Mastering the Command Line Interface: A Comprehensive Guide for Programmers
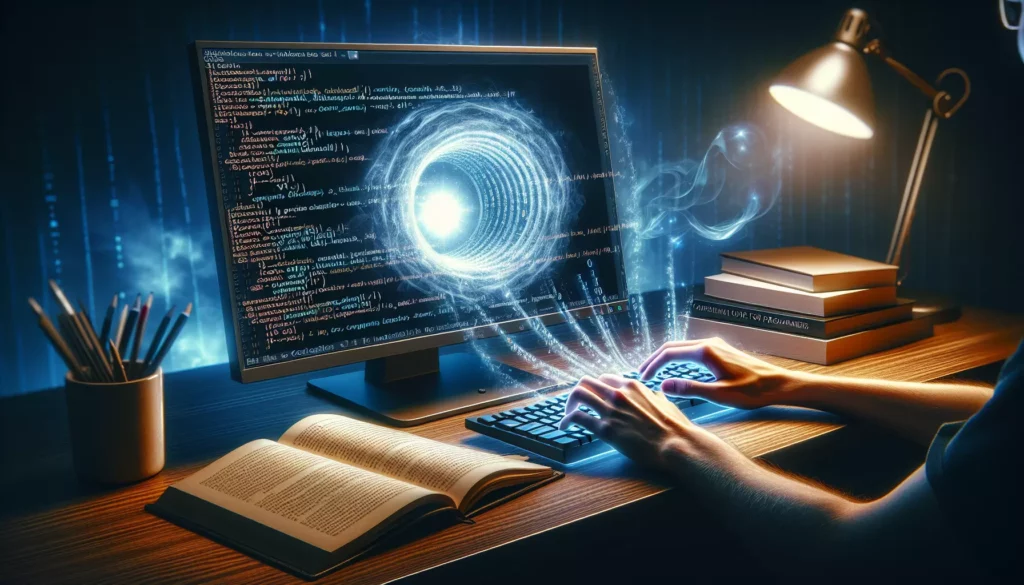
In the world of programming and software development, the Command Line Interface (CLI) remains an indispensable tool. Despite the prevalence of graphical user interfaces (GUIs), mastering the CLI is crucial for any programmer looking to enhance their efficiency, automate tasks, and gain deeper control over their computing environment. This comprehensive guide will walk you through the essentials of the Command Line Interface, its importance in coding education, and how it can significantly boost your programming skills.
Table of Contents
- What is the Command Line Interface?
- The Importance of CLI in Programming
- Basic CLI Commands Every Programmer Should Know
- Advanced CLI Techniques
- CLI in Different Operating Systems
- Using CLI for Web Development
- CLI in Data Science and Machine Learning
- Essential CLI Tools for Programmers
- Best Practices for Using CLI
- CLI Skills in Technical Interviews
- Conclusion
1. What is the Command Line Interface?
The Command Line Interface, often abbreviated as CLI, is a text-based interface used to interact with a computer’s operating system or software by typing commands. Unlike graphical user interfaces (GUIs) that rely on visual elements like icons and menus, CLIs operate purely through text input and output.
Key characteristics of CLIs include:
- Text-based input and output
- Ability to execute complex commands and scripts
- Typically faster and more efficient than GUIs for certain tasks
- Offers more granular control over system operations
- Essential for remote server management and automation
2. The Importance of CLI in Programming
For programmers, the CLI is more than just an alternative to GUIs; it’s a powerful tool that can significantly enhance productivity and capabilities. Here’s why CLI proficiency is crucial in coding education and professional development:
- Efficiency: CLI commands can perform complex operations quickly, often faster than navigating through GUI menus.
- Automation: Scripts can be written to automate repetitive tasks, saving time and reducing errors.
- Remote Access: CLIs are essential for managing remote servers and cloud resources, which often don’t have GUIs.
- Debugging: Many debugging tools and logs are accessed through the command line.
- Version Control: Git, the most popular version control system, is primarily used through CLI.
- Package Management: Installing, updating, and managing software packages and dependencies often requires CLI usage.
3. Basic CLI Commands Every Programmer Should Know
Regardless of your programming specialty, there are several fundamental CLI commands that you should be familiar with:
File and Directory Operations:
ls
(Unix/Linux) ordir
(Windows): List directory contentscd
: Change directorypwd
: Print working directorymkdir
: Make a new directoryrm
(Unix/Linux) ordel
(Windows): Remove files or directoriescp
(Unix/Linux) orcopy
(Windows): Copy files or directoriesmv
(Unix/Linux) ormove
(Windows): Move or rename files or directories
File Viewing and Editing:
cat
(Unix/Linux) ortype
(Windows): Display file contentsnano
orvim
: Text editors in the terminalgrep
: Search for patterns in files
System Information and Management:
top
(Unix/Linux) ortaskmgr
(Windows): View running processesps
: List running processeskill
(Unix/Linux) ortaskkill
(Windows): Terminate processes
Example: Creating and Navigating Directories
mkdir my_project
cd my_project
mkdir src tests docs
ls
cd src
touch main.py
cd ..
tree
This sequence of commands creates a project structure, navigates through it, creates a file, and then displays the directory tree.
4. Advanced CLI Techniques
As you become more comfortable with basic CLI operations, you can explore more advanced techniques to further enhance your productivity:
Piping and Redirection
Piping (|
) allows you to use the output of one command as the input for another. Redirection (>
, <
, >>
) lets you control where the input comes from or where the output goes.
ls -l | grep ".txt" > text_files.txt
This command lists all files, filters for those ending in .txt, and saves the result to a file.
Environment Variables
Environment variables store system-wide values that programs can access. You can set, view, and use these variables in your CLI operations.
export MY_VAR="Hello, World!"
echo $MY_VAR
Aliases
Aliases allow you to create shorthand versions of commonly used commands.
alias ll="ls -la"
ll
Shell Scripting
Shell scripts allow you to combine multiple CLI commands into a single executable file, enabling complex automation tasks.
#!/bin/bash
echo "Starting backup process..."
rsync -avz /path/to/source/ /path/to/destination/
echo "Backup completed!"
5. CLI in Different Operating Systems
While the concept of CLI remains the same across different operating systems, the specific implementations and commands can vary:
Unix-like Systems (Linux, macOS)
These systems use shells like Bash, Zsh, or Fish. They share many common commands and conventions.
- Terminal emulators: Terminal (macOS), GNOME Terminal (Linux)
- Common shells: Bash, Zsh
- Package managers: apt (Debian/Ubuntu), brew (macOS)
Windows
Windows has its own command-line interface, traditionally Command Prompt, and more recently PowerShell.
- Command Prompt: Uses DOS-style commands
- PowerShell: More powerful, with object-oriented pipelines
- Windows Subsystem for Linux (WSL): Allows running a Linux environment directly on Windows
Cross-Platform Considerations
When working across different operating systems, it’s important to be aware of the differences in command syntax and available tools. For cross-platform development, consider using tools like Git Bash on Windows, which provides a Unix-like environment.
6. Using CLI for Web Development
The command line interface plays a crucial role in modern web development workflows. Here are some key areas where CLI is indispensable:
Version Control with Git
Git, the most widely used version control system, is primarily operated through the CLI:
git init
git add .
git commit -m "Initial commit"
git push origin main
Package Management
Package managers like npm (Node Package Manager) for JavaScript or pip for Python are typically used via CLI:
npm install express
pip install django
Build Tools and Task Runners
Many build tools and task runners are configured and run through the command line:
webpack --mode production
gulp build
Database Management
Interacting with databases often involves CLI tools:
mysql -u username -p database_name
psql -U username -d database_name
Deployment and Server Management
Deploying applications and managing servers frequently requires CLI usage:
ssh user@hostname
docker build -t my-app .
docker run -d -p 8080:80 my-app
7. CLI in Data Science and Machine Learning
Data scientists and machine learning engineers also heavily rely on CLI tools for various tasks:
Data Processing
Command-line tools like awk, sed, and cut are powerful for quick data manipulation:
cat data.csv | awk -F"," '{print $2}' | sort | uniq -c
Jupyter Notebooks
Jupyter notebooks, a popular tool for data analysis, are often launched from the command line:
jupyter notebook
Machine Learning Workflows
Many machine learning libraries and tools are used via CLI:
python train_model.py --epochs 100 --batch-size 32
tensorboard --logdir=path/to/logs
Cloud Computing
Interacting with cloud services often requires CLI tools:
aws s3 cp large_dataset.csv s3://my-bucket/
gcloud compute instances create my-instance --machine-type=n1-standard-4
8. Essential CLI Tools for Programmers
Beyond basic system commands, there are numerous CLI tools that can significantly enhance a programmer’s workflow:
Text Editors
- Vim/Neovim: Powerful, customizable text editors
- Emacs: An extensible, customizable text editor
Version Control
- Git: Distributed version control system
- SVN: Centralized version control system
Package Managers
- npm/yarn: For JavaScript
- pip: For Python
- gem: For Ruby
Build Tools
- Make: General-purpose build tool
- Maven/Gradle: For Java projects
Networking Tools
- curl: Transfer data using various protocols
- wget: Retrieve files using HTTP, HTTPS, and FTP
- netstat: Network statistics
System Monitoring
- htop: Interactive process viewer
- nmon: Performance monitoring tool
Example: Using curl for API Testing
curl -X POST https://api.example.com/data \
-H "Content-Type: application/json" \
-d '{"key": "value"}'
This command sends a POST request to an API endpoint with a JSON payload.
9. Best Practices for Using CLI
To make the most of the command line interface, consider these best practices:
1. Use Tab Completion
Most modern shells support tab completion, which can save time and reduce typos.
2. Learn Keyboard Shortcuts
Familiarize yourself with shortcuts like Ctrl+A (move to beginning of line) and Ctrl+E (move to end of line).
3. Create Aliases for Common Commands
Set up aliases for commands you use frequently to save time.
4. Use Command History
Utilize the up and down arrow keys to navigate through your command history.
5. Understand File Permissions
Learn how to view and modify file permissions using commands like chmod
and chown
.
6. Practice Safe Command Execution
Always double-check destructive commands (like rm -rf
) before executing them.
7. Learn to Read Man Pages
Man pages (accessed via the man
command) provide detailed information about commands and their options.
8. Use Version Control
Integrate version control into your CLI workflow for better project management.
9. Customize Your Shell
Personalize your shell environment with custom prompts, color schemes, and configurations.
10. Regularly Update Your Skills
Stay updated with new CLI tools and features relevant to your field.
10. CLI Skills in Technical Interviews
Command line proficiency can be a valuable asset in technical interviews, especially for positions at major tech companies. Here’s how CLI skills might come into play:
System Design Questions
You might be asked to explain how you would use CLI tools to manage and monitor large-scale systems.
Coding Challenges
Some interviews might involve writing shell scripts or using command-line tools to solve problems.
DevOps and SRE Roles
For these positions, deep CLI knowledge is often crucial and may be extensively tested.
General Programming Knowledge
Interviewers might assess your familiarity with common development tools and workflows, many of which are CLI-based.
Example Interview Question
“How would you find all files larger than 100MB in a directory and its subdirectories, then move them to a separate folder?”
A possible solution using CLI:
find /path/to/directory -type f -size +100M -exec mv {} /path/to/large_files \;
11. Conclusion
Mastering the Command Line Interface is an essential skill for any programmer, regardless of their specific area of expertise. From basic file operations to complex system management and development workflows, the CLI offers unparalleled efficiency and control. As you progress in your coding education and prepare for technical interviews, particularly for positions at major tech companies, your proficiency with the command line can set you apart as a skilled and efficient developer.
Remember that becoming proficient with the CLI is an ongoing process. Start with the basics, gradually incorporate more advanced techniques into your workflow, and always be on the lookout for new tools and commands that can enhance your productivity. With practice and persistence, you’ll find that the command line becomes an indispensable part of your programming toolkit, enabling you to work more efficiently and tackle more complex challenges with ease.
As you continue your journey in programming and prepare for technical interviews, consider the CLI as not just a tool, but a fundamental skill that can open doors to more advanced topics in software development, system administration, and beyond. Embrace the power of the command line, and watch as it transforms your approach to problem-solving and coding efficiency.