How To Create A React App: A Comprehensive Guide for Beginners
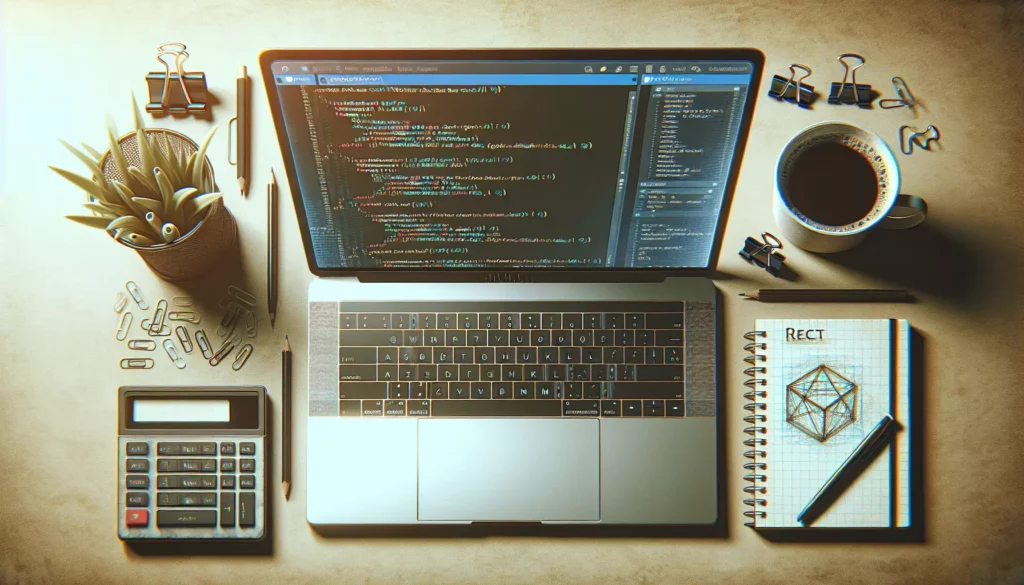
React has become one of the most popular JavaScript libraries for building user interfaces, and for good reason. Its component-based architecture and efficient rendering make it an excellent choice for creating dynamic and responsive web applications. If you’re new to React and wondering how to get started, you’ve come to the right place. In this comprehensive guide, we’ll walk you through the process of creating a React app from scratch, covering everything from setup to deployment.
Table of Contents
- Prerequisites
- Creating a React App
- Understanding the Project Structure
- Creating Components
- Working with State and Props
- Styling Your React App
- Adding Routing to Your App
- Integrating with APIs
- Testing Your React App
- Deploying Your React App
- Best Practices and Tips
- Conclusion
Prerequisites
Before we dive into creating a React app, make sure you have the following prerequisites installed on your system:
- Node.js: React requires Node.js to run. You can download and install it from the official Node.js website.
- npm (Node Package Manager): This comes bundled with Node.js and is used to manage dependencies in your project.
- A code editor: We recommend using Visual Studio Code, but you can use any code editor of your choice.
Creating a React App
The easiest way to create a new React app is by using Create React App, a tool developed by Facebook that sets up a new React project with a solid build configuration. Follow these steps to create your first React app:
- Open your terminal or command prompt.
- Navigate to the directory where you want to create your project.
- Run the following command:
npx create-react-app my-react-app
Replace “my-react-app” with your desired project name.
- Once the installation is complete, navigate into your project folder:
cd my-react-app
- Start the development server:
npm start
This will launch your new React app in your default browser, typically at http://localhost:3000
.
Understanding the Project Structure
After creating your React app, you’ll see a folder structure that looks something like this:
my-react-app/
README.md
node_modules/
package.json
public/
index.html
favicon.ico
src/
App.css
App.js
App.test.js
index.css
index.js
logo.svg
Let’s break down the key files and folders:
- package.json: Contains project dependencies and scripts.
- public/index.html: The main HTML file where your React app will be rendered.
- src/index.js: The JavaScript entry point of your application.
- src/App.js: The main React component of your application.
- src/App.css: CSS styles for the App component.
Creating Components
React is all about components. Let’s create a simple component to understand how they work. In your src
folder, create a new file called Greeting.js
:
import React from 'react';
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
export default Greeting;
Now, let’s use this component in our App.js
:
import React from 'react';
import './App.css';
import Greeting from './Greeting';
function App() {
return (
<div className="App">
<Greeting name="World" />
</div>
);
}
export default App;
This will display “Hello, World!” in your app.
Working with State and Props
React components can have two types of data: props and state. Props are passed down from parent components, while state is managed within a component. Let’s modify our Greeting
component to use state:
import React, { useState } from 'react';
function Greeting(props) {
const [name, setName] = useState(props.name);
const handleChange = (event) => {
setName(event.target.value);
};
return (
<div>
<h1>Hello, {name}!</h1>
<input type="text" value={name} onChange={handleChange} />
</div>
);
}
export default Greeting;
Now the greeting will update as you type in the input field.
Styling Your React App
There are several ways to style your React components. Let’s look at three common approaches:
1. CSS Files
You can create a separate CSS file for each component. For example, create a Greeting.css
file:
.greeting {
font-size: 24px;
color: #333;
}
.greeting input {
margin-top: 10px;
padding: 5px;
}
Then import it in your Greeting.js
file:
import React, { useState } from 'react';
import './Greeting.css';
function Greeting(props) {
// ... (previous code)
return (
<div className="greeting">
<h1>Hello, {name}!</h1>
<input type="text" value={name} onChange={handleChange} />
</div>
);
}
export default Greeting;
2. Inline Styles
You can use inline styles by passing a JavaScript object to the style
prop:
function Greeting(props) {
// ... (previous code)
const styles = {
fontSize: '24px',
color: '#333'
};
return (
<div style={styles}>
<h1>Hello, {name}!</h1>
<input type="text" value={name} onChange={handleChange} />
</div>
);
}
3. CSS-in-JS Libraries
Libraries like styled-components allow you to write CSS directly in your JavaScript files. First, install styled-components:
npm install styled-components
Then use it in your component:
import React, { useState } from 'react';
import styled from 'styled-components';
const GreetingWrapper = styled.div`
font-size: 24px;
color: #333;
input {
margin-top: 10px;
padding: 5px;
}
`;
function Greeting(props) {
// ... (previous code)
return (
<GreetingWrapper>
<h1>Hello, {name}!</h1>
<input type="text" value={name} onChange={handleChange} />
</GreetingWrapper>
);
}
Adding Routing to Your App
As your app grows, you’ll likely want to add multiple pages or views. React Router is the most popular library for handling routing in React applications. Let’s add it to our project:
- Install React Router:
npm install react-router-dom
- Create a new component for a second page, e.g.,
About.js
:
import React from 'react';
function About() {
return <h1>About Us</h1>;
}
export default About;
- Modify your
App.js
to include routing:
import React from 'react';
import { BrowserRouter as Router, Route, Link, Switch } from 'react-router-dom';
import Greeting from './Greeting';
import About from './About';
function App() {
return (
<Router>
<div>
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
</ul>
</nav>
<Switch>
<Route path="/about">
<About />
</Route>
<Route path="/">
<Greeting name="World" />
</Route>
</Switch>
</div>
</Router>
);
}
export default App;
Now your app has two pages that you can navigate between.
Integrating with APIs
Most real-world applications need to interact with APIs to fetch or send data. Let’s create a component that fetches data from an API:
import React, { useState, useEffect } from 'react';
function UserList() {
const [users, setUsers] = useState([]);
useEffect(() => {
fetch('https://jsonplaceholder.typicode.com/users')
.then(response => response.json())
.then(data => setUsers(data));
}, []);
return (
<div>
<h1>Users</h1>
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
</div>
);
}
export default UserList;
This component uses the useEffect
hook to fetch data when the component mounts and the useState
hook to store and update the fetched data.
Testing Your React App
Testing is crucial for maintaining a robust application. React apps created with Create React App come with Jest as the test runner. Let’s write a simple test for our Greeting
component:
Create a file named Greeting.test.js
in the same directory as Greeting.js
:
import React from 'react';
import { render, screen } from '@testing-library/react';
import Greeting from './Greeting';
test('renders greeting with name', () => {
render(<Greeting name="Alice" />);
const greetingElement = screen.getByText(/Hello, Alice!/i);
expect(greetingElement).toBeInTheDocument();
});
Run the test with:
npm test
Deploying Your React App
Once your app is ready, you’ll want to deploy it. Here’s how to deploy to GitHub Pages:
- Install the
gh-pages
package:
npm install gh-pages --save-dev
- Add the following to your
package.json
:
"homepage": "https://yourusername.github.io/your-repo-name",
"scripts": {
// other scripts
"predeploy": "npm run build",
"deploy": "gh-pages -d build"
}
- Deploy your app:
npm run deploy
This will create a gh-pages
branch in your repository with your built app.
Best Practices and Tips
- Keep components small and focused: Each component should do one thing well.
- Use functional components and hooks: They’re simpler and more efficient than class components.
- Manage state carefully: Use local component state for UI-specific state, and consider using Redux or Context API for global state management in larger applications.
- Use PropTypes for type checking: This helps catch bugs early by ensuring that components receive the correct types of props.
- Write tests: Testing helps ensure your components work as expected and makes refactoring easier.
- Use meaningful names: Choose clear, descriptive names for your components, functions, and variables.
- Keep your dependencies up to date: Regularly update your project’s dependencies to get the latest features and security updates.
Conclusion
Creating a React app is an exciting journey that opens up a world of possibilities for building dynamic and interactive web applications. We’ve covered the basics of setting up a React project, creating components, managing state and props, styling, routing, API integration, testing, and deployment. As you continue to work with React, you’ll discover more advanced concepts and techniques that will help you build even more sophisticated applications.
Remember, the key to mastering React (or any programming skill) is practice. Don’t be afraid to experiment, build projects, and learn from your mistakes. The React community is vast and supportive, so don’t hesitate to seek help when you need it.
Happy coding, and may your React journey be filled with exciting discoveries and successful projects!