Tree Traversals: Mastering Preorder, Inorder, and Postorder Algorithms
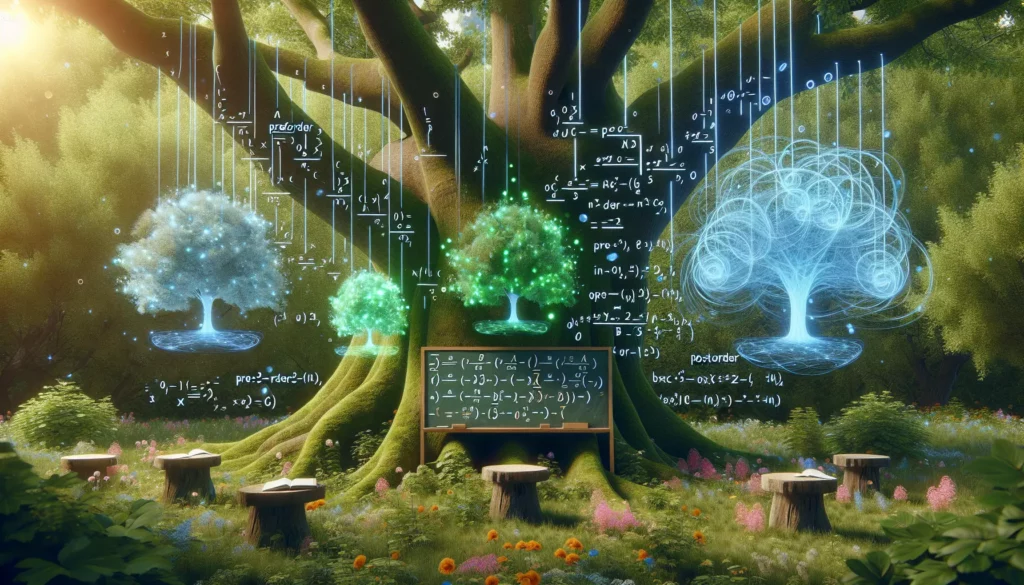
In the world of computer science and programming, tree data structures play a crucial role in organizing and representing hierarchical information. Understanding how to navigate and process these trees efficiently is a fundamental skill for any aspiring programmer or software engineer. In this comprehensive guide, we’ll dive deep into the three primary types of tree traversals: preorder, inorder, and postorder. By the end of this article, you’ll have a solid grasp of these traversal techniques, their implementations, and their practical applications.
What Are Tree Traversals?
Before we delve into the specifics of each traversal method, let’s first understand what tree traversals are and why they’re important.
Tree traversal, also known as tree search, is the process of visiting (checking and/or updating) each node in a tree data structure, exactly once. Unlike linear data structures (Array, Linked List, Queues, Stacks, etc.) which have only one logical way to traverse them, trees can be traversed in different ways.
The importance of tree traversals cannot be overstated. They are used in various applications, including:
- Expression parsing in compilers
- File system navigation
- XML parsing
- Search algorithms in artificial intelligence
- Solving mathematical problems
Now, let’s explore each of the three main types of tree traversals in detail.
1. Preorder Traversal
Preorder traversal is a type of depth-first traversal. In this method, we follow the following sequence:
- Visit the root node
- Traverse the left subtree
- Traverse the right subtree
The “pre” in preorder indicates that the root is visited before the traversals of left and right subtrees.
Preorder Traversal Algorithm
Here’s a recursive implementation of preorder traversal in Python:
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def preorder_traversal(root):
if root:
print(root.value, end=" ") # Visit root
preorder_traversal(root.left) # Traverse left subtree
preorder_traversal(root.right) # Traverse right subtree
Let’s break down how this algorithm works:
- We start by checking if the current node (root) exists. If it doesn’t, we simply return, as there’s nothing to traverse.
- If the node exists, we first print its value. This is the “visit” step.
- Then, we recursively call the function on the left child of the current node. This traverses the entire left subtree.
- After the left subtree is fully traversed, we recursively call the function on the right child, traversing the entire right subtree.
Applications of Preorder Traversal
Preorder traversal has several practical applications:
- Creating a copy of the tree: Since we visit the root first, we can easily create a new node and then recursively create left and right subtrees.
- Prefix expression (Polish notation): Preorder traversal is used to create prefix expressions from expression trees.
- Serializing/Deserializing a tree: Preorder traversal can be used to serialize a binary tree, which can later be deserialized to reconstruct the tree.
2. Inorder Traversal
Inorder traversal is another type of depth-first traversal. In this method, we follow the following sequence:
- Traverse the left subtree
- Visit the root node
- Traverse the right subtree
The “in” in inorder indicates that the root is visited in between the traversals of left and right subtrees.
Inorder Traversal Algorithm
Here’s a recursive implementation of inorder traversal in Python:
def inorder_traversal(root):
if root:
inorder_traversal(root.left) # Traverse left subtree
print(root.value, end=" ") # Visit root
inorder_traversal(root.right) # Traverse right subtree
Let’s break down how this algorithm works:
- We start by checking if the current node (root) exists. If it doesn’t, we simply return, as there’s nothing to traverse.
- If the node exists, we first recursively call the function on the left child of the current node. This traverses the entire left subtree.
- After the left subtree is fully traversed, we print the value of the current node. This is the “visit” step.
- Finally, we recursively call the function on the right child, traversing the entire right subtree.
Applications of Inorder Traversal
Inorder traversal has several important applications:
- Binary Search Tree (BST) operations: Inorder traversal of a BST gives nodes in non-decreasing order.
- Infix expression: Inorder traversal is used to create infix expressions from expression trees.
- Finding the k-th smallest element in a BST: This can be achieved by doing an inorder traversal and keeping a count of visited nodes.
3. Postorder Traversal
Postorder traversal is the third type of depth-first traversal. In this method, we follow the following sequence:
- Traverse the left subtree
- Traverse the right subtree
- Visit the root node
The “post” in postorder indicates that the root is visited after the traversals of left and right subtrees.
Postorder Traversal Algorithm
Here’s a recursive implementation of postorder traversal in Python:
def postorder_traversal(root):
if root:
postorder_traversal(root.left) # Traverse left subtree
postorder_traversal(root.right) # Traverse right subtree
print(root.value, end=" ") # Visit root
Let’s break down how this algorithm works:
- We start by checking if the current node (root) exists. If it doesn’t, we simply return, as there’s nothing to traverse.
- If the node exists, we first recursively call the function on the left child of the current node. This traverses the entire left subtree.
- After the left subtree is fully traversed, we recursively call the function on the right child, traversing the entire right subtree.
- Finally, after both subtrees have been traversed, we print the value of the current node. This is the “visit” step.
Applications of Postorder Traversal
Postorder traversal has several practical applications:
- Deleting the tree: Postorder is used to delete the tree, as we need to delete the left and right subtrees before deleting the root.
- Postfix expression (Reverse Polish notation): Postorder traversal is used to create postfix expressions from expression trees.
- Finding the height of the tree: By using postorder traversal, we can calculate the height of the tree efficiently.
Comparison of Traversal Methods
To better understand the differences between these traversal methods, let’s consider a simple binary tree and see how each traversal would process it:
1
/ \
2 3
/ \ / \
4 5 6 7
For this tree:
- Preorder traversal would visit nodes in the order: 1 2 4 5 3 6 7
- Inorder traversal would visit nodes in the order: 4 2 5 1 6 3 7
- Postorder traversal would visit nodes in the order: 4 5 2 6 7 3 1
As you can see, each traversal method produces a different order of node visits, which can be useful for different purposes.
Iterative Implementations
While we’ve focused on recursive implementations so far, it’s also possible (and sometimes preferable) to implement these traversals iteratively. Iterative implementations can be more efficient in terms of stack space, especially for very deep trees.
Here’s an example of an iterative implementation of inorder traversal:
def iterative_inorder_traversal(root):
stack = []
current = root
while current or stack:
# Reach the left most Node of the current Node
while current:
stack.append(current)
current = current.left
# Current must be NULL at this point
current = stack.pop()
print(current.value, end=" ")
# We have visited the node and its left subtree.
# Now, it's right subtree's turn
current = current.right
This iterative approach mimics the recursive process by using a stack to keep track of nodes to be processed.
Time and Space Complexity
For all three traversal methods (preorder, inorder, and postorder), the time complexity is O(n), where n is the number of nodes in the tree. This is because each node is visited exactly once.
The space complexity for the recursive implementations is O(h), where h is the height of the tree. This is due to the recursive call stack. In the worst case (a completely unbalanced tree), this could be O(n), but for a balanced tree, it would be O(log n).
For iterative implementations, the space complexity is also O(h) for the explicit stack used.
Advanced Topics: Morris Traversal
While not as commonly used, it’s worth mentioning the Morris Traversal algorithm. This method allows for inorder traversal using O(1) extra space and without using recursion or a stack.
The basic idea of Morris traversal is to create links to Inorder successor and print the data using these links, and finally revert the changes to restore original tree.
Here’s a Python implementation of Morris Traversal for inorder traversal:
def morris_inorder_traversal(root):
current = root
while current is not None:
if current.left is None:
print(current.value, end=" ")
current = current.right
else:
# Find the inorder predecessor of current
pre = current.left
while pre.right is not None and pre.right != current:
pre = pre.right
if pre.right is None:
# Make current as the right child of its inorder predecessor
pre.right = current
current = current.left
else:
# Revert the changes made in the 'if' part to restore the original tree
pre.right = None
print(current.value, end=" ")
current = current.right
This algorithm has a time complexity of O(n) and a space complexity of O(1), making it very efficient for memory-constrained environments.
Practical Applications in Software Development
Understanding tree traversals is not just an academic exercise; it has real-world applications in software development. Here are some examples:
- File Systems: File systems are often represented as trees. Traversing these trees is necessary for operations like searching for files, calculating directory sizes, or backing up data.
- HTML/XML Parsing: The Document Object Model (DOM) in web development is a tree structure. Traversing this tree is crucial for manipulating web pages dynamically.
- Compiler Design: In compiler design, abstract syntax trees are used to represent the structure of program code. Different traversals are used for different phases of compilation.
- Game Development: In game development, scene graphs (which represent the logical and spatial representation of a graphical scene) are often traversed to render scenes or perform collision detection.
- Database Indexing: B-trees and other tree structures are used in database indexing. Efficient traversal of these trees is crucial for database performance.
Conclusion
Tree traversals are fundamental algorithms in computer science, with wide-ranging applications in software development. Understanding preorder, inorder, and postorder traversals provides a solid foundation for working with tree-based data structures and algorithms.
As you continue your journey in programming and computer science, you’ll find that these traversal methods come up frequently, whether you’re working on data processing, compiler design, or web development. The ability to choose the right traversal method for a given problem and implement it efficiently is a valuable skill for any programmer.
Remember, practice is key to mastering these concepts. Try implementing these traversals on different tree structures, and consider how you might use them in your own projects. As you gain more experience, you’ll develop an intuition for when and how to apply these powerful algorithms.
Happy coding, and may your trees always be well-traversed!