Essential Command Line Commands for Programmers: Boost Your Coding Efficiency
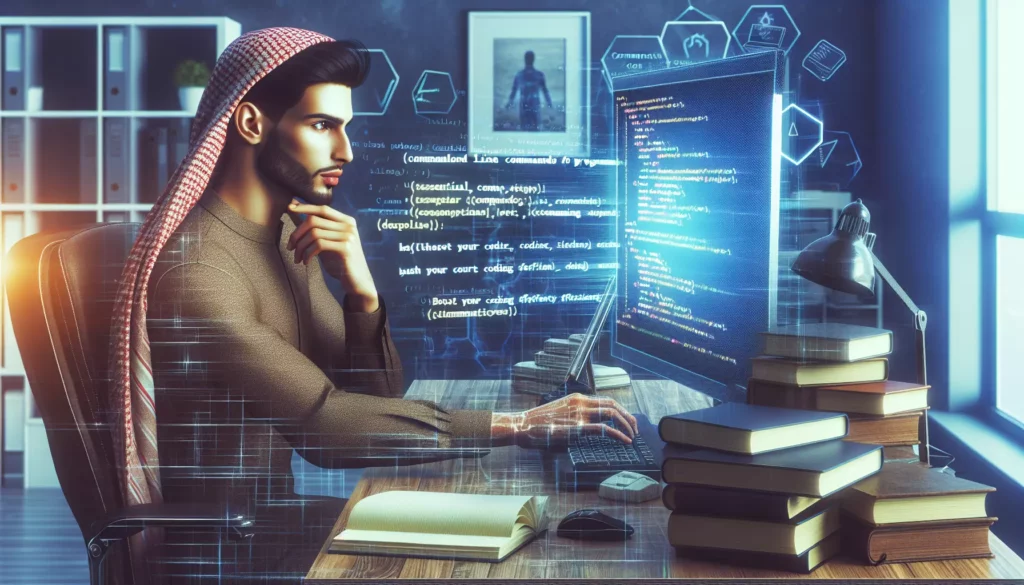
As a programmer, mastering the command line interface (CLI) is crucial for enhancing your productivity and efficiency. Whether you’re a beginner just starting your coding journey or an experienced developer looking to refine your skills, understanding and utilizing command line commands can significantly streamline your workflow. In this comprehensive guide, we’ll explore essential command line commands that every programmer should know, along with practical examples and tips to help you leverage the power of the CLI in your coding projects.
Table of Contents
- Introduction to the Command Line
- Navigation and File Management
- File Manipulation and Text Processing
- System Information and Monitoring
- Networking Commands
- Package Management
- Version Control with Git
- Shell Scripting Basics
- Advanced Commands and Utilities
- Best Practices and Tips
- Conclusion
1. Introduction to the Command Line
The command line interface, also known as the terminal or shell, is a text-based interface for interacting with your computer’s operating system. It allows you to execute commands, manipulate files, and perform various system operations more efficiently than using a graphical user interface (GUI).
Before we dive into specific commands, it’s essential to understand that different operating systems may have slightly different command syntaxes. In this guide, we’ll focus primarily on Unix-like systems (such as Linux and macOS), but many commands are similar or have equivalents in Windows PowerShell.
2. Navigation and File Management
pwd (Print Working Directory)
The pwd
command displays the current directory you’re working in.
$ pwd
/home/user/projects
ls (List)
Use ls
to list the contents of a directory.
$ ls
file1.txt file2.txt folder1 folder2
# List with details
$ ls -l
total 16
-rw-r--r-- 1 user user 1024 Jan 1 12:00 file1.txt
-rw-r--r-- 1 user user 2048 Jan 2 14:30 file2.txt
drwxr-xr-x 2 user user 4096 Jan 3 09:15 folder1
drwxr-xr-x 2 user user 4096 Jan 4 16:45 folder2
# List hidden files
$ ls -a
. .. .hidden_file file1.txt file2.txt folder1 folder2
cd (Change Directory)
Navigate between directories using the cd
command.
$ cd folder1
$ pwd
/home/user/projects/folder1
# Go up one level
$ cd ..
$ pwd
/home/user/projects
# Go to home directory
$ cd ~
$ pwd
/home/user
# Go to root directory
$ cd /
$ pwd
/
mkdir (Make Directory)
Create new directories with the mkdir
command.
$ mkdir new_folder
$ ls
file1.txt file2.txt folder1 folder2 new_folder
# Create nested directories
$ mkdir -p parent/child/grandchild
rm (Remove)
Delete files and directories using the rm
command.
# Remove a file
$ rm file1.txt
# Remove a directory and its contents
$ rm -r folder1
# Force removal without prompting
$ rm -rf folder2
Caution: Be extremely careful when using rm
, especially with the -rf
flags, as it can permanently delete files and directories without confirmation.
cp (Copy)
Copy files and directories with the cp
command.
# Copy a file
$ cp file1.txt file1_backup.txt
# Copy a directory and its contents
$ cp -r folder1 folder1_backup
mv (Move)
Move or rename files and directories using the mv
command.
# Move a file
$ mv file1.txt folder1/
# Rename a file
$ mv file2.txt new_name.txt
# Move and rename a directory
$ mv folder1 new_location/new_folder_name
3. File Manipulation and Text Processing
cat (Concatenate)
Display the contents of a file or concatenate multiple files.
# Display file contents
$ cat file.txt
# Concatenate multiple files
$ cat file1.txt file2.txt > combined.txt
less
View large files one screen at a time.
$ less large_file.txt
Use arrow keys to navigate, press ‘q’ to quit.
head and tail
Display the beginning or end of a file.
# Show first 10 lines
$ head file.txt
# Show last 10 lines
$ tail file.txt
# Show last 20 lines
$ tail -n 20 file.txt
grep (Global Regular Expression Print)
Search for patterns in files or command output.
# Search for a word in a file
$ grep "error" log.txt
# Case-insensitive search
$ grep -i "warning" log.txt
# Recursive search in directories
$ grep -r "TODO" ./src
# Show line numbers
$ grep -n "function" script.js
sed (Stream Editor)
Perform text transformations on files or input streams.
# Replace all occurrences of 'old' with 'new'
$ sed 's/old/new/g' file.txt
# Delete lines containing a pattern
$ sed '/pattern/d' file.txt
# Insert text at the beginning of each line
$ sed 's/^/prefix: /' file.txt
awk
Process and analyze text files.
# Print specific columns
$ awk '{print $1, $3}' data.txt
# Sum values in a column
$ awk '{sum += $2} END {print sum}' numbers.txt
# Filter rows based on a condition
$ awk '$3 > 100 {print $0}' data.txt
4. System Information and Monitoring
top
Display and update sorted information about system processes.
$ top
ps (Process Status)
Show information about active processes.
# Show all processes
$ ps aux
# Show process tree
$ ps axjf
df (Disk Free)
Display disk space usage.
$ df -h
du (Disk Usage)
Estimate file and directory space usage.
# Show directory sizes
$ du -sh *
# Show total size of a directory
$ du -sh /path/to/directory
free
Display amount of free and used memory in the system.
$ free -h
5. Networking Commands
ping
Test network connectivity to a host.
$ ping google.com
curl
Transfer data from or to a server.
# GET request
$ curl https://api.example.com
# POST request
$ curl -X POST -d "data=value" https://api.example.com
# Download a file
$ curl -O https://example.com/file.zip
wget
Download files from the web.
$ wget https://example.com/file.zip
netstat
Display network connections and statistics.
# Show all active connections
$ netstat -a
# Show listening ports
$ netstat -l
6. Package Management
apt (Advanced Package Tool) – for Debian-based systems
# Update package list
$ sudo apt update
# Upgrade installed packages
$ sudo apt upgrade
# Install a package
$ sudo apt install package_name
# Remove a package
$ sudo apt remove package_name
yum (Yellowdog Updater Modified) – for Red Hat-based systems
# Update package list
$ sudo yum check-update
# Upgrade installed packages
$ sudo yum upgrade
# Install a package
$ sudo yum install package_name
# Remove a package
$ sudo yum remove package_name
7. Version Control with Git
git init
Initialize a new Git repository.
$ git init
git clone
Clone a repository into a new directory.
$ git clone https://github.com/user/repo.git
git add
Add files to the staging area.
# Add a specific file
$ git add file.txt
# Add all changes
$ git add .
git commit
Record changes to the repository.
$ git commit -m "Commit message"
git push
Update remote refs along with associated objects.
$ git push origin main
git pull
Fetch from and integrate with another repository or local branch.
$ git pull origin main
git branch
List, create, or delete branches.
# List branches
$ git branch
# Create a new branch
$ git branch new-feature
# Delete a branch
$ git branch -d old-feature
git checkout
Switch branches or restore working tree files.
# Switch to a branch
$ git checkout branch-name
# Create and switch to a new branch
$ git checkout -b new-feature
8. Shell Scripting Basics
Shell scripting allows you to automate tasks and create powerful command-line tools. Here’s a basic example of a shell script:
#!/bin/bash
# This is a comment
echo "Hello, World!"
# Variables
name="John"
echo "Hello, $name!"
# Conditional statements
if [ "$name" == "John" ]; then
echo "Name is John"
else
echo "Name is not John"
fi
# Loops
for i in {1..5}; do
echo "Number: $i"
done
# Functions
greet() {
echo "Hello, $1!"
}
greet "Alice"
Save this script as example.sh
and make it executable with chmod +x example.sh
. Run it using ./example.sh
.
9. Advanced Commands and Utilities
find
Search for files in a directory hierarchy.
# Find files by name
$ find /path/to/search -name "*.txt"
# Find files modified in the last 7 days
$ find /path/to/search -mtime -7
# Find and delete files
$ find /path/to/search -name "*.tmp" -delete
xargs
Build and execute command lines from standard input.
# Find and remove all .log files
$ find . -name "*.log" | xargs rm
# Parallel execution
$ find . -name "*.txt" | xargs -P 4 -I {} gzip {}
screen
Multiplex terminal sessions.
# Start a new screen session
$ screen
# Detach from a screen session
Ctrl+A, then D
# List screen sessions
$ screen -ls
# Reattach to a screen session
$ screen -r [session_id]
tmux
Terminal multiplexer, similar to screen but with more features.
# Start a new tmux session
$ tmux
# Create a new window
Ctrl+B, then C
# Switch between windows
Ctrl+B, then window number
# Split pane vertically
Ctrl+B, then %
# Split pane horizontally
Ctrl+B, then "
10. Best Practices and Tips
- Use tab completion: Most shells support tab completion, which can save time and reduce typing errors.
- Utilize command history: Use the up and down arrow keys to navigate through previously executed commands.
- Create aliases: Set up aliases for frequently used commands or command combinations.
- Use pipes and redirection: Combine commands using pipes (|) and redirect output using > or >>.
- Learn regular expressions: Many command-line tools support regular expressions for powerful text processing.
- Use man pages: Consult the manual pages for detailed information about commands using
man command_name
. - Practice keyboard shortcuts: Learn and use keyboard shortcuts to navigate and edit the command line efficiently.
- Backup important data: Always backup critical data before performing operations that could potentially modify or delete files.
- Use version control: Implement version control for your projects to track changes and collaborate effectively.
- Keep your system updated: Regularly update your system and installed packages to ensure security and stability.
Conclusion
Mastering command line commands is an essential skill for any programmer. The commands and concepts covered in this guide provide a solid foundation for working efficiently in a command-line environment. As you continue to develop your coding skills, you’ll find that the command line becomes an indispensable tool in your programming toolkit.
Remember that the best way to become proficient with these commands is through regular practice and application in real-world scenarios. Don’t be afraid to experiment and explore additional commands and options as you encounter new challenges in your programming projects.
By leveraging the power of the command line, you’ll be able to streamline your workflow, automate repetitive tasks, and gain a deeper understanding of your development environment. This knowledge will prove invaluable as you progress in your coding journey and tackle more complex programming challenges.