Top Cloudflare Interview Questions: Ace Your Next Tech Interview
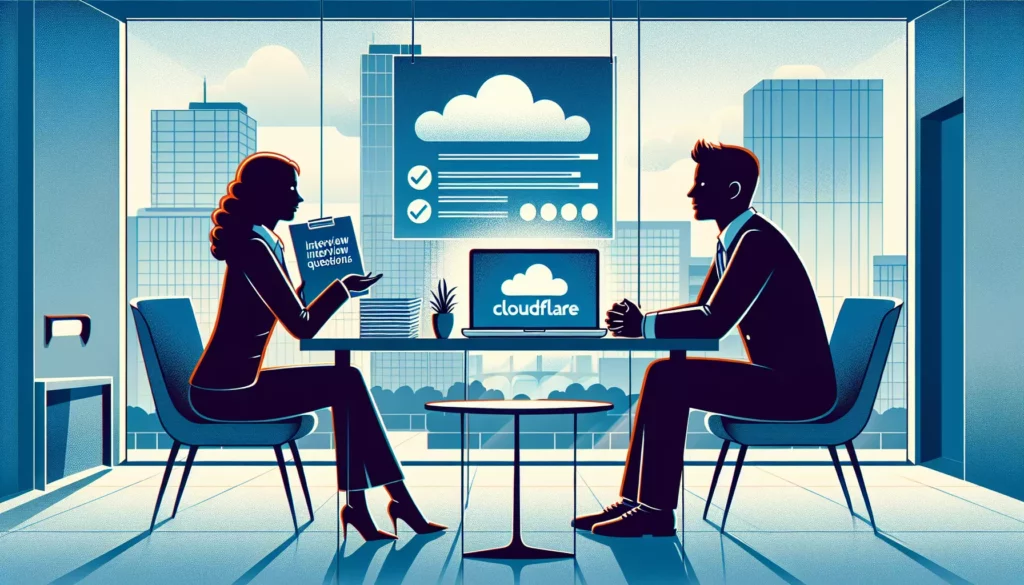
Are you preparing for a technical interview at Cloudflare? As one of the leading companies in internet security and performance, Cloudflare is known for its rigorous interview process. To help you succeed, we’ve compiled a comprehensive list of Cloudflare interview questions, along with tips and strategies to ace your interview. Whether you’re a seasoned professional or a fresh graduate, this guide will give you the edge you need to stand out from the competition.
Table of Contents
- Understanding Cloudflare
- The Cloudflare Interview Process
- Technical Interview Questions
- System Design Questions
- Behavioral Interview Questions
- Coding Challenges
- Tips for Interview Success
- Preparation Resources
- Conclusion
Understanding Cloudflare
Before diving into the interview questions, it’s crucial to have a solid understanding of Cloudflare and its services. Cloudflare is a global network designed to make everything you connect to the Internet secure, private, fast, and reliable. Some key points to remember:
- Cloudflare provides Content Delivery Network (CDN) services
- It offers DDoS protection and Internet security
- The company focuses on improving website performance and reducing latency
- Cloudflare operates one of the world’s largest networks, spanning more than 200 cities worldwide
Having this context will help you better understand the types of questions you might encounter during your interview.
The Cloudflare Interview Process
The Cloudflare interview process typically consists of several stages:
- Initial phone screen with a recruiter
- Technical phone interview or online coding assessment
- On-site interviews (or virtual equivalent) including:
- Multiple technical interviews
- System design interview
- Behavioral interviews
Each stage is designed to assess different aspects of your skills, knowledge, and cultural fit within the company.
Technical Interview Questions
Cloudflare’s technical interviews often focus on core computer science concepts, networking, and security. Here are some common technical questions you might encounter:
1. Networking and Internet Protocols
- Explain the difference between TCP and UDP. When would you use one over the other?
- What happens when you type a URL into a browser and press enter?
- Describe the OSI model and its layers.
- How does DNS resolution work?
- Explain the purpose and functioning of HTTPS.
2. Security Concepts
- What is a DDoS attack, and how can it be mitigated?
- Explain the concept of SSL/TLS and how it ensures secure communication.
- What are the main differences between symmetric and asymmetric encryption?
- How does a firewall work, and what are its limitations?
- Describe the concept of Content Security Policy (CSP).
3. Algorithms and Data Structures
- Implement a function to reverse a linked list.
- Explain the time and space complexity of quicksort. How can it be optimized?
- Design a data structure for a least recently used (LRU) cache.
- Implement a function to find the longest palindromic substring in a given string.
- Explain how a hash table works and how to handle collisions.
Sample Technical Question and Answer
Let’s look at a sample technical question and how you might approach it:
Question: Implement a function to check if a binary tree is balanced.
Here’s a possible solution in Python:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def is_balanced(root):
def check_balance(node):
if not node:
return 0
left_height = check_balance(node.left)
if left_height == -1:
return -1
right_height = check_balance(node.right)
if right_height == -1:
return -1
if abs(left_height - right_height) > 1:
return -1
return max(left_height, right_height) + 1
return check_balance(root) != -1
Explanation:
- We define a helper function
check_balance
that returns the height of the tree if it’s balanced, or -1 if it’s not balanced. - We recursively check the left and right subtrees.
- If at any point we find an unbalanced subtree (height difference > 1), we return -1.
- The main function
is_balanced
returns True if the final result is not -1.
System Design Questions
System design questions are a crucial part of the Cloudflare interview process, especially for more senior positions. These questions assess your ability to design scalable, efficient, and robust systems. Here are some examples:
- Design a global content delivery network (CDN).
- How would you design a system to handle millions of DNS queries per second?
- Design a distributed key-value store.
- How would you implement a rate limiting system for an API?
- Design a system for real-time analytics of website traffic.
Tips for Approaching System Design Questions
- Clarify requirements and constraints
- Start with a high-level design
- Dive into component details
- Discuss trade-offs and potential optimizations
- Consider scalability, reliability, and security aspects
Sample System Design Question and Approach
Question: Design a global content delivery network (CDN).
Approach:
- Clarify requirements:
- Global reach
- Low latency content delivery
- Support for static and dynamic content
- Scalability to handle millions of requests
- High-level design:
- Distributed network of edge servers
- Origin servers to store the original content
- Load balancers to distribute traffic
- DNS-based request routing
- Component details:
- Edge servers: Caching mechanisms, content freshness
- Request routing: Anycast, GeoDNS
- Content management: Push vs. pull, invalidation
- Optimizations:
- Content compression
- TCP optimizations
- SSL/TLS termination at edge
- Scalability and reliability:
- Horizontal scaling of edge servers
- Redundancy and failover mechanisms
- DDoS protection
Behavioral Interview Questions
Behavioral questions are designed to assess your soft skills, problem-solving abilities, and cultural fit. Here are some common behavioral questions you might encounter in a Cloudflare interview:
- Tell me about a time when you had to deal with a difficult team member.
- Describe a situation where you had to make a decision with incomplete information.
- How do you stay updated with the latest technologies and industry trends?
- Give an example of a time when you had to explain a complex technical concept to a non-technical audience.
- Describe a project you’re particularly proud of and your role in it.
Tips for Answering Behavioral Questions
- Use the STAR method (Situation, Task, Action, Result) to structure your answers
- Provide specific examples from your past experiences
- Focus on your individual contributions and learnings
- Be honest and reflective, including lessons learned from challenges
- Align your answers with Cloudflare’s values and culture
Coding Challenges
Cloudflare often includes coding challenges as part of their interview process. These may be conducted during a live interview or as a take-home assignment. Here are some tips and example challenges:
Tips for Coding Challenges
- Practice coding on a whiteboard or in a simple text editor
- Communicate your thought process clearly
- Start with a brute force solution, then optimize
- Consider edge cases and error handling
- Write clean, readable code
Example Coding Challenge
Challenge: Implement a rate limiter
Design and implement a rate limiter that allows n requests per second for a given API key.
import time
class RateLimiter:
def __init__(self, limit, time_window):
self.limit = limit
self.time_window = time_window
self.requests = {}
def is_allowed(self, api_key):
current_time = time.time()
if api_key not in self.requests:
self.requests[api_key] = []
# Remove old requests
self.requests[api_key] = [req for req in self.requests[api_key] if current_time - req < self.time_window]
if len(self.requests[api_key]) < self.limit:
self.requests[api_key].append(current_time)
return True
return False
# Usage
limiter = RateLimiter(5, 1) # 5 requests per second
api_key = "user123"
for _ in range(10):
if limiter.is_allowed(api_key):
print("Request allowed")
else:
print("Request blocked")
time.sleep(0.1)
This implementation uses a sliding window approach to track requests within the specified time window.
Tips for Interview Success
Here are some general tips to help you succeed in your Cloudflare interview:
- Research Cloudflare thoroughly, including its products, services, and recent news
- Review fundamental computer science concepts, especially networking and security
- Practice coding problems regularly, focusing on efficiency and clean code
- Prepare thoughtful questions to ask your interviewers
- Be ready to discuss your past projects and experiences in detail
- Stay calm and composed during the interview, even if you encounter difficult questions
- Show enthusiasm for the role and the company
- Follow up after the interview with a thank-you note
Preparation Resources
To help you prepare for your Cloudflare interview, consider using the following resources:
- LeetCode: Practice coding problems and algorithms
- System Design Primer: A comprehensive guide to system design concepts
- Cloudflare Learning Center: Learn about Cloudflare’s products and technologies
- Networking books: “Computer Networking: A Top-Down Approach” by Kurose and Ross
- Security resources: OWASP Top 10, “Web Application Hacker’s Handbook”
- Behavioral interview guides: Practice common behavioral questions
Conclusion
Preparing for a Cloudflare interview requires a combination of technical knowledge, problem-solving skills, and the ability to communicate effectively. By focusing on the key areas outlined in this guide – networking, security, algorithms, system design, and behavioral aspects – you’ll be well-equipped to tackle the challenges of the interview process.
Remember, the goal of the interview is not just to test your knowledge, but also to assess your thought process, your ability to learn, and your fit within the Cloudflare team. Approach each question as an opportunity to showcase your skills and passion for technology.
With thorough preparation and a positive attitude, you’ll be well on your way to impressing your interviewers and landing that dream job at Cloudflare. Good luck with your interview!