Mastering the Code Review Interview: A Comprehensive Guide
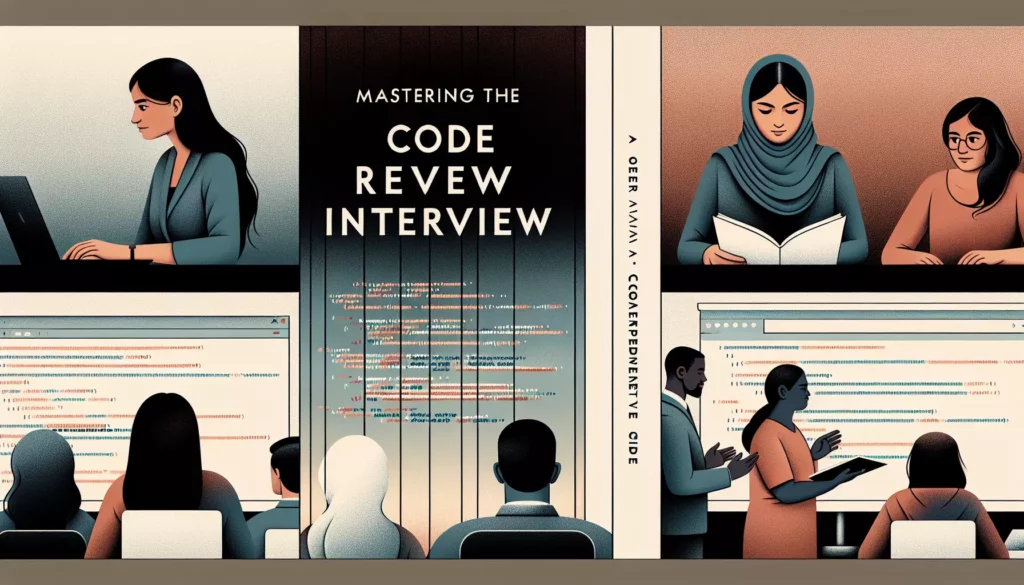
In the ever-evolving landscape of software development, the ability to review and critique code effectively has become an indispensable skill. As companies seek to maintain high-quality codebases and foster collaborative environments, the code review interview has emerged as a crucial component of the hiring process for senior software engineers and tech leads. This comprehensive guide will delve into the intricacies of code review interviews, providing you with the knowledge and strategies needed to excel in this challenging yet rewarding aspect of technical interviews.
Understanding the Code Review Interview
A code review interview is a specialized type of technical assessment that focuses on evaluating a candidate’s ability to analyze, critique, and improve existing code. Unlike traditional coding interviews that may require you to write code from scratch, a code review interview presents you with a pre-written piece of code and asks you to provide feedback, identify issues, and suggest improvements.
This type of interview is particularly relevant for senior positions, as it simulates real-world scenarios where experienced developers are often tasked with reviewing their peers’ work, mentoring junior developers, and maintaining code quality across projects.
Key Skills Tested in Code Review Interviews
Code review interviews are designed to assess a wide range of skills that are essential for senior developers and tech leads. Some of the key areas that interviewers focus on include:
- Code Quality Assessment: The ability to quickly identify well-written code and areas that need improvement.
- Debugging Skills: Spotting bugs, logical errors, and potential runtime issues in the given code.
- Knowledge of Best Practices: Familiarity with industry-standard coding conventions, design patterns, and architectural principles.
- Performance Optimization: Identifying inefficiencies and suggesting ways to improve the code’s performance.
- Security Awareness: Recognizing potential security vulnerabilities and proposing fixes.
- Readability and Maintainability: Assessing how easy the code is to understand and maintain, and suggesting improvements in these areas.
- Communication Skills: Articulating your thoughts clearly and providing constructive feedback in a professional manner.
The Code Review Interview Process
While the specific format may vary between companies, a typical code review interview often follows this general structure:
- Introduction: The interviewer explains the task and provides context for the code you’ll be reviewing.
- Code Presentation: You’re given a piece of code, either on a screen, whiteboard, or as a printout.
- Review Time: You’re allowed time to silently review the code and make notes.
- Discussion: You present your findings, highlighting issues and suggesting improvements.
- Q&A: The interviewer may ask follow-up questions or present hypothetical scenarios based on your review.
- Feedback: Sometimes, you might receive immediate feedback on your performance.
Strategies for Excelling in Code Review Interviews
1. Develop a Systematic Approach
Having a structured method for reviewing code can help you cover all important aspects without missing crucial details. Consider following this step-by-step approach:
- Understand the purpose and context of the code.
- Scan for obvious errors or red flags.
- Evaluate the overall structure and architecture.
- Analyze the code for readability and maintainability.
- Check for potential performance issues.
- Look for security vulnerabilities.
- Consider edge cases and error handling.
- Assess the quality of comments and documentation.
2. Practice Active Reading
When reviewing code, don’t just skim through it passively. Engage with the code actively by:
- Tracing the flow of execution in your mind.
- Questioning the purpose of each function and variable.
- Considering alternative implementations.
- Thinking about how you would test the code.
3. Prioritize Your Feedback
In a time-constrained interview, it’s crucial to focus on the most important issues first. Prioritize your feedback based on:
- Severity of the issue (e.g., bugs that could crash the program)
- Impact on code maintainability and readability
- Performance implications
- Security concerns
- Adherence to best practices and coding standards
4. Be Constructive and Specific
When providing feedback, aim to be constructive rather than purely critical. For each issue you identify:
- Clearly explain the problem
- Provide a specific example from the code
- Suggest a concrete improvement or alternative approach
- Explain the benefits of your suggested change
5. Use Industry-Standard Terminology
Demonstrate your expertise by using appropriate technical terms and concepts. For example:
- Design patterns (e.g., Singleton, Factory, Observer)
- SOLID principles
- Big O notation for discussing complexity
- Language-specific concepts and best practices
6. Be Prepared to Defend Your Position
The interviewer may challenge your feedback or ask for further explanation. Be ready to:
- Provide reasoning for your suggestions
- Discuss trade-offs between different approaches
- Admit when you’re unsure and discuss how you’d find more information
Common Pitfalls to Avoid
To increase your chances of success in a code review interview, be mindful of these common mistakes:
- Nitpicking: Don’t focus too much on minor stylistic issues at the expense of more significant problems.
- Being overly negative: Balance criticism with positive observations about what the code does well.
- Ignoring context: Consider the purpose and constraints of the code before suggesting changes.
- Proposing rewrites: Suggest incremental improvements rather than advocating for complete rewrites, unless absolutely necessary.
- Assuming too much: If something is unclear, ask for clarification rather than making assumptions.
- Forgetting about testing: Consider how changes would affect existing tests or what new tests might be needed.
Example: Reviewing a Code Snippet
Let’s practice by reviewing a simple Python function that calculates the factorial of a number:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
print(factorial(5))
Here’s how you might approach reviewing this code:
- Functionality: The function correctly implements a recursive factorial calculation.
- Error Handling: There’s no handling for negative inputs, which could lead to a stack overflow.
- Performance: For large inputs, this recursive approach could be inefficient and potentially cause a stack overflow.
- Readability: The function is concise and easy to understand, but could benefit from a docstring explaining its purpose and parameters.
- Testing: There’s a print statement that seems to be for testing purposes, which should be removed or turned into a proper unit test.
Based on these observations, you might suggest the following improvements:
def factorial(n):
"""
Calculate the factorial of a non-negative integer.
Args:
n (int): The number to calculate the factorial of.
Returns:
int: The factorial of n.
Raises:
ValueError: If n is negative.
"""
if not isinstance(n, int):
raise TypeError("Input must be an integer")
if n < 0:
raise ValueError("Factorial is not defined for negative numbers")
result = 1
for i in range(1, n + 1):
result *= i
return result
# Example usage
if __name__ == "__main__":
print(factorial(5)) # Expected output: 120
In this improved version:
- We’ve added input validation to handle negative numbers and non-integer inputs.
- The recursive approach has been replaced with an iterative one to improve performance and avoid potential stack overflow issues.
- A comprehensive docstring has been added to explain the function’s purpose, parameters, return value, and possible exceptions.
- The test print statement has been moved into a conditional block, making it easier to import this function into other modules without side effects.
Preparing for Code Review Interviews
To excel in code review interviews, consider incorporating these preparation strategies into your routine:
- Practice regularly: Review open-source projects on platforms like GitHub to hone your skills.
- Stay updated: Keep abreast of the latest best practices, design patterns, and language-specific improvements.
- Participate in code reviews: If possible, engage in code reviews at your current job or contribute to open-source projects.
- Study common anti-patterns: Familiarize yourself with typical coding mistakes and how to address them.
- Improve your communication skills: Practice explaining technical concepts clearly and concisely.
- Learn from others: Read code review comments on open-source projects to see how experienced developers provide feedback.
- Mock interviews: Ask a colleague or mentor to give you sample code to review and provide feedback on your performance.
The Role of Code Reviews in Modern Software Development
Understanding the importance of code reviews in the software development lifecycle can help you appreciate why companies value this skill so highly:
- Quality Assurance: Code reviews help catch bugs and issues early in the development process.
- Knowledge Sharing: They facilitate the exchange of ideas and best practices among team members.
- Mentorship: Senior developers can guide junior team members through code reviews.
- Consistency: Regular reviews help maintain coding standards and consistency across the codebase.
- Collective Ownership: Reviews foster a sense of shared responsibility for the codebase.
- Continuous Improvement: They provide opportunities for ongoing learning and refinement of coding skills.
Conclusion
Code review interviews are a powerful tool for assessing a candidate’s ability to contribute to high-quality software development. By honing your skills in analyzing code, providing constructive feedback, and communicating effectively, you can not only excel in these interviews but also become a valuable asset to any development team.
Remember that the goal of a code review is not just to find faults, but to collaborate on improving the overall quality of the codebase. Approach these interviews with a mindset of continuous learning and improvement, and you’ll be well-positioned to succeed in senior software engineering and tech lead roles.
As you prepare for your next code review interview, keep practicing, stay curious, and always be ready to explain your reasoning. With dedication and the right approach, you can master the art of code reviews and take your software development career to new heights.