Mastering the Sliding Window Technique: A Comprehensive Guide
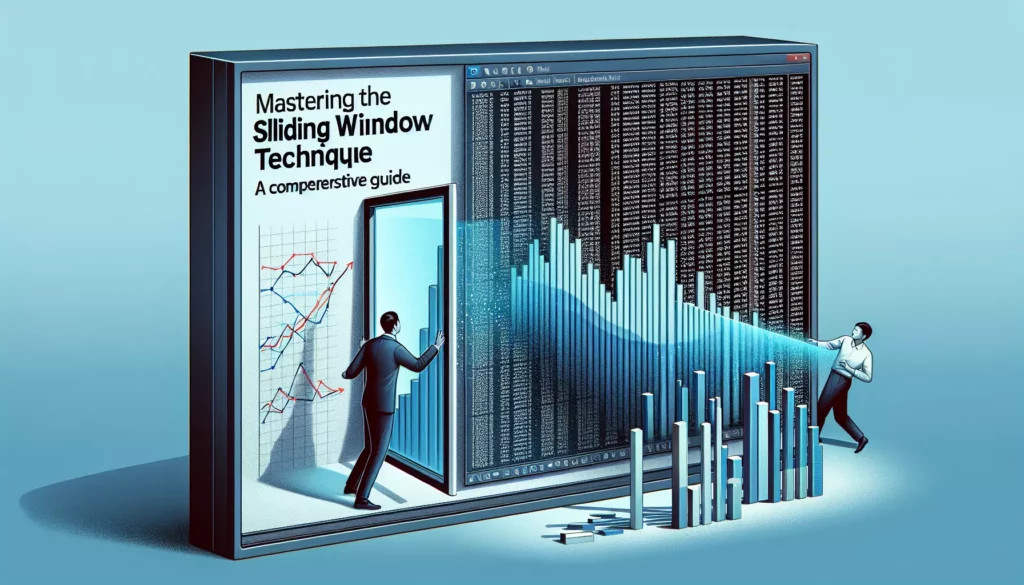
In the world of algorithmic problem-solving, efficiency is key. As developers, we’re constantly seeking ways to optimize our code and tackle complex problems with elegance. One powerful technique that often comes to the rescue is the Sliding Window algorithm. This method is particularly useful when dealing with arrays or strings, offering a way to process data in a linear time complexity. In this comprehensive guide, we’ll dive deep into the Sliding Window technique, exploring its concepts, applications, and implementation strategies.
What is the Sliding Window Technique?
The Sliding Window technique is an algorithmic paradigm that involves maintaining a subset of elements (the “window”) as you iterate through a larger set of data. This window can either grow or shrink depending on certain conditions, allowing you to efficiently process the data without unnecessary repetition.
Imagine you’re looking through a long document with a magnifying glass. Instead of reading every word individually, you move the magnifying glass (your window) across the text, focusing on a specific section at a time. This is essentially what the Sliding Window technique does in programming.
When to Use the Sliding Window Technique
The Sliding Window approach is particularly effective in scenarios where you need to perform operations on a contiguous sequence of elements. Some common use cases include:
- Finding the longest substring with certain properties
- Calculating a moving average
- Detecting patterns in strings or arrays
- Solving problems related to subarrays or substrings
This technique shines when you’re dealing with problems that would otherwise require nested loops, potentially leading to quadratic time complexity (O(n²)). By using a Sliding Window, you can often reduce the time complexity to linear (O(n)).
Types of Sliding Window
There are two main types of Sliding Window algorithms:
1. Fixed-size Window
In this variant, the size of the window remains constant throughout the iteration. This is useful when you’re dealing with problems that require you to consider a fixed number of elements at a time.
2. Variable-size Window
Here, the window size can change dynamically based on certain conditions. This type is more flexible and can be applied to a wider range of problems, especially when you’re looking for optimal subarrays or substrings that meet specific criteria.
Implementing the Sliding Window Technique
Let’s walk through the implementation of both fixed-size and variable-size Sliding Window algorithms with some practical examples.
Fixed-size Window Example: Moving Average
Suppose we want to calculate the moving average of a series of numbers with a window size of 3. Here’s how we can implement this using a fixed-size Sliding Window:
def moving_average(nums, k):
n = len(nums)
if n < k:
return []
window_sum = sum(nums[:k])
result = [window_sum / k]
for i in range(k, n):
window_sum = window_sum - nums[i-k] + nums[i]
result.append(window_sum / k)
return result
# Example usage
numbers = [1, 3, 5, 7, 9, 2, 4, 6, 8]
window_size = 3
averages = moving_average(numbers, window_size)
print(averages)
In this example, we initialize the window with the first ‘k’ elements and then slide it across the array, updating the sum and calculating the average for each position.
Variable-size Window Example: Longest Substring with K Distinct Characters
Now, let’s tackle a more complex problem using a variable-size Sliding Window. We’ll find the longest substring with at most K distinct characters:
def longest_substring_with_k_distinct(s, k):
char_count = {}
max_length = 0
start = 0
for end in range(len(s)):
char_count[s[end]] = char_count.get(s[end], 0) + 1
while len(char_count) > k:
char_count[s[start]] -= 1
if char_count[s[start]] == 0:
del char_count[s[start]]
start += 1
max_length = max(max_length, end - start + 1)
return max_length
# Example usage
string = "aabacbebebe"
k = 3
result = longest_substring_with_k_distinct(string, k)
print(f"Length of the longest substring with at most {k} distinct characters: {result}")
In this implementation, we use a dictionary to keep track of character frequencies. We expand the window until we have more than K distinct characters, then contract it from the start until we’re back to K distinct characters.
Common Patterns in Sliding Window Problems
As you work with more Sliding Window problems, you’ll start to recognize certain patterns. Here are some common ones:
1. Window Expansion and Contraction
Many Sliding Window problems involve expanding the window until a certain condition is met, then contracting it from the start. This pattern is evident in problems like “Longest Substring with At Most K Distinct Characters” or “Minimum Window Substring”.
2. Maintaining a Running Sum or Product
For problems involving sums or products of subarrays, it’s common to maintain a running total that’s updated as the window slides. This is seen in problems like “Maximum Sum Subarray of Size K” or “Product of Array Except Self”.
3. Using Auxiliary Data Structures
Often, you’ll need to use additional data structures like HashMaps or Heaps to keep track of elements within the window. This is particularly useful for problems involving frequencies or ordering of elements.
4. Two-pointer Technique
Many Sliding Window problems can be solved using two pointers – one for the start of the window and one for the end. This allows for efficient traversal and window manipulation.
Advanced Sliding Window Techniques
As you become more comfortable with basic Sliding Window problems, you can start exploring more advanced techniques and variations:
1. Multi-window Approach
Some problems might require you to maintain multiple windows simultaneously. For example, finding the smallest window that contains all elements of another array might involve keeping track of two windows – one for each array.
2. Circular Sliding Window
When dealing with circular arrays or strings, you might need to implement a circular Sliding Window. This involves wrapping around to the beginning of the array when you reach the end.
3. Dynamic Window Size Adjustment
In some cases, you might need to dynamically adjust the window size based on complex conditions. This could involve growing or shrinking the window by varying amounts at each step.
4. Sliding Window with Binary Search
For certain problems, combining the Sliding Window technique with Binary Search can lead to even more efficient solutions. This is particularly useful when you need to find an optimal window size.
Optimizing Sliding Window Solutions
While the Sliding Window technique is inherently efficient, there are ways to further optimize your solutions:
1. Minimize Window Updates
Try to update the window contents as infrequently as possible. If you can maintain the necessary information without updating at every step, you’ll reduce the overall computational cost.
2. Use Appropriate Data Structures
Choose data structures that support efficient operations for your specific problem. For example, if you need to find the maximum element in a window, consider using a deque (double-ended queue) for O(1) operations.
3. Avoid Unnecessary Calculations
Look for opportunities to reuse previously computed values. For instance, in a problem involving sums, you can often update the sum incrementally rather than recalculating it for each window.
4. Early Termination
In some cases, you might be able to terminate the algorithm early if you’ve found a solution that meets certain criteria. This can significantly reduce the average-case running time.
Common Pitfalls and How to Avoid Them
As with any algorithmic technique, there are some common mistakes to watch out for when implementing Sliding Window solutions:
1. Off-by-One Errors
Be careful with your window boundaries, especially when dealing with zero-based indexing. Double-check your loop conditions and window size calculations.
2. Forgetting to Reset Window State
If you’re solving multiple test cases or reusing your Sliding Window function, make sure to reset any window-related variables between runs.
3. Inefficient Window Updates
Avoid unnecessary operations when updating your window. For example, don’t remove and add the same element if you’re just sliding the window by one position.
4. Overlooking Edge Cases
Consider edge cases like empty input, single-element input, or when the window size is larger than the input size. These can often lead to subtle bugs if not handled properly.
Sliding Window in Technical Interviews
The Sliding Window technique is a favorite among interviewers, especially at major tech companies. Here are some tips for tackling Sliding Window problems in interviews:
1. Identify the Window
When presented with a problem, try to identify if there’s a natural “window” in the problem statement. Look for phrases like “contiguous subarray” or “substring”.
2. Determine Window Behavior
Decide whether you need a fixed-size or variable-size window. This will guide your implementation approach.
3. Choose Your Data Structures
Based on the problem requirements, select appropriate data structures to maintain your window state. This could be as simple as two pointers or as complex as a combination of a HashMap and a priority queue.
4. Implement Efficiently
Focus on implementing your solution with the best possible time and space complexity. Interviewers often look for candidates who can optimize their solutions.
5. Test Your Solution
Before declaring your solution complete, test it with various inputs, including edge cases. This demonstrates thoroughness and attention to detail.
Conclusion
The Sliding Window technique is a powerful tool in any programmer’s arsenal. Its ability to solve complex problems with linear time complexity makes it invaluable for optimizing algorithms and tackling challenging coding interviews. By understanding the core concepts, recognizing common patterns, and practicing with diverse problems, you can master this technique and significantly enhance your problem-solving skills.
Remember, like any algorithmic paradigm, proficiency comes with practice. Challenge yourself with a variety of Sliding Window problems, experiment with different implementations, and don’t hesitate to explore advanced variations. With time and effort, you’ll find yourself confidently applying this technique to solve an array of programming challenges.
As you continue your journey in algorithmic problem-solving, keep the Sliding Window technique close at hand. It’s not just a method for passing technical interviews; it’s a fundamental approach that can help you write more efficient, elegant code in your day-to-day programming tasks. Happy coding!