Understanding Database Normalization: A Comprehensive Guide
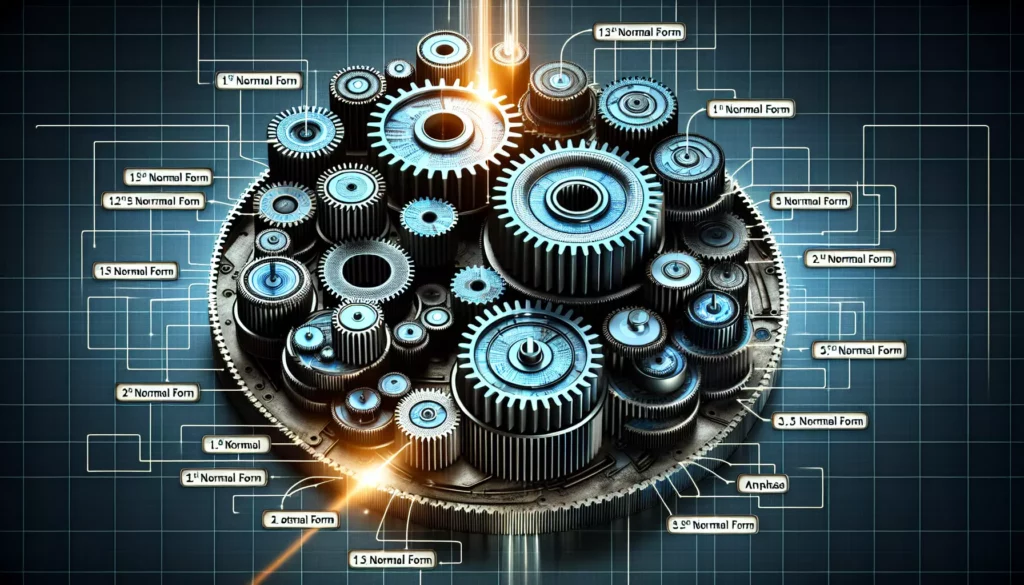
In the world of database design and management, normalization is a fundamental concept that plays a crucial role in ensuring data integrity, reducing redundancy, and optimizing database performance. Whether you’re a beginner in coding education or preparing for technical interviews at major tech companies, understanding normalization is essential for anyone working with databases. In this comprehensive guide, we’ll explore the ins and outs of database normalization, its importance, and how to apply it effectively.
What is Database Normalization?
Database normalization is a systematic approach to organizing data in a relational database. It involves breaking down large tables into smaller, more manageable ones to eliminate redundancy and dependency issues. The primary goals of normalization are:
- Minimizing data redundancy
- Ensuring data integrity
- Reducing data anomalies
- Simplifying data management
- Improving query performance
Normalization is typically achieved through a series of steps, each representing a “normal form.” There are several normal forms, but the most commonly used are the first three: First Normal Form (1NF), Second Normal Form (2NF), and Third Normal Form (3NF).
The Importance of Normalization in Database Design
Proper normalization is crucial for several reasons:
- Data Integrity: By reducing redundancy, normalization helps maintain data consistency across the database.
- Efficiency: Normalized databases often require less storage space and can be more efficiently queried.
- Flexibility: A well-normalized database is easier to modify and extend without causing ripple effects throughout the system.
- Reduced Anomalies: Normalization helps prevent update, insertion, and deletion anomalies that can occur in poorly designed databases.
Normal Forms Explained
Let’s dive into the details of the most common normal forms:
First Normal Form (1NF)
The First Normal Form is the most basic level of normalization. To achieve 1NF, a table must meet the following criteria:
- Each column should contain atomic (indivisible) values
- Each column should have a unique name
- The order of data stored in the table should not matter
- There should be no repeating groups of columns
Example of a table not in 1NF:
| StudentID | Name | Courses |
|-----------|----------|------------------------|
| 1 | John Doe | Math, Physics, Biology |
| 2 | Jane Smith| English, History |
To convert this to 1NF, we would need to split the Courses column:
| StudentID | Name | Course |
|-----------|------------|----------|
| 1 | John Doe | Math |
| 1 | John Doe | Physics |
| 1 | John Doe | Biology |
| 2 | Jane Smith | English |
| 2 | Jane Smith | History |
Second Normal Form (2NF)
To achieve 2NF, a table must first be in 1NF and then meet an additional criterion:
- All non-key attributes must be fully functionally dependent on the primary key
This means that if a table has a composite primary key, all non-key columns should depend on the entire primary key, not just a part of it.
Example of a table not in 2NF:
| OrderID | ProductID | ProductName | Quantity |
|---------|-----------|-------------|----------|
| 1 | 101 | Widget A | 5 |
| 1 | 102 | Widget B | 3 |
| 2 | 101 | Widget A | 2 |
In this example, ProductName depends only on ProductID, not on the entire primary key (OrderID, ProductID). To achieve 2NF, we would split this into two tables:
Orders Table:
| OrderID | ProductID | Quantity |
|---------|-----------|----------|
| 1 | 101 | 5 |
| 1 | 102 | 3 |
| 2 | 101 | 2 |
Products Table:
| ProductID | ProductName |
|-----------|-------------|
| 101 | Widget A |
| 102 | Widget B |
Third Normal Form (3NF)
To achieve 3NF, a table must be in 2NF and satisfy an additional condition:
- All the attributes in a table should be functionally dependent only on the primary key
This means eliminating transitive dependencies, where a non-key column depends on another non-key column.
Example of a table not in 3NF:
| EmployeeID | EmployeeName | DepartmentID | DepartmentName |
|------------|--------------|--------------|----------------|
| 1 | John Doe | 101 | Sales |
| 2 | Jane Smith | 102 | Marketing |
| 3 | Bob Johnson | 101 | Sales |
In this table, DepartmentName is transitively dependent on EmployeeID through DepartmentID. To achieve 3NF, we would split this into two tables:
Employees Table:
| EmployeeID | EmployeeName | DepartmentID |
|------------|--------------|--------------|
| 1 | John Doe | 101 |
| 2 | Jane Smith | 102 |
| 3 | Bob Johnson | 101 |
Departments Table:
| DepartmentID | DepartmentName |
|--------------|----------------|
| 101 | Sales |
| 102 | Marketing |
Advanced Normal Forms
While the first three normal forms are the most commonly used, there are additional, more advanced normal forms:
- Boyce-Codd Normal Form (BCNF): A stricter version of 3NF that deals with certain types of anomalies not addressed by 3NF.
- Fourth Normal Form (4NF): Deals with multi-valued dependencies.
- Fifth Normal Form (5NF): Also known as Project-Join Normal Form, deals with join dependencies.
These advanced forms are less frequently used in practice but can be important in specific scenarios where data integrity is paramount.
Denormalization: When to Break the Rules
While normalization is generally beneficial, there are situations where deliberately denormalizing a database can be advantageous. Denormalization involves adding redundant data to one or more tables to improve read performance.
Reasons for denormalization include:
- Improving query performance for complex joins
- Simplifying certain types of queries
- Reducing the need for table joins in frequently accessed data
However, denormalization should be done cautiously, as it can lead to data inconsistencies if not managed properly.
Practical Steps for Normalizing a Database
When normalizing a database, follow these general steps:
- Identify the entities and attributes in your data
- Create tables for each entity
- Identify the primary keys for each table
- Establish relationships between tables using foreign keys
- Apply the normal forms sequentially (1NF, 2NF, 3NF)
- Review and refine the design
Tools and Techniques for Database Normalization
Several tools and techniques can assist in the normalization process:
- Entity-Relationship Diagrams (ERDs): Visual representations of database structures that help identify entities, attributes, and relationships.
- Functional Dependency Diagrams: Help visualize the dependencies between attributes in a table.
- Database Design Software: Tools like MySQL Workbench, Oracle SQL Developer Data Modeler, or ERwin provide features to assist in database design and normalization.
- Normalization Algorithms: Automated processes that can help identify and resolve normalization issues in existing databases.
Normalization in Practice: A Case Study
Let’s walk through a practical example of normalizing a database for an online bookstore:
Initial unnormalized table:
| OrderID | CustomerName | CustomerEmail | BookTitle | Author | Price | Quantity |
|---------|--------------|---------------|--------------|--------------|-------|----------|
| 1 | John Doe | john@email.com| SQL Basics | Jane Smith | 29.99 | 2 |
| 1 | John Doe | john@email.com| Python Pro | Bob Johnson | 39.99 | 1 |
| 2 | Jane Smith | jane@email.com| SQL Basics | Jane Smith | 29.99 | 1 |
Step 1: Apply 1NF (already satisfied in this case)
Step 2: Apply 2NF
Orders Table:
| OrderID | CustomerID | BookID | Quantity |
|---------|------------|--------|----------|
| 1 | 1 | 1 | 2 |
| 1 | 1 | 2 | 1 |
| 2 | 2 | 1 | 1 |
Customers Table:
| CustomerID | CustomerName | CustomerEmail |
|------------|--------------|----------------|
| 1 | John Doe | john@email.com |
| 2 | Jane Smith | jane@email.com |
Books Table:
| BookID | BookTitle | Author | Price |
|--------|--------------|--------------|-------|
| 1 | SQL Basics | Jane Smith | 29.99 |
| 2 | Python Pro | Bob Johnson | 39.99 |
Step 3: Apply 3NF (already satisfied after 2NF in this case)
This normalized structure eliminates redundancy and separates concerns, making the database more flexible and easier to maintain.
Common Pitfalls in Database Normalization
While normalizing databases, be aware of these common pitfalls:
- Over-normalization: Breaking down tables too much can lead to excessive joins and poor performance.
- Ignoring business requirements: Normalization should balance theoretical correctness with practical needs.
- Neglecting indexing: Proper indexing is crucial for maintaining performance in normalized databases.
- Failing to consider future growth: Design should accommodate potential changes and expansions in data structure.
Normalization and Database Performance
While normalization generally improves data integrity and reduces redundancy, it can impact database performance in various ways:
Positive Impacts:
- Reduced storage requirements
- Faster updates and inserts
- Improved data consistency
Potential Negative Impacts:
- Increased complexity of queries involving multiple joins
- Potential performance degradation for read-heavy operations
To mitigate potential performance issues:
- Use appropriate indexing strategies
- Consider selective denormalization for frequently accessed data
- Optimize queries and database design based on specific use cases
Normalization in Different Database Systems
While the principles of normalization are universal, their implementation can vary across different database management systems:
Relational Databases (e.g., MySQL, PostgreSQL, Oracle)
These systems are built on the relational model and are ideal for implementing normalized designs. They provide robust support for enforcing relationships and constraints.
NoSQL Databases (e.g., MongoDB, Cassandra)
NoSQL databases often use denormalized data models to improve read performance and scalability. However, normalization concepts can still be applied to structure data within documents or column families.
NewSQL Databases (e.g., Google Spanner, CockroachDB)
These systems aim to provide the scalability of NoSQL while maintaining the ACID properties of traditional relational databases. They often support normalized schemas with distributed architectures.
Normalization and Data Warehousing
In data warehousing, the approach to normalization often differs from transactional databases:
- Star Schema: A denormalized structure with a central fact table connected to dimension tables, optimized for analytical queries.
- Snowflake Schema: A more normalized version of the star schema, where dimension tables are normalized into multiple related tables.
The choice between these schemas depends on the specific requirements of the data warehouse, balancing query performance with data integrity and storage efficiency.
Normalization in Modern Application Development
In the context of modern application development, particularly in preparing for technical interviews at major tech companies, understanding normalization remains crucial:
- Microservices Architecture: Proper data modeling and normalization are essential when designing databases for individual microservices.
- API Design: Normalized data structures can inform the design of efficient and flexible APIs.
- ORM (Object-Relational Mapping): Many ORMs work best with normalized database schemas, mapping cleanly to object-oriented code.
Practical Exercises for Learning Normalization
To reinforce your understanding of normalization, try these exercises:
- Take an unnormalized dataset and normalize it to 3NF, explaining each step.
- Analyze a real-world database schema and identify any normalization issues.
- Design a normalized database schema for a simple application (e.g., a library management system).
- Practice writing SQL queries that join normalized tables to retrieve denormalized views of data.
Conclusion
Database normalization is a fundamental concept in data management that plays a crucial role in designing efficient, maintainable, and scalable database systems. By understanding and applying normalization principles, developers can create robust data structures that support the needs of modern applications while minimizing data redundancy and inconsistencies.
As you progress in your coding education and prepare for technical interviews, remember that normalization is not just a theoretical concept but a practical tool that informs database design decisions in real-world scenarios. Whether you’re working with traditional relational databases or exploring newer data storage paradigms, the principles of normalization will continue to be relevant in ensuring data integrity and optimizing database performance.
Keep practicing normalization techniques, and don’t hesitate to apply these concepts in your projects. With time and experience, you’ll develop an intuitive understanding of when and how to apply normalization, as well as when denormalization might be appropriate. This knowledge will be invaluable as you tackle complex data management challenges in your career as a software developer or database administrator.