Top Spotify Interview Questions: Ace Your Tech Interview
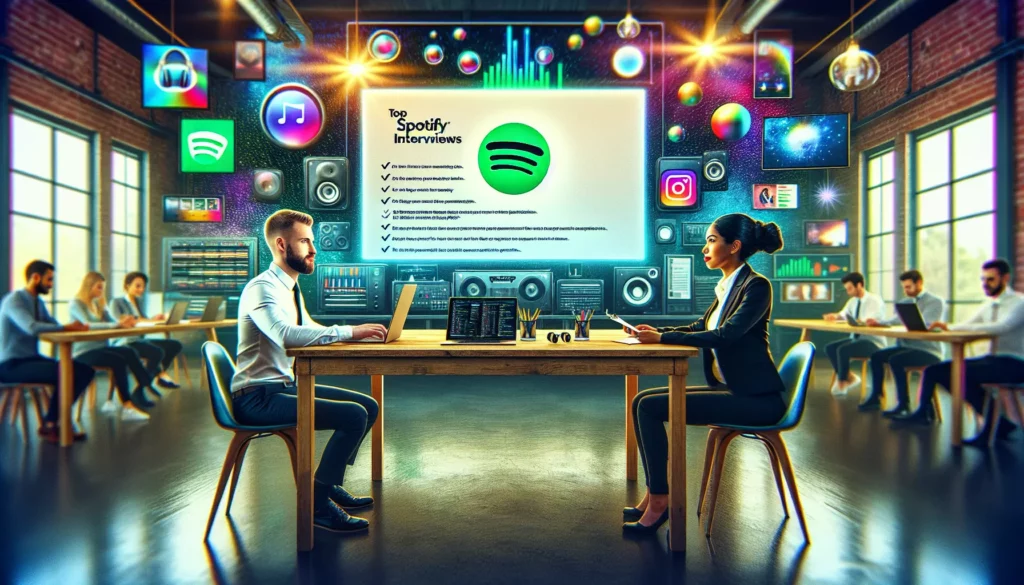
Are you gearing up for a technical interview at Spotify? As one of the leading music streaming platforms, Spotify is known for its innovative technology and challenging interview process. In this comprehensive guide, we’ll explore some of the most common Spotify interview questions, provide insights into their interview process, and offer tips to help you succeed. Whether you’re a seasoned developer or just starting your tech career, this article will help you prepare for your Spotify interview with confidence.
Table of Contents
- Understanding Spotify’s Interview Process
- Technical Interview Questions
- System Design Questions
- Behavioral Interview Questions
- Coding Challenges
- Preparation Tips
- Conclusion
1. Understanding Spotify’s Interview Process
Before diving into specific questions, it’s essential to understand Spotify’s interview process. Typically, it consists of several stages:
- Initial Screening: This usually involves a phone or video call with a recruiter to discuss your background and the role.
- Technical Phone Screen: You’ll likely have a technical discussion or a coding exercise with an engineer.
- On-site Interviews: This stage usually involves multiple rounds, including:
- Coding interviews
- System design discussions
- Behavioral interviews
- Final Decision: After the on-site interviews, the hiring team will make a decision.
Now, let’s explore some common questions you might encounter during these stages.
2. Technical Interview Questions
Spotify’s technical questions often focus on data structures, algorithms, and problem-solving skills. Here are some examples:
2.1. Implement a Playlist Shuffling Algorithm
Question: Design and implement an algorithm to shuffle a playlist of songs, ensuring each song is played exactly once and the order is random.
Solution: This problem can be solved using the Fisher-Yates shuffle algorithm. Here’s a Python implementation:
import random
def shuffle_playlist(playlist):
n = len(playlist)
for i in range(n - 1, 0, -1):
j = random.randint(0, i)
playlist[i], playlist[j] = playlist[j], playlist[i]
return playlist
# Example usage
original_playlist = ['Song A', 'Song B', 'Song C', 'Song D', 'Song E']
shuffled_playlist = shuffle_playlist(original_playlist.copy())
print(f"Original playlist: {original_playlist}")
print(f"Shuffled playlist: {shuffled_playlist}")
2.2. Implement a Cache with a Least Recently Used (LRU) Eviction Policy
Question: Design and implement a data structure for a Least Recently Used (LRU) cache. It should support the following operations: get and put.
- get(key) – Get the value (will always be positive) of the key if the key exists in the cache, otherwise return -1.
- put(key, value) – Set or insert the value if the key is not already present. When the cache reaches its capacity, it should invalidate the least recently used item before inserting a new item.
The cache is initialized with a positive capacity.
Solution: We can use a combination of a hash map and a doubly linked list to implement an LRU cache with O(1) time complexity for both operations.
class Node:
def __init__(self, key=0, value=0):
self.key = key
self.value = value
self.prev = None
self.next = None
class LRUCache:
def __init__(self, capacity: int):
self.cache = {}
self.capacity = capacity
self.head = Node()
self.tail = Node()
self.head.next = self.tail
self.tail.prev = self.head
def get(self, key: int) -> int:
if key in self.cache:
node = self.cache[key]
self._remove(node)
self._add(node)
return node.value
return -1
def put(self, key: int, value: int) -> None:
if key in self.cache:
self._remove(self.cache[key])
node = Node(key, value)
self._add(node)
self.cache[key] = node
if len(self.cache) > self.capacity:
lru = self.head.next
self._remove(lru)
del self.cache[lru.key]
def _remove(self, node):
node.prev.next = node.next
node.next.prev = node.prev
def _add(self, node):
node.prev = self.tail.prev
node.next = self.tail
self.tail.prev.next = node
self.tail.prev = node
# Example usage
cache = LRUCache(2)
cache.put(1, 1)
cache.put(2, 2)
print(cache.get(1)) # returns 1
cache.put(3, 3) # evicts key 2
print(cache.get(2)) # returns -1 (not found)
cache.put(4, 4) # evicts key 1
print(cache.get(1)) # returns -1 (not found)
print(cache.get(3)) # returns 3
print(cache.get(4)) # returns 4
2.3. Implement a Rate Limiter
Question: Design and implement a rate limiter that can be used to control the rate of requests sent to Spotify’s API. The rate limiter should allow a maximum of N requests per minute for each user.
Solution: We can use the token bucket algorithm to implement a rate limiter. Here’s a simple implementation in Python:
import time
class RateLimiter:
def __init__(self, capacity, refill_rate):
self.capacity = capacity
self.refill_rate = refill_rate
self.tokens = capacity
self.last_refill_time = time.time()
def allow_request(self):
self._refill()
if self.tokens > 0:
self.tokens -= 1
return True
return False
def _refill(self):
now = time.time()
time_passed = now - self.last_refill_time
new_tokens = time_passed * self.refill_rate
self.tokens = min(self.capacity, self.tokens + new_tokens)
self.last_refill_time = now
# Example usage
limiter = RateLimiter(capacity=10, refill_rate=1) # 10 requests per minute
for i in range(15):
if limiter.allow_request():
print(f"Request {i+1} allowed")
else:
print(f"Request {i+1} blocked")
time.sleep(0.1) # Simulate time between requests
3. System Design Questions
System design questions are crucial in Spotify interviews, especially for more senior positions. Here are some examples:
3.1. Design Spotify’s Music Streaming System
Question: Design a system that can stream music to millions of users simultaneously, ensuring low latency and high availability.
Key Considerations:
- Content Delivery Network (CDN) for efficient music distribution
- Caching mechanisms to reduce latency
- Load balancing to handle high traffic
- Database design for storing user data, playlists, and music metadata
- Scalability to handle millions of concurrent users
- Fault tolerance and redundancy
3.2. Design Spotify’s Recommendation System
Question: Design a system that can provide personalized music recommendations to users based on their listening history and preferences.
Key Considerations:
- Data collection and storage of user listening history
- Machine learning algorithms for recommendation (e.g., collaborative filtering, content-based filtering)
- Real-time processing of user actions
- Scalability to handle millions of users and songs
- A/B testing framework to improve recommendation quality
3.3. Design Spotify’s Search Functionality
Question: Design a system that allows users to search for songs, artists, albums, and playlists quickly and efficiently.
Key Considerations:
- Indexing strategies for fast search
- Handling typos and providing suggestions
- Ranking search results based on relevance and popularity
- Scalability to handle a large number of concurrent searches
- Caching frequently searched queries
4. Behavioral Interview Questions
Behavioral questions are an essential part of Spotify’s interview process. They help assess your cultural fit and how you handle various situations. Here are some examples:
4.1. Describe a time when you had to work on a challenging project. How did you approach it, and what was the outcome?
When answering this question, use the STAR method (Situation, Task, Action, Result). Highlight your problem-solving skills, teamwork, and ability to overcome obstacles.
4.2. How do you stay updated with the latest technologies and trends in your field?
Discuss your learning habits, such as following tech blogs, attending conferences, or participating in online courses. Emphasize your passion for continuous learning and growth.
4.3. Tell me about a time when you had to work with a difficult team member. How did you handle the situation?
Focus on your communication and conflict resolution skills. Explain how you maintained professionalism and worked towards a common goal despite the challenges.
4.4. Describe a situation where you had to make a decision with incomplete information. How did you proceed?
Highlight your analytical skills and ability to make informed decisions under uncertainty. Discuss how you gathered available information, weighed the pros and cons, and made a decision.
4.5. How do you handle feedback, both positive and constructive?
Emphasize your openness to feedback and your ability to use it for personal and professional growth. Provide examples of how you’ve implemented feedback in the past.
5. Coding Challenges
Spotify often includes coding challenges as part of their interview process. These challenges are designed to test your problem-solving skills and coding ability. Here are a few examples:
5.1. Implement a Function to Detect Cycles in a Playlist
Question: Given a playlist represented as a linked list, write a function to detect if there’s a cycle in the playlist.
Solution: We can use Floyd’s Cycle-Finding Algorithm (also known as the “tortoise and hare” algorithm) to solve this problem efficiently.
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def has_cycle(head):
if not head or not head.next:
return False
slow = head
fast = head.next
while slow != fast:
if not fast or not fast.next:
return False
slow = slow.next
fast = fast.next.next
return True
# Example usage
# Creating a cyclic playlist
node1 = ListNode(1)
node2 = ListNode(2)
node3 = ListNode(3)
node4 = ListNode(4)
node1.next = node2
node2.next = node3
node3.next = node4
node4.next = node2 # Creating a cycle
print(has_cycle(node1)) # Output: True
# Creating a non-cyclic playlist
node5 = ListNode(5)
node6 = ListNode(6)
node7 = ListNode(7)
node5.next = node6
node6.next = node7
print(has_cycle(node5)) # Output: False
5.2. Implement a Function to Merge K Sorted Playlists
Question: Given K sorted playlists, merge them into one sorted playlist.
Solution: We can use a min-heap to efficiently merge the playlists.
import heapq
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def merge_k_playlists(playlists):
dummy = ListNode(0)
current = dummy
heap = []
# Add the first node of each playlist to the heap
for i, playlist in enumerate(playlists):
if playlist:
heapq.heappush(heap, (playlist.val, i, playlist))
while heap:
val, i, node = heapq.heappop(heap)
current.next = ListNode(val)
current = current.next
if node.next:
heapq.heappush(heap, (node.next.val, i, node.next))
return dummy.next
# Helper function to create a linked list from a list
def create_linked_list(arr):
dummy = ListNode(0)
current = dummy
for val in arr:
current.next = ListNode(val)
current = current.next
return dummy.next
# Helper function to convert a linked list to a list
def linked_list_to_list(head):
result = []
while head:
result.append(head.val)
head = head.next
return result
# Example usage
playlist1 = create_linked_list([1, 4, 5])
playlist2 = create_linked_list([1, 3, 4])
playlist3 = create_linked_list([2, 6])
merged = merge_k_playlists([playlist1, playlist2, playlist3])
print(linked_list_to_list(merged)) # Output: [1, 1, 2, 3, 4, 4, 5, 6]
6. Preparation Tips
To increase your chances of success in a Spotify interview, consider the following tips:
- Review Data Structures and Algorithms: Ensure you have a solid understanding of fundamental data structures (arrays, linked lists, trees, graphs, etc.) and algorithms (sorting, searching, dynamic programming, etc.).
- Practice Coding: Solve coding problems on platforms like LeetCode, HackerRank, or AlgoCademy. Focus on implementing efficient solutions and explaining your thought process.
- Study System Design: Familiarize yourself with system design concepts, especially those relevant to music streaming services. Practice designing scalable systems.
- Understand Spotify’s Technology Stack: Research Spotify’s tech stack and familiarize yourself with the technologies they use.
- Prepare for Behavioral Questions: Reflect on your past experiences and prepare stories that demonstrate your skills and problem-solving abilities.
- Stay Updated: Keep up with the latest trends in music streaming technology and Spotify’s recent developments.
- Mock Interviews: Practice with friends or use online platforms that offer mock interviews to get comfortable with the interview process.
- Ask Questions: Prepare thoughtful questions about Spotify’s technology, culture, and the specific role you’re applying for.
7. Conclusion
Preparing for a Spotify interview can be challenging, but with the right approach and practice, you can increase your chances of success. Remember to focus on both technical skills and soft skills, as Spotify values both equally. Use the questions and tips provided in this guide as a starting point for your preparation, but don’t limit yourself to just these. The key is to develop a deep understanding of computer science fundamentals, system design principles, and Spotify’s unique challenges in the music streaming industry.
As you prepare, keep in mind that the interview process is not just about getting the right answers, but also about demonstrating your problem-solving approach, communication skills, and passion for technology and music. Spotify is known for its innovative culture, so don’t be afraid to think creatively and showcase your unique perspectives.
Good luck with your Spotify interview! Remember, each interview is a learning experience, regardless of the outcome. Stay positive, be yourself, and let your skills and enthusiasm shine through.