Top Snap Inc. Interview Questions: Cracking the Code for Success
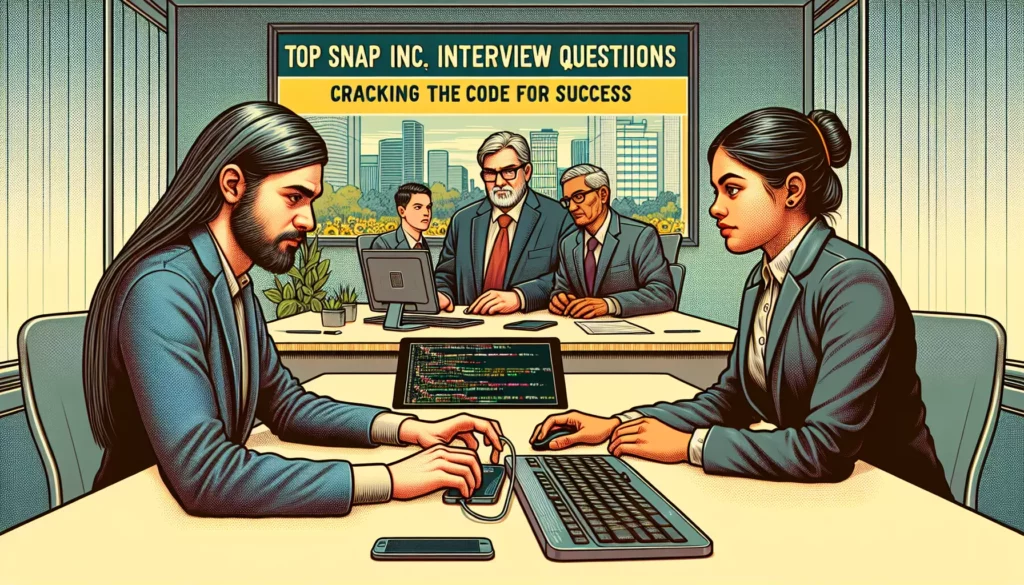
As one of the leading social media and technology companies, Snap Inc. (the parent company of Snapchat) is known for its innovative approach to mobile communication and augmented reality. Landing a job at Snap Inc. can be a dream come true for many software engineers and tech professionals. However, the interview process can be challenging and requires thorough preparation. In this comprehensive guide, we’ll explore some of the most common Snap Inc. interview questions, provide insights into the company’s hiring process, and offer tips to help you succeed in your interview.
Table of Contents
- Understanding Snap Inc. and Its Interview Process
- Technical Interview Questions
- Behavioral Interview Questions
- System Design Questions
- Coding Challenges and Algorithmic Problems
- Preparation Tips for Snap Inc. Interviews
- Conclusion
1. Understanding Snap Inc. and Its Interview Process
Before diving into specific interview questions, it’s essential to understand Snap Inc.’s culture and interview process. Snap Inc. values creativity, innovation, and a strong focus on user experience. The company looks for candidates who are not only technically proficient but also align with its mission to “empower people to express themselves, live in the moment, learn about the world, and have fun together.”
The typical interview process at Snap Inc. may include:
- Initial phone screen or online assessment
- Technical phone interview
- On-site interviews (or virtual on-site interviews)
- Coding challenges
- System design discussions
- Behavioral interviews
Now, let’s explore some of the common questions you might encounter during your Snap Inc. interview.
2. Technical Interview Questions
Technical questions at Snap Inc. are designed to assess your knowledge of fundamental computer science concepts, programming languages, and problem-solving skills. Here are some examples:
2.1 Data Structures and Algorithms
- Implement a function to reverse a linked list.
- How would you design a hash table from scratch?
- Explain the difference between a stack and a queue. Provide an example use case for each.
- Implement a binary search tree and write functions for insertion, deletion, and searching.
- What is the time complexity of quicksort? How does it compare to other sorting algorithms?
2.2 Programming Languages and Concepts
- What are the key differences between object-oriented programming and functional programming?
- Explain the concept of closure in JavaScript.
- How does memory management work in languages like C++ compared to garbage-collected languages like Java?
- What are some features of your favorite programming language that make it unique or particularly useful?
- Describe the difference between processes and threads.
2.3 Database and SQL
- What is the difference between a LEFT JOIN and an INNER JOIN in SQL?
- How would you optimize a slow-running SQL query?
- Explain the concept of database normalization and its benefits.
- What are the ACID properties in database systems?
- How does indexing work in databases, and when should you use it?
Sample Technical Question and Answer
Let’s look at a sample technical question you might encounter in a Snap Inc. interview:
Question: Implement a function to find the longest palindromic substring in a given string.
Here’s a possible solution using dynamic programming:
def longest_palindromic_substring(s):
n = len(s)
# Create a table to store results of subproblems
dp = [[False for _ in range(n)] for _ in range(n)]
# All substrings of length 1 are palindromes
for i in range(n):
dp[i][i] = True
start = 0
max_length = 1
# Check for substrings of length 2
for i in range(n - 1):
if s[i] == s[i + 1]:
dp[i][i + 1] = True
start = i
max_length = 2
# Check for lengths greater than 2
for length in range(3, n + 1):
for i in range(n - length + 1):
j = i + length - 1
if s[i] == s[j] and dp[i + 1][j - 1]:
dp[i][j] = True
if length > max_length:
start = i
max_length = length
return s[start:start + max_length]
# Test the function
print(longest_palindromic_substring("babad")) # Output: "bab" or "aba"
print(longest_palindromic_substring("cbbd")) # Output: "bb"
This solution uses dynamic programming to efficiently find the longest palindromic substring. It has a time complexity of O(n^2) and a space complexity of O(n^2), where n is the length of the input string.
3. Behavioral Interview Questions
Behavioral questions are designed to assess your soft skills, problem-solving abilities, and cultural fit within Snap Inc. Here are some common behavioral questions you might encounter:
- Tell me about a time when you had to work on a challenging project. How did you approach it?
- Describe a situation where you had to work with a difficult team member. How did you handle it?
- Can you share an example of when you had to learn a new technology quickly? How did you go about it?
- Tell me about a time when you had to make a difficult decision. What was your thought process?
- How do you stay updated with the latest trends and technologies in your field?
- Describe a situation where you had to explain a complex technical concept to a non-technical audience.
- Tell me about a time when you failed at something. What did you learn from the experience?
- How do you handle stress and pressure in your work?
- Can you give an example of how you’ve contributed to improving a process or system?
- Describe a time when you had to prioritize multiple tasks. How did you manage your time effectively?
When answering behavioral questions, use the STAR method (Situation, Task, Action, Result) to structure your responses and provide concrete examples from your past experiences.
4. System Design Questions
System design questions are crucial for senior-level positions and assess your ability to design scalable, efficient, and robust systems. Here are some system design questions you might encounter in a Snap Inc. interview:
- Design a real-time chat application like Snapchat’s messaging feature.
- How would you design a system to handle millions of concurrent users for a live video streaming platform?
- Design a distributed cache system.
- How would you architect a system for processing and storing large volumes of image data?
- Design a URL shortening service like bit.ly.
- How would you design a notification system for a social media platform?
- Design a system for detecting and preventing spam in a messaging application.
- How would you architect a system for real-time geolocation tracking of millions of users?
- Design a content delivery network (CDN) for efficient distribution of multimedia content.
- How would you design a recommendation system for a social media platform?
When approaching system design questions, remember to:
- Clarify requirements and constraints
- Start with a high-level design
- Dive into component details
- Discuss trade-offs and potential optimizations
- Consider scalability, reliability, and performance
5. Coding Challenges and Algorithmic Problems
Snap Inc. often includes coding challenges as part of their interview process. These challenges are designed to test your problem-solving skills, coding ability, and algorithmic thinking. Here are some examples of coding challenges you might encounter:
5.1 Array and String Manipulation
- Implement a function to remove duplicates from an unsorted array.
- Find the kth largest element in an unsorted array.
- Implement a function to perform string compression (e.g., “aabcccccaaa” becomes “a2b1c5a3”).
- Determine if two strings are anagrams of each other.
- Implement a function to rotate a matrix by 90 degrees.
5.2 Linked Lists and Trees
- Detect a cycle in a linked list.
- Implement a function to find the lowest common ancestor of two nodes in a binary tree.
- Convert a binary search tree to a sorted doubly linked list.
- Implement a function to check if a binary tree is balanced.
- Serialize and deserialize a binary tree.
5.3 Dynamic Programming and Recursion
- Implement a function to find the longest increasing subsequence in an array.
- Solve the coin change problem (find the minimum number of coins to make a given amount).
- Implement a function to calculate the nth Fibonacci number efficiently.
- Find all possible combinations of a set of parentheses.
- Implement a function to solve the knapsack problem.
5.4 Graphs and Search Algorithms
- Implement Depth-First Search (DFS) and Breadth-First Search (BFS) for a graph.
- Find the shortest path in a weighted graph (Dijkstra’s algorithm).
- Detect a cycle in a directed graph.
- Implement a function to clone a graph.
- Find the number of islands in a 2D grid (connected components problem).
Sample Coding Challenge and Solution
Let’s look at a sample coding challenge you might encounter in a Snap Inc. interview:
Challenge: Implement a LRU (Least Recently Used) Cache with O(1) time complexity for both get and put operations.
Here’s a possible solution using a combination of a hash map and a doubly linked list:
class Node:
def __init__(self, key, value):
self.key = key
self.value = value
self.prev = None
self.next = None
class LRUCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = {}
self.head = Node(0, 0)
self.tail = Node(0, 0)
self.head.next = self.tail
self.tail.prev = self.head
def _add_node(self, node):
node.prev = self.head
node.next = self.head.next
self.head.next.prev = node
self.head.next = node
def _remove_node(self, node):
prev = node.prev
new = node.next
prev.next = new
new.prev = prev
def _move_to_head(self, node):
self._remove_node(node)
self._add_node(node)
def get(self, key):
if key in self.cache:
node = self.cache[key]
self._move_to_head(node)
return node.value
return -1
def put(self, key, value):
if key in self.cache:
node = self.cache[key]
node.value = value
self._move_to_head(node)
else:
new_node = Node(key, value)
self.cache[key] = new_node
self._add_node(new_node)
if len(self.cache) > self.capacity:
lru = self.tail.prev
self._remove_node(lru)
del self.cache[lru.key]
# Test the LRU Cache
cache = LRUCache(2)
cache.put(1, 1)
cache.put(2, 2)
print(cache.get(1)) # returns 1
cache.put(3, 3) # evicts key 2
print(cache.get(2)) # returns -1 (not found)
cache.put(4, 4) # evicts key 1
print(cache.get(1)) # returns -1 (not found)
print(cache.get(3)) # returns 3
print(cache.get(4)) # returns 4
This implementation uses a hash map for O(1) lookup and a doubly linked list to maintain the order of elements based on their recent usage. The most recently used item is always at the head of the list, while the least recently used item is at the tail.
6. Preparation Tips for Snap Inc. Interviews
To increase your chances of success in a Snap Inc. interview, consider the following preparation tips:
- Study core computer science concepts: Review data structures, algorithms, system design, and programming fundamentals.
- Practice coding challenges: Use platforms like LeetCode, HackerRank, or CodeSignal to practice coding problems regularly.
- Brush up on your preferred programming language: Be comfortable with at least one programming language and its standard libraries.
- Understand Snap Inc.’s products and culture: Familiarize yourself with Snapchat and other Snap Inc. products, as well as the company’s values and mission.
- Prepare for behavioral questions: Reflect on your past experiences and prepare stories that demonstrate your skills and problem-solving abilities.
- Work on communication skills: Practice explaining complex technical concepts clearly and concisely.
- Stay updated with industry trends: Keep abreast of the latest developments in mobile technology, augmented reality, and social media.
- Participate in mock interviews: Practice with friends, mentors, or use online platforms that offer mock interview services.
- Review system design concepts: For senior positions, study distributed systems, scalability, and performance optimization techniques.
- Prepare questions for your interviewers: Show your interest in the role and the company by asking thoughtful questions.
7. Conclusion
Preparing for a Snap Inc. interview requires a combination of technical knowledge, problem-solving skills, and cultural fit. By familiarizing yourself with the types of questions asked, practicing coding challenges, and understanding the company’s values, you’ll be well-equipped to tackle the interview process.
Remember that the key to success lies not only in your technical abilities but also in your ability to communicate effectively, think creatively, and demonstrate your passion for technology and innovation. With thorough preparation and a positive attitude, you’ll be well on your way to impressing your interviewers and landing that dream job at Snap Inc.
Good luck with your interview preparation, and may your journey to becoming a part of the Snap Inc. team be successful!