of these:
1. XOR Swapping
XOR can be used to swap two numbers without using a temporary variable:
void xorSwap(int &a, int &b) {
a ^= b;
b ^= a;
a ^= b;
}
2. Finding the missing number
Given an array containing n distinct numbers taken from 0, 1, 2, …, n, find the one that is missing. This can be solved efficiently using XOR.
int findMissingNumber(vector<int>& nums) {
int result = nums.size();
for (int i = 0; i < nums.size(); i++) {
result ^= i ^ nums[i];
}
return result;
}
3. Gray Code
Gray code is a sequence of numbers where adjacent numbers differ by only one bit. It has applications in error correction and digital communications.
int grayCode(int n) {
return n ^ (n >> 1);
}
4. Bitwise AND of Numbers Range
Given a range [m, n], find the bitwise AND of all numbers in this range. This problem tests your understanding of bit patterns.
int rangeBitwiseAnd(int m, int n) {
int shift = 0;
while (m != n) {
m >>= 1;
n >>= 1;
shift++;
}
return m << shift;
}
Bit Manipulation in Real-World Applications
Bit manipulation isn’t just for coding interviews; it has numerous real-world applications:
1. Network Programming
In network programming, bit manipulation is used extensively for tasks like:
- IP address manipulation
- Subnet mask calculations
- Packet header processing
2. Graphics Programming
Bit manipulation is crucial in graphics programming for:
- Color manipulation
- Pixel operations
- Texture compression
3. Cryptography
Many cryptographic algorithms rely heavily on bit manipulation for:
- Encryption and decryption
- Hash functions
- Random number generation
4. Embedded Systems
In embedded systems and microcontroller programming, bit manipulation is used for:
- Configuring hardware registers
- Implementing communication protocols
- Optimizing memory usage
Common Pitfalls and Best Practices
While bit manipulation can be powerful, it’s important to be aware of common pitfalls and follow best practices:
Pitfalls:
- Overflow: Be careful when shifting bits, especially with signed integers.
- Readability: Bit manipulation can make code hard to read if not used judiciously.
- Portability: Some bit manipulation techniques may not work consistently across different architectures or data types.
Best Practices:
- Use meaningful variable names and add comments to explain complex bit operations.
- Be aware of the size and signedness of your integer types.
- Use bitwise operations only when they provide a clear advantage in terms of performance or memory usage.
- Test your bit manipulation code thoroughly, including edge cases.
Preparing for Coding Interviews
If you’re preparing for coding interviews, especially with FAANG companies, here are some tips to master bit manipulation:
- Practice Regularly: Solve bit manipulation problems on platforms like LeetCode, HackerRank, or AlgoCademy.
- Understand the Fundamentals: Make sure you have a solid grasp of binary representation and bitwise operators.
- Learn Common Patterns: Familiarize yourself with common bit manipulation patterns and techniques.
- Analyze Time and Space Complexity: Understand how bit manipulation can improve the efficiency of your algorithms.
- Implement Real-World Applications: Try implementing bit manipulation in practical projects to reinforce your understanding.
Conclusion
Bit manipulation is a powerful tool in a programmer’s arsenal. It allows for efficient algorithms, optimized memory usage, and is crucial in many areas of computer science. By mastering bit manipulation techniques, you’ll not only be better prepared for coding interviews but also equipped to write more efficient and optimized code in your professional career.
Remember, like any skill, proficiency in bit manipulation comes with practice. Don’t be discouraged if it seems challenging at first. Keep practicing, and soon you’ll find yourself manipulating bits with ease, solving complex problems efficiently, and impressing interviewers with your deep understanding of low-level operations.
As you continue your journey in coding education and skills development, platforms like AlgoCademy can provide valuable resources, interactive tutorials, and AI-powered assistance to help you master bit manipulation and other essential programming concepts. Keep coding, keep learning, and embrace the power of bits!