How to Ace Remote Coding Interviews: A Comprehensive Guide
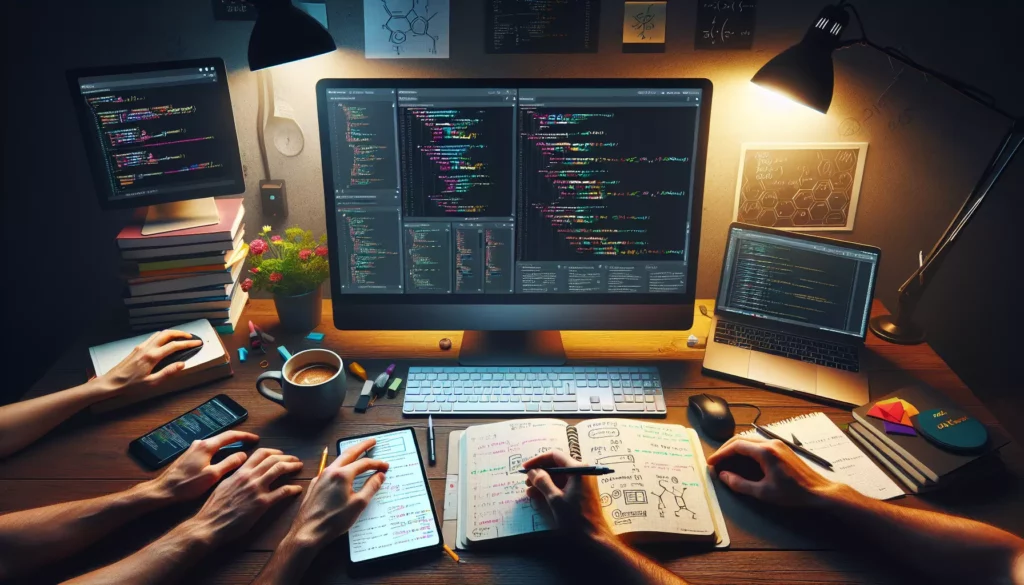
In today’s digital age, remote coding interviews have become increasingly common, especially in the tech industry. With the rise of distributed teams and global talent acquisition, companies are leveraging technology to conduct interviews from anywhere in the world. This shift presents both opportunities and challenges for job seekers. In this comprehensive guide, we’ll explore strategies and tips to help you excel in remote coding interviews, covering everything from preparation to execution.
Understanding the Remote Coding Interview Process
Before diving into specific strategies, it’s essential to understand what a remote coding interview typically entails. These interviews usually consist of several components:
- Introduction and background discussion
- Technical questions about your experience and knowledge
- One or more coding challenges
- System design or architecture discussions (for more senior roles)
- Behavioral questions
- Q&A session
The coding challenges are often conducted using online coding platforms or collaborative tools that allow the interviewer to see your code in real-time. This setup mimics pair programming and enables the interviewer to assess your problem-solving approach, coding style, and communication skills.
Preparing for Your Remote Coding Interview
1. Master the Fundamentals
Regardless of the specific role you’re applying for, a solid grasp of fundamental computer science concepts is crucial. Focus on:
- Data structures (arrays, linked lists, trees, graphs, etc.)
- Algorithms (sorting, searching, dynamic programming, etc.)
- Time and space complexity analysis
- Object-oriented programming principles
- Basic system design concepts
Platforms like AlgoCademy offer structured learning paths and interactive tutorials to help you reinforce these concepts and practice their application in coding challenges.
2. Practice, Practice, Practice
The saying “practice makes perfect” couldn’t be more true for coding interviews. Regularly solving coding problems will help you:
- Improve your problem-solving skills
- Become more comfortable with different types of questions
- Enhance your ability to explain your thought process
- Reduce anxiety during the actual interview
Utilize platforms like LeetCode, HackerRank, or AlgoCademy’s practice modules to work through a variety of coding challenges. Aim to solve at least one problem daily, gradually increasing the difficulty as you improve.
3. Familiarize Yourself with Online Coding Environments
Many companies use online coding platforms for remote interviews. Get comfortable with these environments by:
- Practicing on platforms like CoderPad or HackerRank’s code pad
- Setting up a similar environment on your local machine
- Learning keyboard shortcuts for efficiency
- Understanding how to run and test your code in these environments
4. Brush Up on Your Chosen Programming Language
While most companies allow you to choose your preferred programming language, it’s crucial to be proficient in it. Make sure you:
- Know the syntax inside and out
- Understand language-specific features and best practices
- Are familiar with the standard library and commonly used functions
- Can write clean, readable code quickly
For example, if you’re using Python, you might want to refresh your knowledge of list comprehensions, lambda functions, and the collections module.
5. Prepare Your Technical Environment
A smooth technical setup is crucial for a successful remote interview. Ensure you have:
- A reliable internet connection
- A quiet, well-lit space for the interview
- A working webcam and microphone
- The necessary software installed (e.g., Zoom, Skype, or the company’s preferred platform)
- A backup plan in case of technical issues (e.g., a phone number to call)
Strategies for Excelling During the Interview
1. Communicate Clearly and Constantly
In a remote setting, clear communication becomes even more critical. Follow these tips:
- Speak clearly and at a moderate pace
- Use the STAR method (Situation, Task, Action, Result) when answering behavioral questions
- Verbalize your thought process as you work through coding problems
- Ask clarifying questions when needed
- Confirm that the interviewer can see your screen and hear you properly
2. Follow a Structured Problem-Solving Approach
When tackling coding challenges, use a systematic approach:
- Clarify the problem and requirements
- Discuss potential approaches and trade-offs
- Choose an approach and outline your solution
- Implement the solution, explaining your code as you go
- Test your solution with various inputs, including edge cases
- Analyze the time and space complexity
- Discuss potential optimizations or alternative solutions
This structured approach demonstrates your problem-solving skills and helps you stay organized during the interview.
3. Write Clean, Readable Code
Even under time pressure, strive to write clean, well-organized code. This includes:
- Using meaningful variable and function names
- Adding comments to explain complex logic
- Properly indenting your code
- Breaking down complex problems into smaller functions
- Following language-specific conventions and best practices
Here’s an example of clean, readable code in Python:
def is_palindrome(s: str) -> bool:
"""
Check if a given string is a palindrome.
Args:
s (str): The input string to check.
Returns:
bool: True if the string is a palindrome, False otherwise.
"""
# Remove non-alphanumeric characters and convert to lowercase
cleaned_s = ''.join(char.lower() for char in s if char.isalnum())
# Compare the string with its reverse
return cleaned_s == cleaned_s[::-1]
# Test the function
test_cases = ["A man, a plan, a canal: Panama", "race a car", ""]
for test in test_cases:
print(f"Is '{test}' a palindrome? {is_palindrome(test)}")
4. Manage Your Time Effectively
Time management is crucial in coding interviews. Here are some tips:
- Ask about the time allocated for each section of the interview
- Set a mental timer for each problem
- If you’re stuck, communicate this to the interviewer and ask for hints
- Don’t spend too much time optimizing prematurely; get a working solution first, then improve it
5. Handle Technical Issues Gracefully
If you encounter technical problems during the interview:
- Stay calm and professional
- Communicate the issue clearly to the interviewer
- Have a backup plan ready (e.g., switching to a phone call or rescheduling)
- Test your setup thoroughly before the interview to minimize the risk of issues
Advanced Topics for Senior-Level Interviews
For more experienced developers or those applying for senior positions, remote coding interviews may include additional components:
1. System Design Questions
These questions assess your ability to design large-scale systems. To prepare:
- Study common system design patterns and architectures
- Understand concepts like load balancing, caching, and database sharding
- Practice explaining your design decisions and trade-offs
- Be familiar with real-world examples of large-scale systems
2. Architecture and Design Patterns
Senior developers are often expected to have a deep understanding of software architecture and design patterns. Focus on:
- Common design patterns (e.g., Singleton, Factory, Observer)
- Architectural patterns (e.g., MVC, MVVM, Microservices)
- SOLID principles
- How to apply these concepts in real-world scenarios
3. Leadership and Project Management
For senior roles, interviewers may ask about your experience with:
- Leading development teams
- Managing complex projects
- Mentoring junior developers
- Making architectural decisions
- Handling conflicts and challenges in a team setting
Prepare specific examples from your past experiences to illustrate your skills in these areas.
Post-Interview Steps
1. Follow Up
After the interview:
- Send a thank-you email to your interviewer(s) within 24 hours
- Reiterate your interest in the position
- Briefly mention a specific point from the interview that you found interesting
- Ask about the next steps in the process
2. Reflect and Improve
Regardless of the outcome, use each interview as a learning experience:
- Review the questions you were asked and research any topics you struggled with
- Practice similar problems to reinforce your skills
- Seek feedback from the interviewer or recruiter if possible
- Adjust your preparation strategy based on your performance
Leveraging AlgoCademy for Interview Preparation
AlgoCademy offers a comprehensive platform to help you prepare for remote coding interviews:
- Interactive Tutorials: Learn and practice fundamental concepts through hands-on coding exercises.
- Problem Sets: Access a vast library of coding challenges, categorized by difficulty and topic.
- AI-Powered Assistance: Get personalized hints and explanations as you work through problems.
- Mock Interviews: Simulate real interview conditions with timed coding challenges and feedback.
- Progress Tracking: Monitor your improvement over time and identify areas for further study.
- Community Support: Connect with other learners, share experiences, and get advice from experienced developers.
By incorporating AlgoCademy into your preparation routine, you can systematically build the skills and confidence needed to excel in remote coding interviews.
Conclusion
Remote coding interviews present unique challenges, but with the right preparation and approach, they also offer opportunities to showcase your skills to potential employers around the world. By mastering the fundamentals, practicing regularly, and developing strong communication skills, you can position yourself for success in these interviews.
Remember that interviewing is a skill in itself, and like any skill, it improves with practice. Each interview, regardless of the outcome, is an opportunity to learn and grow as a developer. Stay persistent, keep refining your approach, and leverage resources like AlgoCademy to continually enhance your coding and problem-solving abilities.
As you embark on your journey to ace remote coding interviews, remember that the goal is not just to get the job, but to become a better developer in the process. Embrace the challenges, stay curious, and keep coding. With dedication and the right resources, you’ll be well-equipped to tackle any remote coding interview that comes your way.